Answered step by step
Verified Expert Solution
Question
1 Approved Answer
# queens.py # # ICS 3 3 Winter 2 0 2 4 # Project 0 : History of Modern # # A module containing tools
# queens.py
#
# ICS Winter
# Project : History of Modern
#
# A module containing tools that could assist in solving variants of the
# wellknown nqueens" problem. Note that we're only implementing one part
# of the problem: immutably managing the "state" of the board ie which
# queens are arranged in which cells The rest of the problem determining
# a valid solution for it is not our focus here.
#
# Your goal is to complete the QueensState class described below, though
# you'll need to build it incrementally, as well as test it incrementally by
# writing unit tests in testqueens.py Make sure you've read the project
# writeup before you proceed, as it will explain the requirements around
# following and documenting an incremental process of solving this problem.
#
# DO NOT MODIFY THE Position NAMEDTUPLE OR THE PROVIDED EXCEPTION CLASSES.
from collections import namedtuple
from typing import Self
Position namedtuplePositionrow 'column'
# Ordinarily, we would write docstrings within classes or their methods.
# Since a namedtuple builds those classes and methods for us we instead
# add the documentation by hand afterward.
Position.doc 'A position on a chessboard, specified by zerobased row and column numbers.
Position.row.doc 'A zerobased row number'
Position.column.doc 'A zerobased column number'
class DuplicateQueenErrorException:
An exception indicating an attempt to add a queen where one is already present."""
def initself position: Position:
Initializes the exception, given a position where the duplicate queen exists."""
self.position position
def strself str:
return f'duplicate queen in row selfposition.row column selfposition.column
class MissingQueenErrorException:
An exception indicating an attempt to remove a queen where one is not present."""
def initself position: Position:
Initializes the exception, given a position where a queen is missing."""
self.position position
def strself str:
return f'missing queen in row selfposition.row column selfposition.column
class QueensState:
Immutably represents the state of a chessboard being used to assist in
solving the nqueens problem."""
def initself rows: int, columns: int:
Initializes the chessboard to have the given numbers of rows and columns,
with no queens occupying any of its cells."""
pass
def queencountself int:
Returns the number of queens on the chessboard."""
pass
def queensself listPosition:
Returns a list of the positions in which queens appear on the chessboard,
arranged in no particular order."""
pass
def hasqueenself position: Position bool:
Returns True if a queen occupies the given position on the chessboard, or
False otherwise."""
pass
def anyqueensunsafeself bool:
Returns True if any queens on the chessboard are unsafe ie they can
be captured by at least one other queen on the chessboard or False otherwise."""
pass
def withqueensaddedself positions: listPosition Self:
Builds a new QueensState with queens added in the given positions.
Raises a DuplicateQueenException when there is already a queen in at
least one of the given positions."""
pass
def withqueensremovedself positions: listPosition Self:
Builds a new QueensState with queens removed from the given positions.
Raises a MissingQueenException when there is no queen in at least one of
the given positions."""
pass
Step by Step Solution
There are 3 Steps involved in it
Step: 1
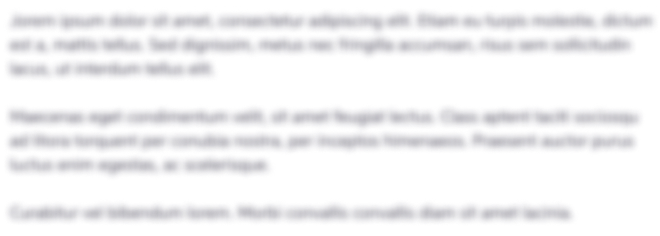
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started