Question
queues.py: class BoundedQueue: # Creates a new empty queue: def __init__(self, capacity): assert isinstance(capacity, int), ('Error: Type error: %s' % (type(capacity))) # throws an assertion
queues.py:
class BoundedQueue: # Creates a new empty queue: def __init__(self, capacity): assert isinstance(capacity, int), ('Error: Type error: %s' % (type(capacity))) # throws an assertion error on not true assert capacity >= 0, ('Error: Illegal capacity: %d' % (capacity)) self.__items = [] # init the list / queue as empty self.__capacity = capacity # Adds a new item to the back of the queue, and returns nothing: def enqueue(self, item): ''' Enqueue the element to the back of the queue :param item: the element to be enqueued :return: No returns ''' ##### START CODE HERE ##### ''' Remember to check the conditions ''' pass ##### END CODE HERE ###### # Removes and returns the front-most item in the queue. # Returns nothing if the queue is empty. def dequeue(self): ''' Dequeue the element from the front of the queue and return it :return: The object that was dequeued '''
##### START CODE HERE ##### ''' 1. remember to check the conditions 2. return the appropriate value '''
pass ##### END CODE HERE ###### # Returns the front-most item in the queue, and DOES NOT change the queue. def peek(self): if len(self.__items)
# Returns a string representation of the queue: def __str__(self): str_exp = "" for item in self.__items: str_exp += (str(item) + " ") return str_exp # Returns a string representation of the object bounded queue: def __repr__(self): return str(self) + " Max=" + str(self.__capacity)
class CircularQueue: # Creates a new empty queue: def __init__(self, capacity): # Check validity of capacity type and value assert isinstance(capacity, int), ('Error: Type error: %s' % (type(capacity))) assert capacity >= 0, ('Error: Illegal capacity: %d' % (capacity)) # Initialize private attributes self.__items = [] self.__capacity = capacity self.__count=0 self.__head=0 self.__tail=0 # Allocate space for the circular queue for i in range(self.__capacity): self.__items.append(None) # Adds a new item to the back of the queue, and returns nothing: def enqueue(self, item): ''' This function enqueues the item into the back of the queue :param item: The item to be queued :return: No returns '''
##### START CODE HERE ##### ''' Remember to check the proper conditions ''' pass ##### END CODE HERE ###### # Removes and returns the front-most item in the queue. # Returns nothing if the queue is empty. def dequeue(self): ''' Dequeue the the element from the front of the queue and return the value :return: Returns the object that is dequeued '''
##### START CODE HERE ##### ''' Remember to check the proper conditions (some hints) 1. get item at the head of queue 2. remove the item from the head of queue 3. decrease stored size of queue 4. Shift the head 5. return the item ''' pass ##### END CODE HERE ###### # Returns the front-most item in the queue, and DOES NOT change the queue. def peek(self): if self.__count == 0: raise Exception('Error: Queue is empty') return self.__items[self.__head] # Returns True if the queue is empty, and False otherwise: def is_empty(self): return self.__count == 0 # Returns True if the queue is full, and False otherwise: def is_full(self): return self.__count == self.__capacity # Returns the number of items in the queue: def size(self): return self.__count # Returns the capacity of the queue: def capacity(self): return self.__capacity # Removes all items from the queue, and sets the size to 0 # clear() should not change the capacity def clear(self): self.__items = [] self.__count = 0 self.__head = 0 self.__tail = 0 # Returns a string representation of the queue: def __str__(self): str_exp = "]" i = self.__head for j in range(self.__count): str_exp += str(self.__items[i]) + " " i = (i+1) % self.__capacity return str_exp + "]" # Returns a string representation of the object CircularQueue def __repr__(self): return str(self.__items) + " H= " + str(self.__head) + " T="+str(self.__tail) + " (" +str(self.__count)+"/"+str(self.__capacity)+")" def main(): # Test bounded queue creation bq=BoundedQueue(3) print("My bounded queue is:", bq) print(repr(bq)) print("Is my bounded queue empty?", bq.isEmpty()) print('----------------------------------') ''' # 1. To Do # Test when we try to dequeue from an EMPTY queue try: # To Do: Write your own test
except Exception as dequeueError: # To Do: Write a way to handle it
print('----------------------------------') ''' ''' # 2. To Do # Test adding one element to queue # Your test code goes here... print(bq) print(str(bq)) print("Is my bounded queue empty?", bq.isEmpty()) print('----------------------------------') ''' ''' # 3. Uncomment and run # Test adding more elements to queue bq.enqueue("eva") bq.enqueue("paul") print(repr(bq)) print("Is my bounded queue full?", bq.isFull()) print("There are", bq.size(), "elements in my bounded queue.") print('----------------------------------') ''' ''' # 4. To Do # Test trying to add an element to a FULL queue # Your test code goes here...hint: look at dequeuing from EMPTY queue print('----------------------------------') ''' ''' # 5. Uncomment and run # Test removing element from full queue item = bq.dequeue() print(repr(bq)) print(item,"was first in the bounded queue:", bq) print("There are", bq.size(), "elements in my bounded queue.") print('----------------------------------') ''' ''' # 6. Uncomment and run # Test capacity of queue print("Total capacity is:", bq.capacity()) '''
''' # 7. To Do: Uncomment print statements, one at a time # Can we just access private capacity attribute directly outside of Class definition? #print(bq.capacity) #print(bq.__capacity) '''
if __name__ == '__main__': main()
Exercise 1: Complete the Bounded and Circular Queue Class Definitions, and Test 1. Download and save queues.py from eClass. This file contains partial implementations for the Bounded Queue and the Circular Queue, as discussed in the lectures. enqueue (item) - Complete this method for both types of queues. You may refer directly to the lecture slides on eClass. dequeue () - Complete this method for both types of queues. You may refer directly to the lecture slides on eClass. 2. Test your Bounded Queue and Circular Queue classes (especially the methods you have just written). To get you started, queues.py contains the skeleton code for a number of tests to check various aspects of the Bounded Queue's implementation. Uncomment and run/write the code for each test, one at a time, to check that you get the same sample output as below. Test 1: Complete the try statement. If the Bounded Queue's dequeue method is correct, an exception should be raised when you attempt to dequeue from the empty queue, bq. Handle it by printing out the argument of the exception. Change the argument string in the Bounded Queue's dequeue definition and rerun this test - is the new message displayed on the screen now? Test 2: Test the Bounded Queue's enqueue method by trying to add 'bob' to bq. When printing the contents of bq to the screen, are print (bq) and print (str (bq)) the same? Does the method is Empty return the expected result? Test 3: Run given code. When multiple items are added to the queue, does the queue store them in the correct order (test the repr function)? Do the is FullO and size methods give the expected results? Test 4: Write a try statement to attempt to add an item to the full bq. If the Bounded Queue's enqueue method is correct, it should raise an exception. Handle that exception in the main by printing out the exception's argument. Test 5: Run given code. Can an item be removed from a full bounded queue? Does the dequeue method return the expected item, and are the contents of bq updated? Test 6: Run given code. Test the capacity0 method. How is capacity different from size? Test 7: Try to access the private capacity attribute outside of the Bounded Queue class definition (i.e. in the main). What happens? Does the same thing happen if you try to access a non-private attribute outside of its class definition? Sample Output: My bounded queue is: Max=3 Is my bounded queue empty? True Try to de queue an empty bounded queue... Error: Queue is empty - - - - - - bob bob Is my bounded queue empty? False -------------- bob eva paul Max=3 Is my bounded queue full? True There are 3 elements in my bounded queue. -- - - - - - - - - - - - - - - Try to enqueue a full bounded queue.. Error: Queue is full eva paul Max=3 bob was first in the bounded queue: eva paul There are 2 elements in my bounded queue. - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - Total capacity is: 3 Exercise 1: Complete the Bounded and Circular Queue Class Definitions, and Test 1. Download and save queues.py from eClass. This file contains partial implementations for the Bounded Queue and the Circular Queue, as discussed in the lectures. enqueue (item) - Complete this method for both types of queues. You may refer directly to the lecture slides on eClass. dequeue () - Complete this method for both types of queues. You may refer directly to the lecture slides on eClass. 2. Test your Bounded Queue and Circular Queue classes (especially the methods you have just written). To get you started, queues.py contains the skeleton code for a number of tests to check various aspects of the Bounded Queue's implementation. Uncomment and run/write the code for each test, one at a time, to check that you get the same sample output as below. Test 1: Complete the try statement. If the Bounded Queue's dequeue method is correct, an exception should be raised when you attempt to dequeue from the empty queue, bq. Handle it by printing out the argument of the exception. Change the argument string in the Bounded Queue's dequeue definition and rerun this test - is the new message displayed on the screen now? Test 2: Test the Bounded Queue's enqueue method by trying to add 'bob' to bq. When printing the contents of bq to the screen, are print (bq) and print (str (bq)) the same? Does the method is Empty return the expected result? Test 3: Run given code. When multiple items are added to the queue, does the queue store them in the correct order (test the repr function)? Do the is FullO and size methods give the expected results? Test 4: Write a try statement to attempt to add an item to the full bq. If the Bounded Queue's enqueue method is correct, it should raise an exception. Handle that exception in the main by printing out the exception's argument. Test 5: Run given code. Can an item be removed from a full bounded queue? Does the dequeue method return the expected item, and are the contents of bq updated? Test 6: Run given code. Test the capacity0 method. How is capacity different from size? Test 7: Try to access the private capacity attribute outside of the Bounded Queue class definition (i.e. in the main). What happens? Does the same thing happen if you try to access a non-private attribute outside of its class definition? Sample Output: My bounded queue is: Max=3 Is my bounded queue empty? True Try to de queue an empty bounded queue... Error: Queue is empty - - - - - - bob bob Is my bounded queue empty? False -------------- bob eva paul Max=3 Is my bounded queue full? True There are 3 elements in my bounded queue. -- - - - - - - - - - - - - - - Try to enqueue a full bounded queue.. Error: Queue is full eva paul Max=3 bob was first in the bounded queue: eva paul There are 2 elements in my bounded queue. - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - - Total capacity is: 3
Step by Step Solution
There are 3 Steps involved in it
Step: 1
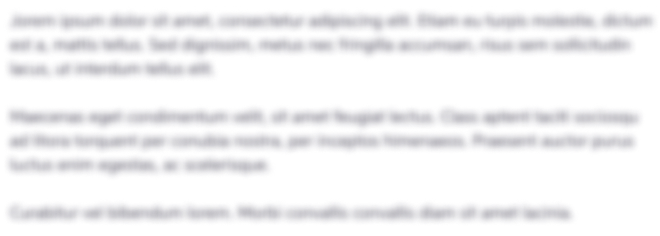
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started