Question
Question for Java GUI Lab I want to change the color of a label if I click the button. There are four different color of
Question for Java GUI Lab
I want to change the color of a label if I click the button. There are four different color of buttons, and if I click the Red button, then the label is going to be red, etc...
Can someone help my code??
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
public class Csci230_buttonaction extends JFrame implements ActionListener {
public Csci230_buttonaction() {
super("Lab1");
setLayout(new BorderLayout());
JLabel label = new JLabel("Color");
label.setHorizontalAlignment(JLabel.CENTER);
add(label, BorderLayout.CENTER);
JButton button1 = new JButton("Red");
JButton button2 = new JButton("Black");
JButton button3 = new JButton("Green");
JButton button4 = new JButton("Blue");
button1.setSize(60, 20);
button2.setSize(60, 20);
button3.setSize(60, 20);
button4.setSize(60, 20);
button1.setForeground(Color.red);
button2.setForeground(Color.black);
button3.setForeground(Color.green);
button4.setForeground(Color.blue);
add(button1, BorderLayout.NORTH);
add(button2, BorderLayout.SOUTH);
add(button3, BorderLayout.EAST);
add(button4, BorderLayout.WEST);
button1.addActionListener(this);
button2.addActionListener(this);
button3.addActionListener(this);
button4.addActionListener(this);
pack();
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if(e.getActionCommand() == "red"){
setForeground(Color.red);
} else if(e.getActionCommand() == "Black"){
setForeground(Color.black);
} else if(e.getActionCommand() == "Green"){
setForeground(Color.green);
} else if(e.getActionCommand() == "Blue"){
setForeground(Color.blue);
}
}
public static void main(String[] args) {
Csci230_buttonaction frame;
frame = new Csci230_buttonaction();
frame.setSize(300, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
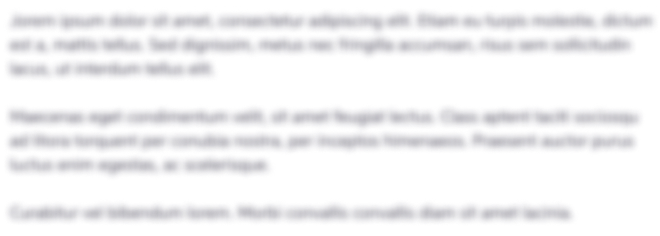
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started