Question
Q1. Write a program to simulate a grocery waiting queue. Your program should ask the user if they want to add a customer to the
Q1. Write a program to simulate a grocery waiting queue. Your program should ask the user if they want to add a customer to the queue, serve the next customer in the queue, or exit. When a customer is served or added to the queue, the program should print out the name of that customer and the remaining customers in the queue. The store has two queues: one is for normal customers, another is for VIP customers. Normal customers can only wait in the normal customers queue, and VIP customers can only wait in the VIP customers queue. Each time a new customer comes; the user should input the customer name and whether he/she is VIP, then put the user in the corresponding queue. You should always serve VIP customers first! When add/ serve customers, you also need to print out the current customer name, and names of all customers in each queue. If you want to add one customer but the corresponding queue is full, your program should print: Error: Normal customers queue is full or Error: VIP customers queue is full. When you want to serve one customer, if both queues are empty, your program should print: Error: Both queues are empty. You may restrict the capacity of each queue to 3 for testing simplicity. Each queue (normal or VIP) should be an instance of the CircularQueue class. Therefore, this class should be included in your program. Following is the output from a sample run of the program
Q2 The goal of this exercise is to create a doubly linked list. You have to use the file d_linked_node.py and the d_linked_list.py .The d_linked_node is ready. You need to complete the d_linked_list.py. Please note that item is the value in the d_linked_node and not the next and previous. Methods given in the d_linked_list.py file search(self, item) Returns true if the item is inside the list false otherwise index(self,item) Returns the index of the item or -1 if the item is not in the list Methods to be completed in the d_linked_list.py file add(self, item) Adds a new item to the front of the list remove(self,item) Removes the first element that is equal to item from the list. if that doesn't exist it changes nothing append(self, item) Adds item to the end of the list insert(self, pos, item) Add item to the given postition in the list (i.e if pos is 0 then item goes to the beginning of the list) pop1(self) Removes and returns the last item in the list pop(self, pos) Removes and returns the item in the given postion search_larger(self, item) Returns the position of the first item that is larger than item, -?1 if there is no item larger get_size(self) Returns the size of the list get_item(self, pos) Returns the item that exists at pos, Raises exception if pos doesnt exist pos can be negative which means the position from the end of the list. (-? 1 is the last item, -?2 the second from last etc.) __str__(self) Returns a string that is the elements of the DLinkedList with spaces in between Once you have completed the method d_linked_list.py file, you can run the test function in provided in the d_linked_list.py file to determine if the methods have been implemented correctly or not.
d_linked_node.py
class d_linked_node: def __init__(self, initData, initNext, initPrevious): # constructs a new node and initializes it to contain # the given object (initData) and links to the given next # and previous nodes. self.__data = initData self.__next = initNext self.__previous = initPrevious if (initPrevious != None): initPrevious.__next = self if (initNext != None): initNext.__previous = self def getData(self): return self.__data def getNext(self): return self.__next def getPrevious(self): return self.__previous def setData(self, newData): self.__data = newData def setNext(self, newNext): self.__next= newNext def setPrevious(self, newPrevious): self.__previous= newPrevious
d_linked_list.py
from d_linked_node import d_linked_node
class d_linked_list: def __init__(self): self.__head=None self.__tail=None self.__size=0
def search(self, item): current = self.__head found = False while current != None and not found: if current.getData() == item: found= True else: current = current.getNext() return found def index(self, item): current = self.__head found = False index = 0 while current != None and not found: if current.getData() == item: found= True else: current = current.getNext() index = index + 1 if not found: index = -1 return index def add(self, item): # TODO: def remove(self, item): # TODO: def append(self, item): # TODO: def insert(self, pos, item): # TODO: def pop1(self): # TODO: def pop(self, pos): # TODO: def search_larger(self, item): # TODO: def get_size(self): # TODO: def get_item(self, pos): # TODO: def __str__(self): # TODO:
def test(): linked_list = d_linked_list() is_pass = (linked_list.get_size() == 0) assert is_pass == True, "fail the test" linked_list.add("World") linked_list.add("Hello") is_pass = (str(linked_list) == "Hello World") assert is_pass == True, "fail the test" is_pass = (linked_list.get_size() == 2) assert is_pass == True, "fail the test" is_pass = (linked_list.get_item(0) == "Hello") assert is_pass == True, "fail the test" is_pass = (linked_list.get_item(1) == "World") assert is_pass == True, "fail the test" is_pass = (linked_list.get_item(0) == "Hello" and linked_list.get_size() == 2) assert is_pass == True, "fail the test" is_pass = (linked_list.pop(1) == "World") assert is_pass == True, "fail the test" is_pass = (linked_list.pop1() == "Hello") assert is_pass == True, "fail the test" is_pass = (linked_list.get_size() == 0) assert is_pass == True, "fail the test" int_list2 = d_linked_list() for i in range(0, 10): int_list2.add(i) int_list2.remove(1) int_list2.remove(3) int_list2.remove(2) int_list2.remove(0) is_pass = (str(int_list2) == "9 8 7 6 5 4") assert is_pass == True, "fail the test" for i in range(11, 13): int_list2.append(i) is_pass = (str(int_list2) == "9 8 7 6 5 4 11 12") assert is_pass == True, "fail the test" for i in range(21, 23): int_list2.insert(0,i) is_pass = (str(int_list2) == "22 21 9 8 7 6 5 4 11 12") assert is_pass == True, "fail the test" is_pass = (int_list2.get_size() == 10) assert is_pass == True, "fail the test" int_list = d_linked_list() is_pass = (int_list.get_size() == 0) assert is_pass == True, "fail the test" for i in range(0, 1000): int_list.append(i) correctOrder = True is_pass = (int_list.get_size() == 1000) assert is_pass == True, "fail the test" for i in range(0, 200): if int_list.pop1() != 999 - i: correctOrder = False is_pass = correctOrder assert is_pass == True, "fail the test" is_pass = (int_list.search_larger(200) == 201) assert is_pass == True, "fail the test" int_list.insert(7,801) is_pass = (int_list.search_larger(800) == 7) assert is_pass == True, "fail the test" is_pass = (int_list.get_item(-1) == 799) assert is_pass == True, "fail the test" is_pass = (int_list.get_item(-4) == 796) assert is_pass == True, "fail the test" if is_pass == True: print ("=========== Congratulations! Your have finished exercise 1! ============") if __name__ == '__main__': test()
Add, Serve, or Exit: add Enter the name of the person to add: Alice Is the customer VIP? False add Alice to the line people in the line JAlice] VIP customers queue: ]] Add, Serve, or Exit: add Enter the name of the person to add: Bob Is the customer VIP? False add Bob to the line. people in the line JAlice, Bob] VIP customers queue: ] Add, Serve, or Exit: add Enter the name of the person to add: John Is the customer VIP? False add John to the line. people in the Line: JAI1ce, Bob, JohnJ VIP customers queue: ] Add, Serve, or Exit: add Enter the name of the person to add: Mike
Step by Step Solution
There are 3 Steps involved in it
Step: 1
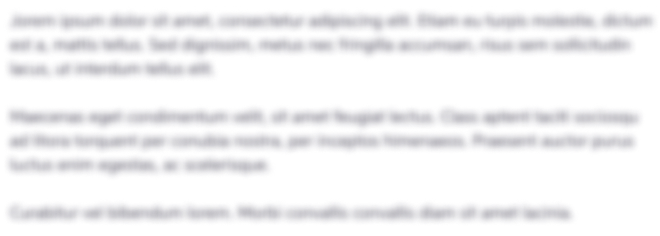
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started