Question
Question C# (Modifying the following changes) QUESTION: Modify the Library example for the following changes *Create a new Interface: interface IDigital { uint LengthInSeconds {
Question C# (Modifying the following changes)
QUESTION:
Modify the Library example for the following changes
*Create a new Interface:
interface IDigital
{
uint LengthInSeconds { get; set; }
DigitalDisc.DiscType MediaType { get; set; }
// following method will return Hours, Minutes, Sec
// for the duration of digital media (lengthSeconds)
// e.g., 1:30:25
string getHMS();
}
*Implement IDigital interface with Music, Movie and AudioBook class:
Make other necessary changes in application to retain the functionality
Add lines in Main() method to check-out and check-in a Music item, Movie item and AudioBook item one each
From Main() method, print duration for each of Music item, Movie item and AudioBook item in HH:MM:SS format (no zero padding required)
*Create a new List as a private member of Item class:
Implement necessary methods to get/set an element publicly for this List
*Implement IComparable interface which compares based on year field of Item class:
*Implement ItemCollection class which implements IEnumerable interface:
Use Dictionary to list all Items accessible by year
Dictionary requires unique key. So have only one item per year for this example
Implement Indexer to access items by value of year
*At the end of Main() method:
Print all (unsorted) elements of List using Iterator
Sort elements of List based on year
Print all (sorted) elements of List using Iterator
Print all elements in dictionary collection using Indexer
*Add a method to read Items from a text file using Regex to parse the entries where each entry is in the following format per line:
Type: Book Year: 1991 Title: This is book 1 ISBN:1234567890 Pages: 500 Authors: Smith, John; Brown, Jack
And likewise for other media types also
Using above data, populate List and Dictionary
*Add a method to write the LibraryCatalog.txt file which dumps all entries in plain text format (as per above mentioned format)
===================================================================================================================================================================================================
CODE: Library Example:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Library
{
class Library
{
// Create Users
public Borrower user1 = new Borrower("user1", "1234");
public Borrower user2 = new Borrower("user2", "1111");
public Admin admin = new Admin("admin", "9999");
// Create Authors
public Author author1 = new Author("Smith", "John");
public Author author2 = new Author("Brown", "Jack");
// Add Books
public Book book1 = new Book(1234567890, 2005, 1000, "This is book 1");
public Book book2 = new Book(1122334455, 2006, 1500, "This is book 2");
// Add eBooks
public eBook ebook1 = new eBook(1234512345, 2005, 1000, "This is eBook 1", @"/eBook/book3");
public eBook ebook2 = new eBook(1212121212, 2006, 1500, "This is eBook 2", @"/eBook/book4");
// Add AudioBooks
public AudioBook audioBook1 = new AudioBook(2233445566, 2005, "This is audio book 1",
DigitalDisc.DiscType.DVD, 7200);
public AudioBook audioBook2 = new AudioBook(3344556677, 2005, "This is audio book 2",
DigitalDisc.DiscType.CD, 3600);
// Add Movies
public Movie movie1 = new Movie(2008, "This is movie 1", DigitalDisc.DiscType.BlueRay, 7200);
public Movie movie2 = new Movie(2007, "This is movie 2", DigitalDisc.DiscType.DVD, 8000);
// Add Music
public Music music1 = new Music(2001, "This is music 1", DigitalDisc.DiscType.CD, 3000);
public Music music2 = new Music(2002, "This is music 2", DigitalDisc.DiscType.DVD, 3500);
public Library()
{
// set the authors
book1.setAuthors(author1, author2);
book2.setAuthors(author2);
ebook1.setAuthors(author2, author1, new Author("Miller", "Julie"));
ebook2.setAuthors(author1, author2);
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class Item
{
protected string title;
protected ushort year;
private Author[] authors = new Author[500];
public string printAuthors
{
get
{
string s = String.Empty;
int i = 0;
while (authors[i] != null)
{
if (i > 0)
{
s += "; ";
}
s += authors[i].LastName + ", " + authors[i].FirstName;
i++;
}
return s;
}
}
public Item()
{
title = String.Empty;
year = 0;
authors[0] = new Author();
}
public Item(string t, ushort y)
{
title = t;
year = y;
}
public void setAuthors(params Author[] a)
{
for (int i = 0; i < a.Length; i++)
{
authors.SetValue(a[i], i);
}
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class Author
{
private string lastName;
private string firstName;
public string LastName
{
get { return lastName; }
set { lastName = value; }
}
public string FirstName
{
get { return firstName; }
set { firstName = value; }
}
public Author()
{
lastName = String.Empty;
firstName = String.Empty;
}
public Author(string last, string first)
{
lastName = last;
firstName = first;
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class User
{
private string uName;
public string UserName
{
get { return uName; }
set { uName = value; }
}
private string passwd;
public string Password
{
get { return passwd; }
set { passwd = value; }
}
private bool isAdmin = false;
protected bool IsAdmin
{
get { return isAdmin; }
set { isAdmin = value; }
}
public DateTime memberSince { get; set; }
public DateTime lastLogin { get; set; }
public User()
{
uName = String.Empty;
passwd = String.Empty;
}
public User(string un, string pass)
{
uName = un;
passwd = pass;
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class Admin : User
{
public Admin()
: base()
{
}
public Admin(string un, string pass)
: base(un,pass)
{
IsAdmin = true;
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Test Program for Library Application");
Console.WriteLine();
Library myLib = new Library();
// check-out one book
myLib.book1.issue(myLib.user1);
// try to check-out the same book by another user
myLib.book1.issue(myLib.user2);
// return the book
myLib.book1.returnIt();
// hold a book
myLib.ebook1.holdIt(myLib.user2);
// try to check-out the same book by another user
myLib.ebook1.issue(myLib.user1);
// remove hold
myLib.ebook1.removeHolds();
Console.WriteLine();
// print authors
Console.WriteLine(myLib.book1.printAuthors);
Console.WriteLine(myLib.ebook1.printAuthors);
Console.Read();
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class Music : Item, Iloanable
{
private DigitalDisc.DiscType mediaType;
internal DigitalDisc.DiscType MediaType
{
get { return mediaType; }
set { mediaType = value; }
}
private uint lengthSeconds;
public uint LengthInSeconds
{
get { return lengthSeconds; }
set { lengthSeconds = value; }
}
public Music()
: base()
{
lengthSeconds = 0;
mediaType = DigitalDisc.DiscType.CD;
}
public Music(ushort myYear, string myTitle, DigitalDisc.DiscType disc, uint sec)
: base(myTitle, myYear)
{
mediaType = disc;
lengthSeconds = sec;
}
#region Iloanable Members
public User currentUser { get; set; }
public DateTime checkOutTime { get; set; }
public DateTime checkInTime { get; set; }
public bool isAvailable { get; set; }
public bool isOnHold { get; set; }
public void issue(User u)
{
if (isAvailable)
{
currentUser = u;
checkOutTime = DateTime.Now;
checkInTime = DateTime.MinValue;
isAvailable = false;
isOnHold = false;
Console.WriteLine(title + " is issued to " + currentUser.UserName + " at " + checkOutTime);
}
else
{
Console.WriteLine(title + " is not available to check-out");
}
}
public void returnIt()
{
if (!isAvailable)
{
checkInTime = DateTime.Now;
Console.WriteLine(title + " is returned by " + currentUser.UserName + " at " + checkInTime);
currentUser = null;
isAvailable = true;
isOnHold = false;
}
else
{
Console.WriteLine("This item is not checked-out yet");
}
}
public void holdIt(User u)
{
if (isAvailable && !isOnHold)
{
currentUser = u;
isAvailable = true;
isOnHold = false;
checkOutTime = DateTime.Now;
Console.WriteLine(title + " is on hold for " + currentUser.UserName + " at " + checkOutTime);
}
else
{
Console.WriteLine("This item can not be put on hold");
}
}
public void removeHolds()
{
if (!isAvailable && isOnHold)
{
currentUser = null;
isAvailable = true;
isOnHold = false;
checkOutTime = DateTime.MaxValue;
Console.WriteLine("Hold is removed");
}
else
{
Console.WriteLine("This item is not on hold");
}
}
#endregion
}
}
--------------------------------------------------------------------------------
namespace Library
{
class Movie : Item, Iloanable
{
private DigitalDisc.DiscType mediaType;
internal DigitalDisc.DiscType MediaType
{
get { return mediaType; }
set { mediaType = value; }
}
private uint lengthSeconds;
public uint LengthInSeconds
{
get { return lengthSeconds; }
set { lengthSeconds = value; }
}
public Movie()
: base()
{
lengthSeconds = 0;
mediaType = DigitalDisc.DiscType.CD;
}
public Movie(ushort myYear, string myTitle, DigitalDisc.DiscType disc, uint sec)
: base(myTitle,myYear)
{
mediaType = disc;
lengthSeconds = sec;
}
#region Iloanable Members
public User currentUser { get; set; }
public DateTime checkOutTime { get; set; }
public DateTime checkInTime { get; set; }
public bool isAvailable { get; set; }
public bool isOnHold { get; set; }
public void issue(User u)
{
if (isAvailable)
{
currentUser = u;
checkOutTime = DateTime.Now;
checkInTime = DateTime.MinValue;
isAvailable = false;
isOnHold = false;
Console.WriteLine(title + " is issued to " + currentUser.UserName + " at " + checkOutTime);
}
else
{
Console.WriteLine(title + " is not available to check-out");
}
}
public void returnIt()
{
if (!isAvailable)
{
checkInTime = DateTime.Now;
Console.WriteLine(title + " is returned by " + currentUser.UserName + " at " + checkInTime);
currentUser = null;
isAvailable = true;
isOnHold = false;
}
else
{
Console.WriteLine("This item is not checked-out yet");
}
}
public void holdIt(User u)
{
if (isAvailable && !isOnHold)
{
currentUser = u;
isAvailable = true;
isOnHold = false;
checkOutTime = DateTime.Now;
Console.WriteLine(title + " is on hold for " + currentUser.UserName + " at " + checkOutTime);
}
else
{
Console.WriteLine("This item can not be put on hold");
}
}
public void removeHolds()
{
if (!isAvailable && isOnHold)
{
currentUser = null;
isAvailable = true;
isOnHold = false;
checkOutTime = DateTime.MaxValue;
Console.WriteLine("Hold is removed");
}
else
{
Console.WriteLine("This item is not on hold");
}
}
#endregion
}
}
--------------------------------------------------------------------------------
namespace Library
{
interface Iloanable
{
User currentUser { get; set; }
DateTime checkOutTime { get; set; }
DateTime checkInTime { get; set; }
bool isAvailable { get; set; }
bool isOnHold { get; set; }
void issue(User u);
void returnIt();
void holdIt(User u);
void removeHolds();
}
}
--------------------------------------------------------------------------------
namespace Library
{
class ReferenceBook : Item
{
private readonly uint isbn;
public uint ISBN
{
get { return isbn; }
}
private readonly uint pages;
public uint Pages
{
get { return pages; }
}
public ReferenceBook()
: base()
{
isbn = 0;
pages = 0;
}
public ReferenceBook(uint myISBN, ushort myYear, uint myPages, string myTitle)
: base(myTitle, myYear)
{
isbn = myISBN;
pages = myPages;
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class eBook : Book
{
private string filePath = String.Empty;
public string FilePath
{
get { return filePath; }
set { filePath = value; }
}
public eBook()
: base()
{
filePath = String.Empty;
}
public eBook(uint myISBN, ushort myYear, uint myPages, string myTitle, string fileP)
: base(myISBN, myYear, myPages, myTitle)
{
filePath = fileP;
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class DigitalDisc
{
public enum DiscType { CD, DVD, BlueRay }
}
}
--------------------------------------------------------------------------------
namespace Library
{
class Borrower : User
{
public Borrower()
: base()
{
IsAdmin = false;
}
public Borrower(string un, string pass)
: base(un, pass)
{
IsAdmin = false;
}
}
}
--------------------------------------------------------------------------------
namespace Library
{
class Book : Item, Iloanable
{
private readonly uint isbn;
public uint ISBN
{
get { return isbn; }
}
private readonly uint pages;
public uint Pages
{
get { return pages; }
}
public Book()
: base()
{
isbn = 0;
pages = 0;
isAvailable = false;
isOnHold = false;
}
public Book(uint myISBN, ushort myYear, uint myPages, string myTitle)
: base(myTitle,myYear)
{
isbn = myISBN;
pages = myPages;
isAvailable = true;
isOnHold = false;
}
#region Iloanable Members
public User currentUser { get; set; }
public DateTime checkOutTime { get; set; }
public DateTime checkInTime { get; set; }
public bool isAvailable { get; set; }
public bool isOnHold { get; set; }
public void issue(User u)
{
if (isAvailable)
{
currentUser = u;
checkOutTime = DateTime.Now;
checkInTime = DateTime.MinValue;
isAvailable = false;
isOnHold = false;
Console.WriteLine(ISBN + " is issued to " + currentUser.UserName + " at " + checkOutTime);
}
else
{
Console.WriteLine(ISBN + " is not available to check-out");
}
}
public void returnIt()
{
if (!isAvailable)
{
checkInTime = DateTime.Now;
Console.WriteLine(ISBN + " is returned by " + currentUser.UserName + " at " + checkInTime);
currentUser = null;
isAvailable = true;
isOnHold = false;
}
else
{
Console.WriteLine("This item is not checked-out yet");
}
}
public void holdIt(User u)
{
if (isAvailable && !isOnHold)
{
currentUser = u;
isAvailable = false;
isOnHold = false;
checkOutTime = DateTime.Now;
Console.WriteLine(ISBN + " is on hold for " + currentUser.UserName + " at " + checkOutTime);
}
else
{
Console.WriteLine("This item can not be put on hold");
}
}
public void removeHolds()
{
if (!isAvailable && isOnHold)
{
currentUser = null;
isAvailable = true;
isOnHold = false;
checkOutTime = DateTime.MaxValue;
Console.WriteLine("Hold is removed");
}
else
{
Console.WriteLine("This item is not on hold");
}
}
#endregion
}
}
--------------------------------------------------------------------------------
namespace Library
{
class AudioBook : Book
{
private DigitalDisc.DiscType mediaType;
internal DigitalDisc.DiscType MediaType
{
get { return mediaType; }
set { mediaType = value; }
}
private uint lengthSeconds;
public uint LengthSeconds
{
get { return lengthSeconds; }
set { lengthSeconds = value; }
}
public AudioBook()
: base()
{
lengthSeconds = 0;
mediaType = DigitalDisc.DiscType.CD;
}
public AudioBook(uint myISBN, ushort myYear, string myTitle, DigitalDisc.DiscType disc, uint sec)
: base(myISBN, myYear, 0, myTitle)
{
mediaType = disc;
lengthSeconds = sec;
}
}
}
--------------------------------------------------------------------------------
--------------------------------------------------------------------------------
Step by Step Solution
There are 3 Steps involved in it
Step: 1
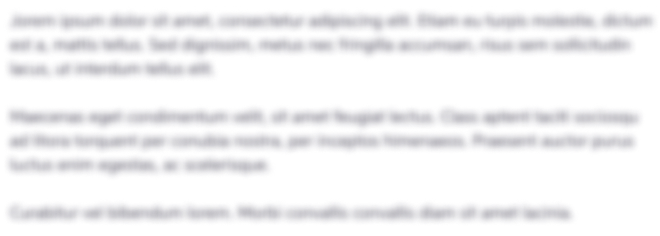
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started