Question
Question 1: Writing string code (15 points) Question 1 provides practice with string operations namely the extraction of words from a sentence. This is a
Question 1: Writing string code (15 points)
Question 1 provides practice with string operations namely the extraction of words from a sentence. This is a very useful program to have in ones toolbox almost every larger program Ive written in the last few years has required this functionality. Details are below. For this question, you will need to use
the string find function, which can take 2 arguments: the string we are looking for, and the index where to start looking
the string len function
the string splicing operator [ < num1 > : < num2 > ]
What should my program do? The program should ask the user to input a sentence, and it should output all the words in that sentence, one per line. Caveat : you must use the provided algorithm on the next page for your code (i.e., even though there are other ways to write the code, e.g., via string concatenation, for this question, you must use the find/string spicing method, described on the next page).
Sample output *:
* Note that the user enters a single sentence, and the program outputs the words in that sentence to the python shell (not a file), one per line.
You must use Algorithm A, which includes using the built in function find and string splicing operator (see next page):
Algorithm A:
ask the user for a sentence
variable last_index will store the starting location of a given word in the input sentence initialize it to 0
while True
use find to find the next blank (i.e., ) in the sentence by asking find to start looking for the blank at index last_index AND assign the index returned by find to a variable called blank_index
if there are still blanks to be processed (i.e., find did not return -1)
- use the splice operator to extract a word from last_index up to blank_index and print the word
- update last_index to be the location directly after blank_index (i.e., assign blank_index + 1 to last_index)
otherwise there are no more blanks to be processed (this means blank_index is equal to -1)
use the splice operator to extract the last word from the users string (starting at last_index, and ending at the last character in the string, obtained by using the len function) and print that word
break out of the loop
To make things more concrete, we will trace the above algorithm with the sentence my cat is cute. Here is a visual of that sentence (AKA string), which includes the index of each character in that string:
Trace of the algorithm:
- Ask the user for a sentence (for this simulation, assume it is my cat is cute); last_index is initialized to 0
Iteration 1 of the loop:
- we call find, asking it to start looking for a blank starting at position last_index (which is 0)
- find returns 2 (the index of the first blank), which we assign to blank_index
- we use the slice operator to grab the characters between last_index (which is 0) and blank_index (which is 2), so we grab my, and we print that word
- last_index is assigned the value of blank_index +1, so last_index is now 3
Iteration 2 of the loop:
- we call find, asking it to start looking for a blank starting at position last_index (which is 3).
- find returns 6 (the index of the second blank), which we assign to blank_index
- we use the slice operator to grab the characters between last_index (which is 3) and the current blank_index (which is 6), so we grab cat, and we print that word
- last_index is assigned the value of blank_index +1, so last_index is now 7
Iteration 3 of the loop:
- we call find, asking it to start looking for a blank starting at position last_index (which is 7)
- find returns 9 (the index of the third blank), which we assign to blank_index
- we use the slice operator to grab the characters between last_index (which is 7) and the current blank_index (which is 9), so we grab is, and we print that word
- last_index is assigned the value of blank_index +1, so last_index is now 10
Iteration 4 of the loop:
- we call find, asking it to start looking for a blank starting at position last_index (which is 10)
- find returns -1 (there are no more blanks)
- we use the slice operator to grab all the characters between last_index (which is 10) to the end of the string, which we get by using the len function -- this pulls out the word cute. We print it. We are done.
Test your program, and then save it as A3_1.py. Upload this file to cuLearn.
Question 2: Files (15 points)
What should my program do? This program will read test scores from a file (line by line), store them in a list, and output the median value. Please use Algorithm B to do this question - details are below. You do not need to use exceptions (i.e., you can assume that the user will enter a valid file name).
Assume the test scores are stored in a file with the following format:
testScore1
testScore2
< etc the number of lines is not known beforehand>
How do I get the median value?
To get the median value
we need the elements of a sequence (here, test scores) to be sorted
given a sorted sequence of test scores, the median value depends on whether we have an odd or an even number of test scores:
If the sequence has an odd number of test scores (i.e., len of sequence is odd), the median is the middle value. Ex.:
Given <65 70 75 81 90>, the middle value is 75
If we instead have an even number of test scores, the middle value is the average of the two middle most scores. Ex.:
Given <50 65 70 72 81 90>, the middle value is (70 + 72) / 2, i.e., 71
Algorithm B:
Initialize a list to be an empty list (this list will store the test scores we will read from the file)
Ask the user for a file name and open the file for reading
For each line in the file
Convert the test score in the current line to a number (since we need numeric data)
Add the test score to the list (either using append or insert, up to you)
Close the file
Sort the list using the built in python function sort (see hint 1 below)
if the list length is odd (see hint 2 below)
median gets assigned the middle value of that sequence (i.e., the element at position list_length divided by 2, see hint 3 below)
otherwise
val1 gets the value at index list_length divided by 2 (see hint 3)
val2 gets the value at index list_length divided by 2 1 (see hint 3)
median gets assigned the average of val1 and val2 (i.e., val1 + val2 / 2)
print the median to the shell (not to a file)
Hint1: <list name>.sort() will sort the list (e.g., if you have a list called l2 then l2.sort() sorts the list)
Hint2: to check if list length is even, we first have to assign the number of elements in the list to some variable, lets call it list_len (hint ; the number of elements is obtained by calling len). Then, we can use the syntax:
if list_len % 2 != 0: #remainder of dividing by 2 is not zero, so we have an odd number of elements
< deal with the odd case, see blue algorithm above >
else: # remainder of dividing by 2 is zero, so we have an even number of elements
How does the above code work? % is a python operator that returns the remainder of dividing two numbers (where remainder can be 0 or some other number). To illustrate, if list_len is 7, then 7 % 2 evaluates to 1 (because the remainder of dividing 7 by 2 is 1), so this tells us we have an odd number of elements. If list_len is 6, 6 % 2 evaluates to 0 (because the remainder of dividing 6 by 2 is 0), so we know we have an even number of elements.
Hint3: to get the index of the middle value, we have to use division that results in an integer, since the index operator [ ] expects an integer (rather than a float). Thus, we MUST use // (instead of just /) for division. Example : 9 // 2 gives us 4; 8 // 2 gives us 4.
To test your program, create a text file using notepad or textedit if you use Word, make sure you save it as PLAIN TEXT (use SaveAs and then select Plain Text). The file should have with a series of test scores, as follows:
one number PER line
the numbers should NOT be sorted (since thats the job of your program)
create one test file with an even number of scores and one with an odd number of scores and test your program with each.
Sample text file with an even number of scores:
Sample text file with an odd number of scores:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
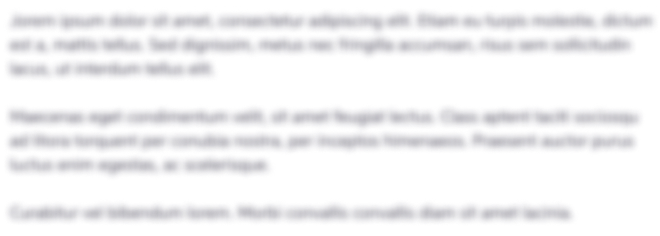
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started