Question
Question: Introduction In this assignment, you will create a memory allocation simulator. You will be evaluated only on the correctness of your simulated heap, so
Question:
Introduction
In this assignment, you will create a memory allocation simulator. You will be evaluated only on the correctness of your simulated heap, so you don't have to worry about throughput. You may use any programming language you choose from the following options:
C
C++
Java
Ruby
Python
You will not actually make any memory calls in your program. You will simply operate on a simulated heap. You may make all the decisions about how you represent your simulated heap, but you will need to accept input and produce output according to the specifications that follow in the "Specifications" section.
You read a series of allocation, reallocation, and free calls from an input text file, detailed in the "Specifications" section below, and process them using both implicit and explicit free list approaches. You must use both headers and footers for blocks in both approaches. You will also allow the user to choose whether to use a First-fit or Best-fit approach to allocating memory blocks. For all cases, you will use immediate coalescing of free blocks.
All work should be your own. You are prohibited from using any code that is not your own and from working in groups. If you find examples of HOW to do something on the internet or elsewhere you must reference the source and what you used it for in your report. For example, you may look for examples on how to write output to a file, as long as you give credit to the source in your references and specify the reason you referenced that resource. Any use of code that is not your own will be considered to be academic dishonesty. You MUST write your own code (no use of ANY code from the internet or elsewhere) for the four primary functions (myalloc, myrealloc, myfree, mysbrk) in the assignment.
Specifications
Your Heap
Start your heap at address 0
Assume a 32-bit system (so each word is 4 bytes)
Assume double-word alignment of all allocated blocks
Calls to myalloc and myrealloc take an input parameter indicating the size of the allocation in bytes
Invalid calls to any of your primary functions will give an error but not crash your simulator (though they may have no effect on the heap)
Your initial heap size will be 1000 words, and you may expand your heap to 100,000 words maximum
As an example, your heap would start at word 0. If your first call is myalloc(5), then you would start the header at word 1, your payload at word 2, and your footer at word 4 to meet alignment requirements. This is because the payload would have to start at an address divisible by 8, and take up two words, 5 bytes for the payload and 3 bytes of padding. So, your header would start at word 1 (address 4), the payload would start at word 2 (address 8), and your footer would start at word 4 (address 16). This would allow your next header to start at word 5 (address 20) and the next payload to start at word 6 (address 24).
|header| payload |footer |
|--------|--------|--------|--------|--------|--------|
0 1 2 3 4 5 6
Primary Functions
You will have four primary functions in your assignment, which MUST be named as follows:
myalloc(size)
takes an integer value indicating the number of bytes to allocate for the payload of the block
returns a "pointer" to the starting address of the payload of the allocated block
The "pointer" above can take any form you like, depending on the data structure you use to represent your heap
myrealloc(pointer, size)
takes a pointer to an allocated block and an integer value to resize the block to
returns a "pointer" to the new block
frees the old block
a call to myrealloc with a size of zero is equivalent to a call to myfree
myfree(pointer)
frees the block pointed to by the input parameter "pointer"
returns nothing
only works if "pointer" represents a previously allocated or reallocated block that has not yet been freed
otherwise, does not change the heap
mysbrk(size)
grows or shrinks the size of the heap by a number of words specified by the input parameter "size"
you may call this whenever you need to in the course of a simulation, as you need to grow the heap
this call will return an error and halt the simulation if your heap would need to grow past the maximum size of 100,000 words
User Options
The user must be able to specify the following (either in a GUI or on the command line) for each run of your simulator:
Input text file
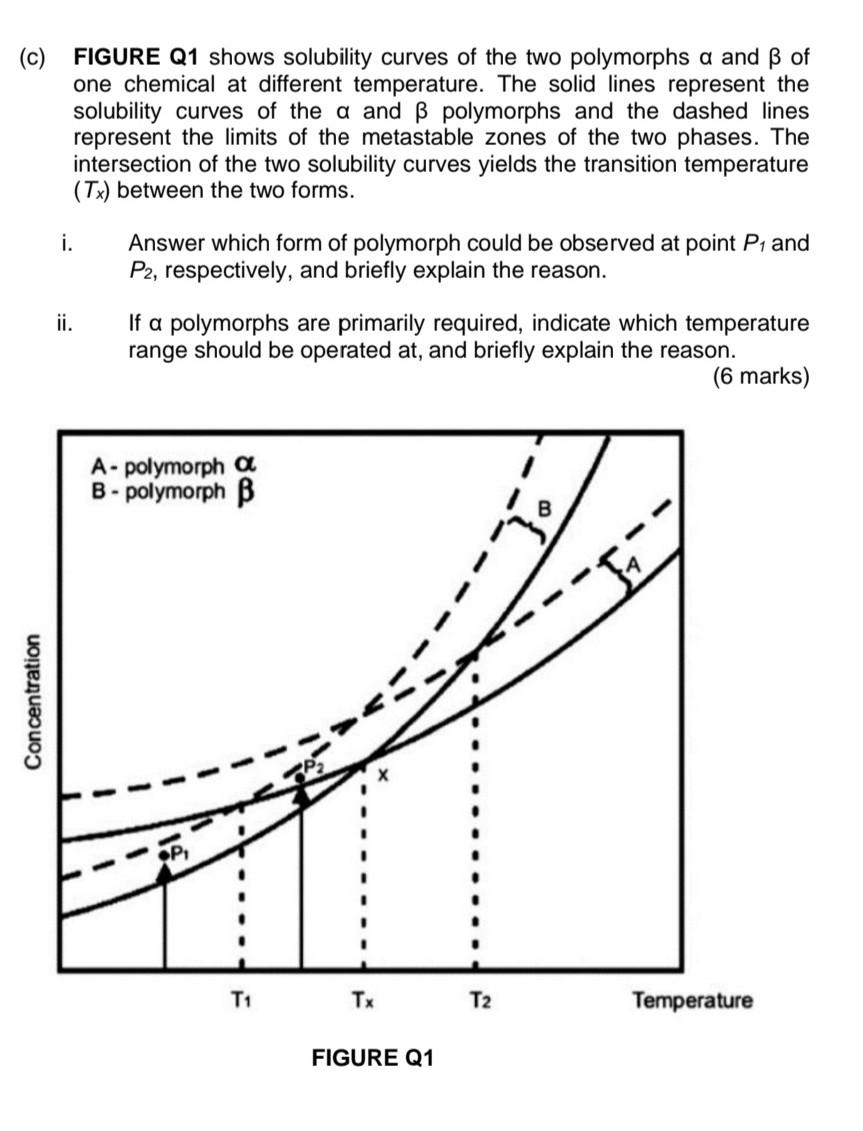
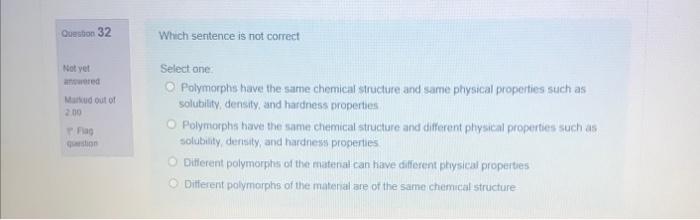
Step by Step Solution
There are 3 Steps involved in it
Step: 1
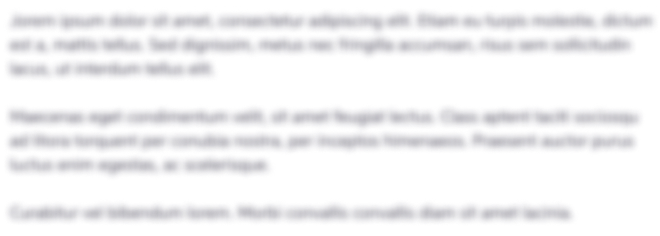
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started