Question
Q1) To class SinglyList, add method removeBeforeLast() which removes the node before the last node, consider all possible cases. Q2) To class DoublyLinkedList, add method
Q1) To class SinglyList, add method removeBeforeLast() which removes the node before the last node, consider all possible cases.
Q2) To class DoublyLinkedList, add method reverse which reverse the order of the elements in the list.
Q3) Our implementation of a doubly list relies on two sentinel nodes, header and trailer. Re-implement the DoublyLinkedList without using these nodes. class SinglyList:
public class SinglyLinkedList
public static class Node
private E element;
private Node
public Node(E e, Node
element = e;
next = n;
}
public E getElement() {
return element;
}
public Node
return next;
}
public void setNext(Node
this.next = next;
}
}
private Node
private Node
private int size = 0;
public SinglyLinkedList(){
}
public int size() {
return size;
}
public boolean isEmpty(){
return size ==0;
}
public E first(){
if(isEmpty())
return null;
return head.getElement();
}
public E last(){
if (isEmpty())
return null;
return tail.getElement();
}
}
class DoublyLinkedList:
public class DoublyLinkedList
public static class Node
private E element;
private Node
private Node
public Node(E e, Node
element = e;
prev = p;
next = n;
}
public E getElement() {
return element;
}
public Node
return prev;
}
public Node
return next;
}
public void setPrev(Node
next = p;
}
public void setNext(Node
next = n;
}
}
private Node
private Node
private int size = 0;
public DoublyLinkedList(){
header = new Node<>(null,null,null);
trailer = new Node<>(null, header, null);
header.setNext(trailer);
}
public int size(){
return size;
}
public boolean isEmpty(){
return size ==0;
}
public E first(){
if(isEmpty())
return null;
return header.getNext().getElement();
}
public E last(){
if(isEmpty())
return null;
return trailer.getPrev().getElement();
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
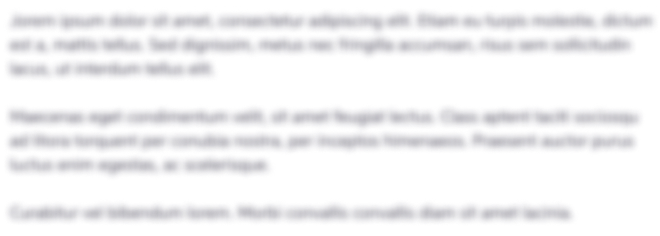
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started