Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Question: Write a program to simulate producer-consumer problem using bounded buffer whose size is 10. (this means the buffer only can store 10 messages.) For
Question: Write a program to simulate producer-consumer problem using bounded buffer whose size is 10. (this means the buffer only can store 10 messages.) For this, there will be one program creating two threads (one for producer and the other for consumer) which act as different processes. After 15 seconds of running, the program (both producer and consumer threads) should be terminated gracefully without any error.
I have this code:
import java.security.SecureRandom; import java.util.concurrent.*; /** * Created by Leon.H on 2016/1/13. */ public class ProducerConsumer { private int producerNumber = 0; private int consumerNumber = 0; private int bufferSize = 0; private final int seconds; public ProducerConsumer(int producerNumber, int consumerNumber, int bufferSize, int seconds) { this.producerNumber = producerNumber; this.consumerNumber = consumerNumber; this.bufferSize = bufferSize; this.seconds = seconds; System.out.println(this.producerNumber+ ": the number of producer threads"); System.out.println(this.consumerNumber+ ": the number of consumer threads"); System.out.println(this.bufferSize+ ": the number of producer threads"); } public void process() throws InterruptedException { ExecutorService producerExecutorService = Executors.newFixedThreadPool(this.producerNumber); ExecutorService consumerExecutorService = Executors.newFixedThreadPool(this.consumerNumber); BlockingQueueintegers = new ArrayBlockingQueue (this.bufferSize); for (int i = 0; i integers; public ProducerTask(BlockingQueue integers) { this.integers = integers; } public void run() { while (true) { Integer content = new SecureRandom().nextInt(1000); System.out.println("Producer #" + Thread.currentThread().getId() + " put: " + content); integers.offer(content); try { Thread.sleep(new SecureRandom().nextInt(1000)); } catch (InterruptedException e) { e.printStackTrace(); } } } } private class ConsumerTask implements Runnable { private final BlockingQueue integers; public ConsumerTask(BlockingQueue integers) { this.integers = integers; } public void run() { while (true) { try { System.out.println("Consumer #" + Thread.currentThread().getId() + " get: " + integers.take()); } catch (InterruptedException e) { e.printStackTrace(); } try { Thread.sleep(new SecureRandom().nextInt(1000)); } catch (InterruptedException e) { e.printStackTrace(); } } } } }
import org.testng.annotations.Test; /** * Created by Leon.H on 2016/1/13. */ public class ProducerConsumerTest { @Test public void oneProducerOneConsumerSizeOne() throws InterruptedException { int ProducerNumber = 15; int ConsumerNumber = 15; int size = 1; int seconds=5; ProducerConsumer producerConsumer = new ProducerConsumer(ProducerNumber, ConsumerNumber, size, seconds); producerConsumer.process(); }
}
How to start integer from 0 to 14 and message from 1 to 10?15: the number of producer threads 15: the number of consumer threads 1: the number of producer threads Producer #30 put: 844 Producer #34 put: 138 Producer #24 put: 824 Producer #32 put: 166 Producer #27 put: 908 Producer #37 put: 673 Producer #28 put: 95 Producer #25 put: 292
Step by Step Solution
There are 3 Steps involved in it
Step: 1
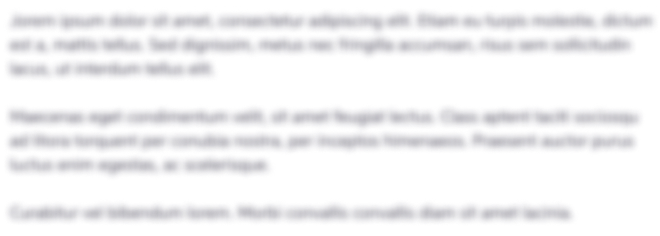
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started