Question
Question1 .) Compiling, linking, and executing program cc, vi or vim or notepad, cat, running program You can type the given program in the vi
Question1 .) Compiling, linking, and executing program cc, vi or vim or notepad, cat, running program
You can type the given program in the vi editor. See below
/*
* Program filename: *** type the actual filename you chose here like in the textbook. ***
*
* Program Description:
* This program reads the content of a given directory and displays it.
* Uses the system call function opendir( ) to open the directory file.
* Uses the system call function readdir( ) to read the directory content one file entry at time
* in a the while loop until there is no more entry (end of file).
* Finally, after reading all the entries, the directory file is closed using the
* system call function closedir( ).
*
* Function: main ( )
* Input parameter:
* Directory name
* Output parameter:
* None
*
* Return value:
* 0, if successful
* -1, if a directory name is not given as argument
* -2, if opening the directory file (opendir) fails
*
* Program Defect:
* This program does not explicitly check for readdir system call failure.
* But returns successful status.
*/
#include
#include
int main (
int argc, /* Number of arguments supplied when running the program */
char **argv /* Values of arguments */
)
{
DIR *dir; /* Directory file pointer returned by opendir */
struct dirent *direntry; /* Information for one file in the directory */
/* returned by readir */
/* Check number of arguments supplied */
if (argc != 2)
{
printf(Run the program specifying one directory name as argument. );
exit (-1); /* return error status */
}
/* open the directory file */
if ((dir = opendir(argv[1])) == NULL)
{
printf(%s directory file open failed. , argv[1]);
exit (-2); /* return error status */
}
/* Read directory entries one file entry at a time until end of file or error */
while ((direntry = readdir(dir)) != NULL) /* until entries are exhausted */
{
printf (%10d %s , direntry->d_ino, direntry->d_name);
}
/* Close directory file */
closedir (dir);
exit (0); /* return successful status */
} /* end main ( ) */
a.) Compile and create an executable file for this program using the cccommand.
b.) The following command will produce a.out as the executable file assuming hw3q1.c is the program file name:
cc hw3q1.c
c.) The following command will produce hw3q1 as the executable file assuming hw3q1.c is the program file name. The file name after the o option is the executable filename.
cc hw3q1.c o hw3q1
d.) Use the second method as it is the preferred choice by professionals and it is easy to identify the executable file and the corresponding .c program file.
e.) Display the content of the program file, which is also called the source code file.
f.) Run the program by typing the executable file name followed by a directory name as argument. The argument could be current working directory or any directory.
g.) Look at the output of the program, then using echo command answer what are the two information stored in a directory file.
h.) Using echo command, answer what are . and .. displayed in the output
Question 2: . C Programming cc, vi or vim or notepad, cat, gets, scanf, printf, strcmp, strtok, running program
Write a C program to perform the following. Read the name of an ftp command, identify whether it is a valid command or not and print a line indicating the command is valid or invalid.
The list of valid ftp command to use for this question is: (1) ascii, (2) recv (and/or get), (3) send (and/or put), (4) rmdir, (5) mkdir, (6) pwd, (7) ls, (8) cd, (9) status and (10) quit.
The pseudo code is given below.
Step 1: Read an ftp command into a character array from keyboard using gets function or scanf.
Step 2: Identify what command it is using strcmpfunction.
Step 3: For valid ftp command print a line of output as: pwd is a valid ftp command
For invalid ftp command print a line of output as: xyz is not a valid ftp command
-Display the content of source code file hw3q2.c.
-Run the executable file which will produce output.
NOTE:You can run the program multiple times testing for valid ftp commands and one invalid command. Alternately, you can write a loop around Step 1 to 3 and loop until quit command is typed.
Depending on the ftp command, it may be just the command name (example: ascii, quit, status) or command name followed by one argument (example: get hw1.c, put hw3q1.c).
-read an ftp command with or without an argument and separate the command and argument using strtok function. Perform the comparison to find out the ftp command is valid, and then print both command and argument like: The ftp command is get and argument is hw1.c
Incorporating this feature is optional. This requires only couple of lines of extra code. You still write one program for Q2.
Create the source code file, compile and create an executable file. Run the program and get the output. You have to test the program using all the ftp commands given above one by one as input.
Print the source code file, cc command, running the command and the output as part of this question.
-Make utility and makefileare widely used in the software development on UNIX systems. Knowing it will be helpful to you. Hence, if you do it with the makefileas explained below, you will get extra points. Make sure you understand the concept of makecommand and the makefile.
Create a file called makefilecontaining the following 4 lines assuming hw3q2.c is the name of the C program filename for this Q2.
hw3q2: hw3q2.o
cc hw3q2.o -o hw3q2
hw3q2.o: hw3q2.c
cc -c hw3q2.c
The statements in the above makefileconsist of a line with (1) target filename to be created like hw3q2.o followed by : (colon character), (2) followed by a list of dependent filename(s) like hw3q2.c which are needed to create the target file, and (3) in the next line the command to create the target file like the cc command. This command line MUST start with tab keyas the first character in the line, otherwise, make command will generate an error resulting in the target file not getting created. Using this description, describe the first two lines and understand them.
-Note, the ultimate target file you want to create must be specified first, in this case hw3q2, and then other target file(s) to be created.
-Now try to understand all the four lines together. You will see what the makefiledoes.
-To perform the statements in the makefile, you have to run the makecommand. The make command will read the statements (lines) specified in the makefileand perform what you have asked to do. The makecommand can be invoked in one of two ways as shown below:
make f makefile
make
-In the first case, you specify the name of the makefile. If you name of the file with a different name like hw3q2make, then you can specify the make command as
make f hw3q2make
-In the second case, when the filename is not specified, the make command reads the file named makefileby default.
IMPORTANT NOTE: The line containing the cc command MUST start with a tab.
Otherwise, make command will not work and fail with an error.
-Create a directory called q2makedir. Put hw3q2.c file, and the makefilein that directory.
-Change working directory to q2makedir.
Issue makecommand. It will compile hw3q2.c program, link it and create the object file called hw3q2.o and an executable file called hw3q2. Fix any error you made in hw3q2.c and makefile.
-Issue ls l to display all the files in the directory.
-Display the content of source code file hw3q2.c, and the makefile.
-Run the executable file which will produce output.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
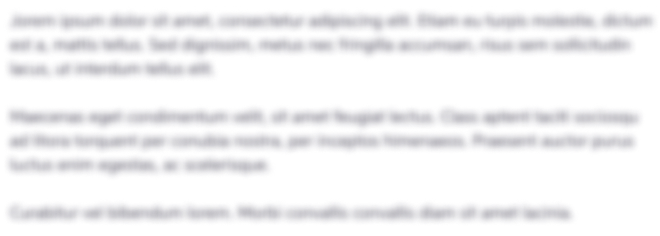
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started