Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Question 1: [10 points] Write a java program that performs the following: Create class named Customer that contains the following: Two private non-static data members:
Question 1: [10 points]
Write a java program that performs the following:
Create class named Customer that contains the following:
- Two private non-static data members:
- name: represents the name of the customer and its type is String.
- category: represent the category of the customer and its type is integer. There are three categories: 1 for first class customers, 2 for second class customers, and 3 for third class customers.
- Member methods as follows:
- Default constructor: initializes the name to no name and the category to 3.
- Parametrized constructor: receives two values for the name and category. Then, it initializes the received values in the name and category data members respectively.
- setName method: that receives a string value and sets the received value in the name data member.
- setCategory method: that receives an integer and sets the received value in the category data member.
- getName method: that returns the name of the customer.
- getCategory method: that returns the category of the customer.
- getDiscount method: that returns the discount given to customer. 30% discount is given to customers of category 1, 20% discount is given to customers of category 2, and 10% discount is given to customers of category 3.
- getStars method: that returns the number of stars given to customer. The number of stars depends on customer category. 3 stars (***) are given to customers of category 1, 2 stars (**) are given to customers of category 2, and 1 star (*) is given to customers of category 3.
- toString overridden method: that returns the following data: customer name, customer category, number of stars, and discount value.
Create class named Invoice that contains the following:
- Three private non-static data members:
- invoiceID: represents the id of the invoice and its type is integer. The value of invoiceID is assigned the current value of the autoGeneratedInvoiceID.
- customer: represents the customer of the invoice and its type is Customer.
- amount: represents the amount of the invoice before discount and its type is double.
- One private static data member:
- autoGeneratedInvoiceID: represents the auto-generated id of the invoice of type integer which is initialized to 0. For each time invoice object is created, autoGeneratedInvoiceID value is incremented automatically by 1 and the result is assigned to invoiceID.
- Member methods as follows:
- Default constructor: initializes the customer with the default constructor of the Customer class and the amount to 0.0.
- Parametrized constructor: receives 2 values for customer object and amount. Then, it initializes the customer object using the received object and the received amount value in the amount data member.
- setCustomer method: that receives an object of type Customer and sets its value in the customer object.
- setAmount method: that receives a double value and sets the received value in the amount data member.
- getCustomer method: that returns the customer object of the invoice.
- getAmount method: that returns the amount of the invoice.
- getAmountAfterDiscount method: that returns the amount of the invoice after discount.
- toString overridden method: that returns the data members of the class in the following way:
invoice id, customer name, customer category, number of stars, discount value, amount of the invoice, amount of the invoice after discount. [Please, see sample output at end of page].
Create class named Demo that contains the main method. In the main method do the following:
- Create an array of 4 objects of type Customer. Ask the user to enter the values of the customer name and category. Fill the array with this data by using the parametrized constructor.
- Create an array of 4 objects of type Invoice. Ask the user to enter the value of the invoice amount. Fill the array with this data by using the parametrized constructor.
Input data:
- Change the name of the third customer to "Rami".
- Change the category of the first customer to 2.
- Change the amount value of the last invoice to 400.
- Print data of all invoices. I.e., invoice id, customer name, customer category, number of stars, discount value, amount of the invoice, amount of the invoice after discount. [Hint: you need to call toString method of Invoice class]. A sample output may look like this:
Sample Output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
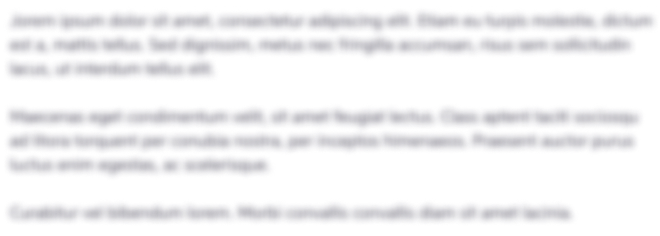
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started