Question
Read and understand the code provided to you here . Modify the code to compute the forward differences, and compare the errors obtained with the
Read and understand the code provided to you here . Modify the code to compute the forward differences, and compare the errors obtained with the central differences. a. Implement the forward differences and modify the code to compute: i. Dc derivative in central differences ii. Df derivative in central differences iii. Ec Truncation Error in central differences iv. Ef Truncation Error in forward differences b. What do you observe in the log-log plot? c. Can you assess the order of magnitude of the truncation error just looking at the graph?
import numpy as np
import matplotlib.pyplot as plt
def diffex(func, dfunc, x, n):
dftrue = dfunc(x)
h = 1
H = np.zeros(n)
D = np.zeros(n)
E = np.zeros(n)
H[0] = h
D[0] = (func(x+h) - func(x-h))/2/h
E[0] = abs(dftrue - D[0])
for i in range(1,n):
h = h/10
H[i] = h
D[i] = (func(x+h) - func(x-h))/2/h
E[i] = abs(dftrue - D[i])
return H, D, E
ff = lambda x : -0.1 * x ** 4 - 0.15 * x ** 3 - 0.5 * x **2 - 0.25 * x + 1.2
df = lambda x : - 0.4 * x ** 3 - 0.45 * x **2 - x - 0.25
# Example 4.5 --> n = 11, but can change
n = 11
H, D, E = diffex(ff, df, 0.5, n)
print(' step size finite differences true error')
for i in range(n):
print('{0:14.10f} {1:16.14f} {2:16.13f}'.format(H[i], D[i], E[i]))
plt.loglog(H, E, "k",label = "Total Error Central")
plt.grid()
plt.title("Plot of the Error(E) versus Step Size(H))")
plt.xlabel("step size")
plt.ylabel("Error")
plt.legend()
plt.show()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
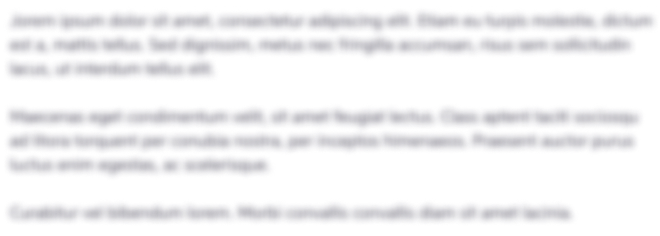
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started