Question
// Question finish struct container * setfbt // When a user buys a book, the frequently bought togther(fbt) linked list should be updated for each
// Question finish struct container * setfbt // When a user buys a book, the frequently bought togther(fbt) linked list should be updated for each book. // The update in fbt list should be done for each book in the buying list. // For simplicity, you do not consider the number of times the books are bought.
struct container * setfbt (struct container * in){
}
// If you modify any of the given code, return types, or parameters, you risk failing test cases.
// The following will be accepted as input in the following format: "title:author:price"
// Example Input: "ProgrammingLanguages:Chen:45"
// Valid title: String containing alphabetical letters beginning with a capital letter
// Valid author: String containing alphabetical letters beginning with a capital letter
// Valid price: A float variable
#include
#include
#include
#include
#pragma warning(disable: 4996)
// used to create a linked list of containers, each contaning a "book"
struct container {
struct book *book;
struct container *next;
} *list = NULL;
// used to hold book information and linked list of "frequently bought together (fbt)"
struct book {
char title[30];
char author[30];
float price;
struct fbt *fbts;
};
// used to create a linked list of frequently bought together (fbt)
struct fbt {
struct book *book;
struct fbt *next;
};
// forward declaration of functions that have already been implemented
void flush();
void branching(char);
void registration(char);
// the following forward declarations are for functions that require implementation
// HW07
// Total: 50 points for hw07
// return type // name and parameters // points
void add_book(char*, char*,float); // 15
struct book* search_book(char*); // 10
void remove_book(char*); // 15
void print_all_books(); // 10
// HW08
// Total: 50 points for hw08
struct container * buy_book(); // 15
struct container * setfbt(struct container *); // 25
void display_fbt(struct container*); // 10
int main()
{
char ch = 'i';
printf("Book Information ");
do
{
printf("Please enter your selection: ");
printf("\ta: add a new book to the list ");
printf("\ts: search for a book on the list ");
printf("\tr: remove a book from the list ");
printf("\tl: print the list of all books ");
printf("\tb: buy a book ");
printf("\tq: quit ");
ch = tolower(getchar());
flush();
branching(ch);
} while (ch != 'q');
return 0;
}
// consume leftover ' ' characters
void flush()
{
int c;
do c = getchar(); while (c != ' ' && c != EOF);
}
// branch to different tasks
void branching(char c)
{
switch (c)
{
case 'a':
case 's':
case 'r':
case 'l':registration(c); break;
case 'b':{
struct container * buy = buy_book();
buy = setfbt(buy);
display_fbt(buy);
break;
}
case 'q': break;
default: printf("Invalid input! ");
}
}
//
// This function will determine what info is needed and which function to send that info to.
// It uses values that are returned from some functions to produce the correct ouput.
// There is no implementation needed here, but you should trace the code and know how it works.
// It is always helpful to understand how the code works before implementing new features.
// Do not change anything in this function or you risk failing the automated test cases.
void registration(char c)
{
if (c == 'a')
{
char input[100];
printf(" Please enter the book's info in the following format: ");
printf("title:author:price ");
fgets(input, sizeof(input), stdin);
// discard ' ' chars attached to input
input[strlen(input) - 1] = '\0';
char* title = strtok(input, ":"); // strtok used to parse string
char * author = strtok(NULL, ":");
float price = atof(strtok(NULL, ":"));
struct book* result = search_book(title);
if (result == NULL)
{
add_book(title, author, price);
printf(" Book added to list successfully ");
}
else
printf(" That book is already on the list ");
}
else if (c == 's' || c == 'r' )
{
char title[30];
printf(" Please enter the book's title: ");
fgets(title, sizeof(title), stdin);
// discard ' ' chars attached to input
title[strlen(title) - 1] = '\0';
struct book* result = search_book(title);
if (result == NULL)
printf(" That book is not on the list ");
else if (c == 's'){
printf(" Title: %s ", result->title);
printf("Author: %s ", result->author);
printf("Price: %.2f ", result->price);
}
else
{
remove_book(title);
printf(" Book removed from the list ");
}
}
else
{
print_all_books();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
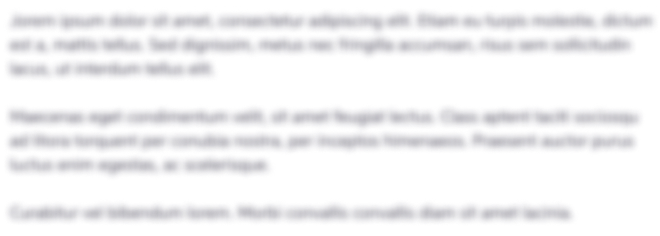
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started