Question
QUESTION: 1) Implement Singly Linked List detectLoop which check whether the linked list contains a loop. - Its an instance method that takes no parameter.
QUESTION:
1) Implement Singly Linked List detectLoop which check whether the linked list contains a loop. - Its an instance method that takes no parameter. It returns true if the linked list contains a
loop that goes on forever if tracing the list from head. It returns false if it does not contain a
loop. 2) Implement Doubly Linked List add method which add an element to a specific position. - Its an instance method that takes a position and an element, then adds the element to this
specific position and shifts the element currently at this position and any subsequent elements to the right. It throws an exception if the position is out of bound. It traverses the list from header if the position is closer to the header and traverses the list from trailer otherwise.
3) Implement Polynomial Linked List add method which adds two polynomials in standard form and returns sum polynomial which also has to be in standard form. - Rewrite the instance method that takes a second Polynomial Linked List, and then adds all
terms in the caller Polynomial and all terms in the second Polynomial Linked List together into another sum Polynomial. Both caller and parameter Polynomials are expected to be in standard form, the method should return the sum Polynomial also in standard form. A standard form polynomial has no duplicate terms (each terms exponent is unique) and the exponent is in descending order.
4) Implement Polynomial Linked list multiply method, similar to add, it returns the result polynomial in standard form. using this code below:
public class A1LinkedList{ public static void main(String argc[]){ Linkedlistsl = new Linkedlist<>(); DLinkedList dl = new DLinkedList<>(); PolynomialLinkedlist sum, prod; for (int i = 1000; i > 0; i-=3) sl.add(i); try { sl.insert(111, sl.getNode(50), sl.getNode(51)); if (sl.detectLoop()) System.out.println("Loop!"); else System.out.println("No loop."); sl.insert(123, sl.getNode(51), sl.getNode(50)); if (sl.detectLoop()) System.out.println("Loop!"); else System.out.println("No loop."); } catch(Exception e){ e.printStackTrace(); } dl.add("Three",0); dl.add("Five",1); dl.add("One",0); dl.add("Two",1); dl.add("Four",3); dl.print(); PolynomialLinkedlist p1 = new PolynomialLinkedlist(2,3); PolynomialLinkedlist p2 = new PolynomialLinkedlist(3,2); p3 = p1.add(p2); p1 = new PolynomialLinkedlist(3,2); p2 = new PolynomialLinkedlist(1,0); p4 = p1.add(p2); sum = p3.add(p4); prod = p3.multiply(p4); sum.print(); prod.print(); p1.print(); p2.print(); } } class Linkedlist { private static class Node { private E element; private Node next; public Node(E e, Node n){ element = e; next = n; } public E getE(){ return element; } public Node getNext(){ return next; } public void setE(E e){ element = e; } public void setNext(Node n){ next = n; } } private Node head; public Linkedlist(){ head = null; } public void add(E e){ Node temp = new Node<>(e, head); head = temp; } public void insert(E e, Node p, Node n){ p.setNext(new Node<>(e, n)); } public Node getNode(int i) throws Exception{ Node temp = head; while (i > 0){ if (temp == null) throw new Exception("Out of bound"); temp = temp.getNext(); i--; } return temp; } } class DLinkedList { private static class DNode { private E element; private DNode prev; private DNode next; public DNode(E e){ this(e, null, null); } public DNode(E e, DNode p, DNode n){ element = e; prev = p; next = n; } public E getE(){ return element; } public DNode getPrev(){ return prev; } public DNode getNext(){ return next; } public void setE(E e){ element = e; } public void setPrev(DNode p){ prev = p; } public void setNext(DNode n){ next = n; } } private DNode header; private DNode trailer; private int size; public DLinkedList(){ header = new DNode (null); trailer = new DNode (null, header, null); header.setNext(trailer); size = 0; } public void print(){ DNode temp = header.getNext(); while (temp != trailer){ System.out.print(temp.getE().toString() + ", "); temp = temp.getNext(); } System.out.println(); } } class PolynomialLinkedlist{ private static class PNode{ private int coe; private int exp; private PNode next; public PNode(int c, int e){ this(c, e, null); } public PNode(int c, int e, PNode n){ coe = c; exp = e; next = n; } public void setCoe(int c){ coe = c;} public void setExp(int e){ exp = e;} public void setNext(PNode n){ next = n;} public int getCoe(){ return coe;} public int getExp(){ return exp;} public PNode getNext(){ return next;} } private PNode first; private PNode last; public PolynomialLinkedlist(){ first = last = null; } public PolynomialLinkedlist(int c, int e){ PNode tempn = new PNode(c, e); first = last = tempn; } public void print(){ if (first == null){ System.out.println(); return; } PNode temp = first; String ans = ""; while (temp != null){ if (temp.getCoe() > 0) { if (temp != first) ans = ans + " + "; ans = ans + temp.getCoe(); } else if (temp.getCoe() < 0) ans = ans + " - " + temp.getCoe() * -1; if (temp.getExp() != 0){ ans = ans + "X^" + temp.getExp(); } temp = temp.getNext(); } System.out.println(ans); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
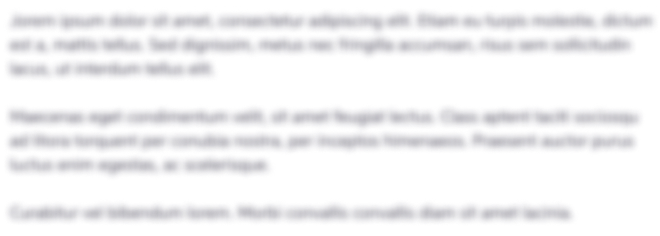
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started