Question
Question 1 What is the output of the code corresponding to the following pseudocode? (In the answer options, new lines are separated by commas.) Declare
Question 1
What is the output of the code corresponding to the following pseudocode? (In the answer options, new lines are separated by commas.)
Declare X As Integer
Declare Y As Integer
For (X = 1; X <=2; X++)
For (Y = 3; Y <= 4; Y++)
Write X * Y
End For(Y)
End For(X)
3, 4, 6, 8 |
None of these are correct output |
4, 5, 5, 6 |
1, 3, 2, 4 |
Question 2
Which of the following loops cannot be nested in a For loop?
Repeat ... Until |
Do ... While |
For |
All of these can be nested in a For loop |
While |
Question 3
What is the output of the code corresponding to the pseudocode shown?
Declare G As Integer
Declare H As Integer
Set G = 7
While G <= 8
Set H = 6
While H <= 7
Write G + H
Set H = H + 1
End While(H)
Set G = G + 1
End While(G)
7, 6, 7, 8 |
13, 14, 14, 15 |
7, 6, 7, 7, 8, 6, 8, 7 |
13, 14, 15 |
Question 4
What will be displayed after code corresponding to the following pseudocode is run?
Declare A As Integer
Declare B As Integer
Declare C As Integer
Set A = 3
While A <= 6
Set B = (2 * A) 1
Write B
Set C = A
While C <= (A+1)
Write C
Set C = C + 1
End While(C)
Set A = A + 2
End While(A)
5, 3, 3, 9, 5, 5 |
5, 3, 4, 9, 5, 6 |
5, 3, 9, 5 |
3, 5, 4, 5, 9, 5 |
Question 5
What is the output of code corresponding to the following pseudocode? (In the answer options, new lines are separated by commas.)
Declare A As Integer
Declare B As Float
Set A = 2
While A <= 3
Set B = 2.5 * A
Write B
Set B = Int(B)
Write B
Set A = A + 1
End While
5, 5, 7.5, 7 |
2, 3, 5, 7 |
5, 7 |
5, 5, 7, 7 |
Question 6
If MyNumber = 7.82, what is the value of Int(MyNumber/2)+ 0.5?
3.91 |
3.5 |
4.41 |
4.5 |
Question 7
What is wrong with the following pseudocode?
Declare Count As Integer
Declare TheNumber As Integer
Set TheNumber = 12
For (Count = 10; Count>TheNumber; Count--)
Write TheNumber + Count
End For
The loop will never be entered since the initial value of Count is less than the test condition |
The limit condition in a For loop cannot be a variable |
A counter must start at 0 or 1 |
The loop will never end since the test condition will never be met |
Question 8
Which statement would produce an output of one of the following numbers:
5, 6, 7, 8, 9, 10
Floor(Random() * 5) + 5 |
Floor(Random()) + 5 |
Floor(Random() * 9) - 5 |
Floor(Random() * 6) + 5 |
Question 9
What is the output of the code corresponding to the following pseudocode? (In the answer options, new lines are separated by commas.)
Declare Y As Integer
Declare X As Integer
For (Y = 1; Y <=2; Y++)
For (X = 1; X <= 2; X++)
Write Y * X
End For(X)
End For(Y)
None of these are correct ouput |
1, 2, 2, 4 |
4, 5, 5, 6 |
3, 4, 6, 8 |
Question 10
What does the following program segment do?
Declare Count As Integer
Declare Sum As Integer
Set Sum = 0
For (Count = 1; Count < 50; Count++)
Set Sum = Sum + Count
End For
It does nothing since there is no Write statement |
It sums all the integers from 1 through 49 |
It sums all the integers from 0 through 50 |
It sums all the integers from 1 through 50 |
Question 11
What is the first and last output corresponding to the following pseudocode? (Note, this question has 2 parts; you must answer each part correctly to receive full marks. Enter only the numbers in the space provided.)
The first iteration displays the value .
The last iteration displays the value .
For (N = 4; N <= 9; N + 4)
Set X = 10/N
Write X
Set X = Int(X)
Write X
End For
Question 12
Complete the test condition for the following pseudocode that simulates flipping a coin 25 times by generating displaying 25 random integers, each of which is either 1 or 2. (Note, this question has 2 parts; you must answer each part correctly to receive full marks. Enter only the numbers in the space provided.)
Declare Coin As Integer
Declare X As Integer
For (X = ; X <= ; X++)
Set Coin = Floor(Random() * 2) + 1
Select Case Of Coin
Case 1:
Write Heads
Break
Case 2:
Write Tails
Break
Default: Break
End Case
End For
Question 13
Complete the test condition for the following pseudocode that validates the input data.
Declare Number As Float
Repeat
Write Enter a negative number:
Input Number
Until Number __________
Question 14
If a counter named MyCount in a For loop has the initial value of 5 on the first pass and we want it to go through 4 iterations, increasing its value by 5 on each pass, the test condition would be written as __________.
Question 15
Complete the random generator code for the following pseudocode that simulates rolling a die 10 times by generating and displaying 10 random integers, with a range of 1 to 6. (Note, this question has 2 parts; you must answer each part correctly to receive full marks. Enter only the numbers in the space provided.)
Declare Die, X As Integer
For (X = 1; X <= 10; X++)
Set Die = Floor(Random()* )+
Write Die face: + Die
End For
Question 16
When one loop is contained within another loop, we say these are __________ loops.
Flag this Question
Question 171 pts
What is the first output corresponding to the following pseudocode? (Note, this question has 2 parts; you must answer each part correctly to receive full marks. Enter only the numbers in the space provided.)
The first iteration displays the value and
Declare M As Integer
Declare P As Integer
Repeat
Set P = 1
While P < 4
Write M + and + P
Set P = P + 1
End While(P)
Set M = M + 1
Until M = 3
Question 18
What is the first and second output corresponding to the following pseudocode?
(Note, this question has 2 parts; you must answer each part correctly to receive full marks. Enter only the numbers in the space provided.)
The first iteration displays the value .
The second iteration displays the value .
Declare I, J as Integer
For (I = 1; I <= 3; I++)
For (J = 4; J <= 5; J++)
Write I * J
End For (J)
End For (I)
Question 19
If a counter named MyCount in a For loop has the value of 5 on the first pass, 10 on the second pass, 15 on the third pass, and so on, the increment would be written as __________. (Do not include spaces in your answer).
Question 20
The ____________ statement and
Question 21
How many times will the Write statement be executed based on the following pseudocode?
Declare HelloCount As Integer
Set HelloCount = 1
Repeat
Repeat
Write "Hello"
Set HelloCount = HelloCount = HelloCount + 1
Until HelloCount <=1
Until HelloCount == 2
Question 22
The statement
If Int(Number) != Number Then...
checks to see if the value of Number is a(n) _________.
Question 23
Complete the test condition to allow the following pseudocode that validates the input data.
Declare Number As Float
While Number ______ Int(Number)
Write Enter an integer:
Input Number
End While
Question 24
In a program with nested loops, the outer loop is completed __________ (before/after) the inner loop.
Question 25
The function that returns the number of characters in a string is the __________ function. (For your answer, enter only the function, not any parameters that the function can carry.)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
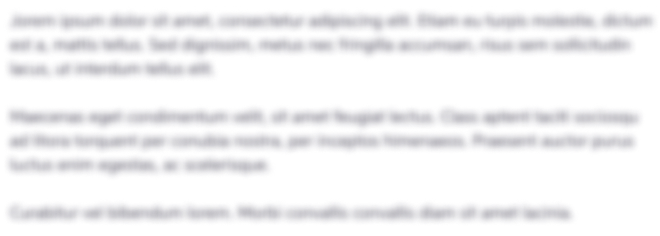
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started