Question
Question is C++ REFERENCE: #include #include #include using namespace std; // the Person class - this is the class from assignment 1 class Person {
Question is C++
REFERENCE:
#include
// the Person class - this is the class from assignment 1 class Person { // the private data members private: string firstName; string lastName; string address;
// the public data members public:
// the constructor - default constructor Person() { // set all the data members to empty setFirstName(""); setLastName(""); setAddress(""); }
// the constructor - that takes in the first name and the last name Person(string firstName, string lastName) { setFirstName(firstName); setLastName(lastName); // set the address to blank setAddress(""); }
// the constructor - that takes in the first name and the last name and address Person(string firstName, string lastName, string address) { setFirstName(firstName); setLastName(lastName); // set the address to blank setAddress(address); }
// getters string getFirstName() { return firstName; } string getLastName() { return lastName; } string getAddress() { return address; }
// setters void setFirstName(string firstName) { this->firstName = firstName; } void setLastName(string lastName) { this->lastName = lastName; } void setAddress(string address) { this->address = address; }
// the print function virtual void print() { cout << firstName << " " << lastName << ", " << address << endl; } };
// the class Player extends the class Person class Player : public Person { // the private data members of this class private: double average; double onBasePercentage; double sluggingPercentage;
// the public data members public:
// the default constructor Player() { // set all the players to 0 setAverage(0); setOBP(0); setSlugging(0); }
// the constructor with all the values Player(string first, string last, string address, double average, double slugging, double onBase) { // set all the data members setFirstName(first); setLastName(last); setAddress(address);
// set the average, onbase and slugging setAverage(average); setOBP(onBase); setSlugging(slugging); }
// setter for the values void setAverage(double average) { this->average = average; } void setSlugging(double slug) { this->sluggingPercentage = slug; } void setOBP(double OnBase) { this->onBasePercentage = OnBase; } // the print void print() { cout << getFirstName() << " " << getLastName() << ", " << getAddress() << ", " << average << ", " << sluggingPercentage << "%, " << onBasePercentage << "%" << endl; } };
// The address book that extends the vector class - Task1 class AddressBook : public vector
// the constructor that takes in a first name and the last name AddressBook(string first, string last) { push_back(new Person(first, last, "")); }
// the constructor that takes in a first name, the last name and the address AddressBook(string first, string last, string address) { push_back(new Person(first, last, address)); }
// the mutator void setPerson(string first, string last, string address) { push_back(new Person(first, last, address)); }
// returns the next person Person* getPerson() { // if there is no person to return - return null if (size() == 0) return NULL;
// return the next person Person* nextPerson = at(index);
// move the index forward index = (index + 1) % size();
// return the next person return nextPerson; }
// find the person with this last name Person* findPerson(string last) { // find the person with this last name for (int i = 0; i < size(); i++) { // if this has the same last name, return this person if (at(i)->getLastName() == last) return at(i); } // return null pointer return NULL; }
// find the person with this first name and last name Person* findPerson(string first, string last) { // find the person with this last name for (int i = 0; i < size(); i++) { // if this has the same last name, return this person if (at(i)->getLastName() == last && at(i)->getFirstName() == first) return at(i); } // return null pointer return NULL; }
// print the entire address book void print() { // print all the persons for (int i = 0; i < size(); i++) { at(i)->print(); } } };
// using the same main as in previous part - no changes to the main // works the same int main() { // create an address Book AddressBook addressBook1;
// since this is empty right now, there is no person inside this if (addressBook1.findPerson("Wayne") != NULL) cout << "Error: Address Book must be empty!" << endl; else cout << "Pass: Address Book is empty when created!" << endl;
// add members to this address book 1 addressBook1.setPerson("Bruce", "Wayne", "Wayne Tower"); addressBook1.setPerson("Clark", "Kent", "Daily Mail"); addressBook1.setPerson("Thor", "Son of Odin", "Asgard");
// look for last name "Wayne" if (addressBook1.findPerson("Wayne") != NULL) cout << "Pass: Person with last name found!" << endl; else cout << "Error: Person with last name not found!" << endl;
// look for first name and last name - to be found if (addressBook1.findPerson("Clark", "Kent") != NULL) cout << "Pass: Person with last name and first name found!" << endl; else cout << "Error: Person with last name and first name not found!" << endl;
// look for first name and last name - not to be found if (addressBook1.findPerson("Bruce", "Banner") == NULL) cout << "Pass: Person with last name and first name not found!" << endl; else cout << "Error: Person with last name and first name found!" << endl;
// change line cout << endl; // print the address book addressBook1.print();
// change line cout << endl;
/********************************************************************************/ /****************************** Task 2 ******************************************/
// create two players Player player1("Steve", "Rogers", "1970 America", 3.56, 12.5, 67.8); Player player2("Diana", "Prince", "Amazon", 5.88, 44.78, 41.8);
// print the players player1.print(); player2.print();
// return 0 return 0; }
QUESTION:
We have been working on a simple address book. The major problem with it at this point is that we lose all of our information when the program ends. It would be nice to store all of this information to a file. That way, the data will always be there for us.
Your mission is to write all of the entries of your address book to a text file. When you write the file you should write one person per line comma separated. For example
Glenn,Stevenson,1313 Mockingbird Lane,951-639-5532 Jane,Smith,30333 South Street,951-555-5325
This way you can use the comma as a delimiter for each field of the record.
In your constructors you should have functionality to load the address book from file and put the contents into your vector. This means you will have to parse out each field for each record. You should also have a save method that will save the entire contents of the vector to the file formatted.
If you are writing this project in C++ you may want to investigate the idea of writing the contents of the address book to file inside a destructor. This is not required here, only something to think about.
In any event, you must have functionality that will write the contents of your address book to file and have a methodology of saving it.
Question:
File IO
We have been working on a simple address book. The major problem with it at this point is that we lose all of our information when the program ends. It would be nice to store all of this information to a file. That way, the data will always be there for us.
Your mission is to write all of the entries of your address book to a text file. When you write the file you should write one person per line comma separated. For example
Glenn,Stevenson,1313 Mockingbird Lane,951-639-5532 Jane,Smith,30333 South Street,951-555-5325
This way you can use the comma as a delimiter for each field of the record.
In your constructors you should have functionality to load the address book from file and put the contents into your vector. This means you will have to parse out each field for each record. You should also have a save method that will save the entire contents of the vector to the file formatted.
If you are writing this project in C++ you may want to investigate the idea of writing the contents of the address book to file inside a destructor. This is not required here, only something to think about.
In any event, you must have functionality that will write the contents of your address book to file and have a methodology of saving it.
Please make the coding in the Vectures and File-O for the Main.cpp
Step by Step Solution
There are 3 Steps involved in it
Step: 1
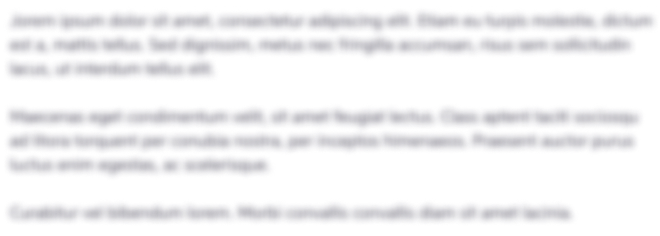
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started