Question
Question 2: Identify the output of the following Java code. Justify the syntax. File Name:ColorCheckBoxWindow.java (it can be downloaded from Moodle under Week 8 Lecture
Question 2: Identify the output of the following Java code. Justify the syntax.
File Name:ColorCheckBoxWindow.java (it can be downloaded from Moodle under Week 8 Lecture 8 T322 Resources)
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ColorCheckBoxWindow extends JFrame
{
private JLabel messageLabel;
private JCheckBox yellowCheckBox;
private JCheckBox redCheckBox;
private final int WINDOW_WIDTH = 300;
private final int WINDOW_HEIGHT = 100;
public ColorCheckBoxWindow()
{
setTitle("Color Check Boxes");
setSize(WINDOW_WIDTH, WINDOW_HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
messageLabel = new JLabel("Select the check " +
"boxes to change colors.");
yellowCheckBox = new JCheckBox("Yellow background");
redCheckBox = new JCheckBox("Red foreground");
yellowCheckBox.addItemListener(new CheckBoxListener());
redCheckBox.addItemListener(new CheckBoxListener());
setLayout(new FlowLayout());
add(messageLabel);
add(yellowCheckBox);
add(redCheckBox);
setVisible(true);
}
private class CheckBoxListener implements ItemListener
{
public void itemStateChanged(ItemEvent e)
{
if (e.getSource() == yellowCheckBox)
{
if (yellowCheckBox.isSelected())
{
getContentPane().setBackground(Color.yellow);
yellowCheckBox.setBackground(Color.yellow);
redCheckBox.setBackground(Color.yellow);
}
else
{
getContentPane().setBackground(Color.lightGray);
yellowCheckBox.setBackground(Color.lightGray);
redCheckBox.setBackground(Color.lightGray);
}
}
else if (e.getSource() == redCheckBox)
{
if (redCheckBox.isSelected())
{
messageLabel.setForeground(Color.red);
yellowCheckBox.setForeground(Color.red);
redCheckBox.setForeground(Color.red);
}
else
{
messageLabel.setForeground(Color.black);
yellowCheckBox.setForeground(Color.black);
redCheckBox.setForeground(Color.black);
}
}
}
}
public static void main(String[] args)
{
new ColorCheckBoxWindow();
}
}
File Name:ColorWindow.java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ColorWindow extends JFrame
{
private JLabel messageLabel;
private JButton redButton;
private JButton blueButton;
private JButton yellowButton;
private JPanel panel;
private final int WINDOW_WIDTH = 200;
private final int WINDOW_HEIGHT = 125;
public ColorWindow()
{
setTitle("Colors");
setSize(WINDOW_WIDTH, WINDOW_HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
messageLabel = new JLabel("Click a button to " +
"select a color.");
redButton = new JButton("Red");
blueButton = new JButton("Blue");
yellowButton = new JButton("Yellow");
redButton.addActionListener(new RedButtonListener());
blueButton.addActionListener(new BlueButtonListener());
yellowButton.addActionListener(new YellowButtonListener());
panel = new JPanel();
panel.add(messageLabel);
panel.add(redButton);
panel.add(blueButton);
panel.add(yellowButton);
add(panel);
setVisible(true);
}
private class RedButtonListener implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
panel.setBackground(Color.RED);
messageLabel.setForeground(Color.BLUE);
}
}
private class BlueButtonListener implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
panel.setBackground(Color.BLUE);
messageLabel.setForeground(Color.YELLOW);
}
}
private class YellowButtonListener implements ActionListener
{
public void actionPerformed(ActionEvent e)
{
panel.setBackground(Color.YELLOW);
messageLabel.setForeground(Color.BLACK);
}
}
public static void main(String[] args)
{
new ColorWindow();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
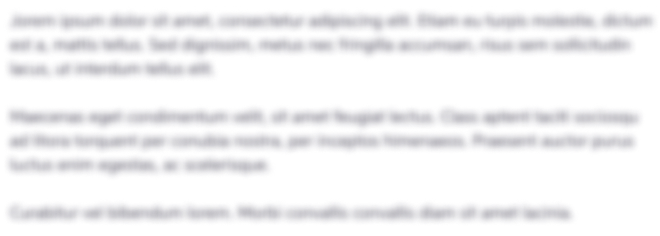
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started