Question
Random Number Generation In the first three programs, the user has been asked for input. This program will be different. Rather than asking the user
Random Number Generation In the first three programs, the user has been asked for input. This program will be different. Rather than asking the user how many values are in a set of numbers or even what the values are, a random number generator will be used to determine the size of a set and the actual values in the set. To use the random number generator, first add a #include statement for the cstdlib library to the top of the program: #include
Basic Program Logic Initialize the random number generator using a seed value of 30. Note: other seed values may be used to produce different results. However, the version that is handed in for grading MUST use a seed value of 30. Generate a random number between 1 and 500. This will be the number of values in the first set. Display the number of values in the set with an appropriate label. In a for loop that executes exactly "number of values in the first set" number of times, generate a random number (no restrictions), and display the random number. Make sure that there are only 5 values displayed per line. Generate a random number between 1 and 300. This will be the number of values in the second set. Display the number of values in the set with an appropriate label. In a while loop that executes exactly "number of values in the second set" number of times, generate a random number (no restrictions), and display the random number. Make sure that there are only 7 values displayed per line. Generate a random number between 1 and 100. This will be the number of values in the third set. Display the number of values in the set with an appropriate label. In a do while loop that executes exactly "number of values in the third set" number of times, generate a random number (no restrictions), and display the random number. Make sure that there are only 8 values displayed per line. Symbolic Constants This program MUST use at least 6 symbolic constants. Three of the constants should be for the maximum values for the sizes of the sets of numbers. The values should be 100, 300, and 500. The other three constants should be for the maximum number of values to be displayed for each set of numbers. The values should be 5, 7, and 8. More symbolic constants may be added to the code if necessary. Output Run 1 (using srand(30);) on Windows PC The for loop will generate 137 random values. 2491 107 3440 23380 31295 3435 10003 14278 10010 1416 29422 9949 5151 30942 11775 27052 21986 25150 32292 2993 4799 13348 6968 30563 5406 12777 4991 14602 21238 26938 12100 25555 3728 24521 14272 19748 29838 18754 9534 4653 23339 22913 30845 7104 1732 1794 17238 5218 6040 20889 9374 1186 1228 24931 15182 2473 18610 4604 21729 23059 8778 15858 23294 3325 4105 24186 17495 22367 26586 21346 3281 13213 12387 26439 30949 10072 8073 25608 10310 7050 25142 19322 15300 31442 4441 2142 4720 2572 30767 11152 25089 12069 4034 2433 11894 6701 21423 19430 25853 1198 21547 19378 7700 31549 10568 18242 19182 25934 11125 18648 21016 16205 5444 20811 24412 15082 29297 6909 27366 14772 31 2316 15269 21993 25188 5837 26868 28044 21256 23414 8058 25529 11658 30234 11598 3126 31844
The while loop will generate 172 random values. 6495 30026 27536 4699 18964 978 17483 18547 32559 5521 22041 12072 8951 7624 28937 6362 13974 28678 21818 21695 24000 11586 15262 9360 19861 1831 23550 14978 8905 19887 5183 21368 25346 31475 5347 16759 11649 12610 14035 31322 24325 24432 24155 32455 11500 32624 3958 11617 31644 8997 5964 22079 29729 17270 28409 8862 7447 1441 15248 9426 31435 27345 3935 9347 31389 7666 20597 31509 11689 21444 26722 5224 5798 28974 8510 26562 3853 27606 21485 32009 1918 6382 19360 21046 10081 24145 671 13574 4041 31182 7239 25842 17821 21187 16462 4450 1785 2542 15183 2078 4050 15233 2178 10245 4569 10100 10442 27649 25506 13343 27382 8762 11014 32520 2368 9098 1734 28666 16410 7986 31600 599 16939 20171 10119 14510 11668 9855 734 28304 26231 21880 11589 31904 31418 10905 4199 25322 29448 12652 22771 28022 13593 7004 2810 1820 23348 18725 13721 11929 9458 2184 2519 16867 25924 23107 22736 5050 4535 21568 15636 32640 22263 29709 29514 28490 22162 30092 9753 2103 29183 492
The do while loop will generate 17 random values. 28929 18143 19159 8227 26321 16514 26093 27425 5208 25261 31858 14533 24347 12699 9027 9666 7950
Step by Step Solution
There are 3 Steps involved in it
Step: 1
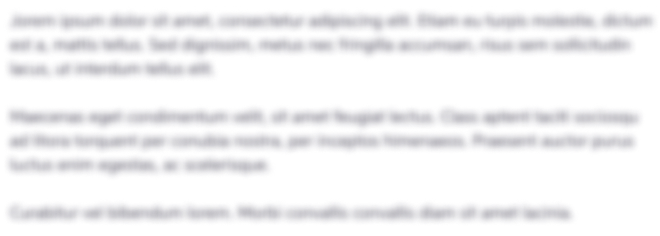
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started