Answered step by step
Verified Expert Solution
Question
1 Approved Answer
// READ BEFORE YOU START: // Please read the given Word document for the project description with an illustrartive diagram. // You are given a
// READ BEFORE YOU START: // Please read the given Word document for the project description with an illustrartive diagram. // You are given a partially completed program that creates a list of students for a school. // Each student has the corresponding information: name, standard, and a linked list of absents. // Please read the instructions above each required function and follow the directions carefully. // If you modify any of the given code, return types, or parameters, you risk failing test cases. // // Note, Textbook Section 2.10 gives a case study on complex linked list operations. // This project is based on that case study. Make sure you read the code in section 2.10. // The following will be accepted as input in the following format: "name:standard" // Example Input: "Tom:2nd" or "Daisy:3rd" // Valid name: String containing alphabetical letters beginning with a capital letter // Valid standard: String containing alphabetical letters beginning with a number eg: 1st 2nd // Valid date: String in the following format: "MM/DD/YYYY" ex: "01/01/2010" // All input will be a valid length and no more than the allowed number of dogs will be added to the linked list. #include#include #include #include // included to check for memory leaks #define CRTDBG_MAP_ALLOC #include #pragma warning(disable: 4996) // used to create a linked list of containers, each contaning a "student" struct container { struct student *student; struct container *next; } *list = NULL; // used to hold student information and linked list of "absents" struct student { char name[30]; char standard[30]; struct absent *absents; }; // used to create a linked list of absents containing "dates" struct absent { char date[30]; struct absent *next; }; // forward declaration of functions that have already been implemented void flush(); void branching(char); void registration(char); void remove_all(struct container*); void display(struct container*); // the following forward declarations are for functions that require implementation // return type // name and parameters // points void add_student(char*, char*); // 5 struct student* search_student(char*); // 5 void add_absent(char*, char*); // 10 char* last_absent(char*); // 15 void remove_one(char*); // 15 // Total: 50 points for hw07 struct container* list_of_standard(char*); // 25 struct container* list_by_name(); // 25 // Total: 50 points for hw08 int main() { char ch = 'i'; printf("Student Information "); do { printf("Please enter your selection: "); printf("\ta: add a new student to the list "); printf("\ts: search for a student on the list "); printf("\tr: remove a student from the list "); printf("\tc: add an absence date for a student "); printf("\tl: display last absence date for a student "); printf("\tn: display list of students by name "); printf("\tb: display list of students of a given standard "); printf("\tq: quit "); ch = tolower(getchar()); flush(); branching(ch); } while (ch != 'q'); remove_all(list); list = NULL; _CrtDumpMemoryLeaks(); // check for memory leaks (VS will let you know in output if they exist) return 0; } // consume leftover ' ' characters void flush() { int c; do c = getchar(); while (c != ' ' && c != EOF); } // branch to different tasks void branching(char c) { switch (c) { case 'a': case 's': case 'r': case 'c': case 'l': case 'b': case 'n': registration(c); break; case 'q': break; default: printf("Invalid input! "); } } // This function will determine what info is needed and which function to send that info to. // It uses values that are returned from some functions to produce the correct ouput. // There is no implementation needed here, but you should trace the code and know how it works. // It is always helpful to understand how the code works before implementing new features. // Do not change anything in this function or you risk failing the automated test cases. void registration(char c) { if (c == 'a') { char input[100]; printf(" Please enter the student's info in the following format: "); printf("name:standard "); fgets(input, sizeof(input), stdin); // discard ' ' chars attached to input input[strlen(input) - 1] = '\0'; char* name = strtok(input, ":"); // strtok used to parse string char* standard = strtok(NULL, ":"); struct student* result = search_student(name); if (result == NULL) { add_student(name, standard); printf(" Student added to list successfully "); } else printf(" That student is already on the list "); } else if (c == 's' || c == 'r' || c == 'c' || c == 'l') { char name[30]; printf(" Please enter the student's name: "); fgets(name, sizeof(name), stdin); // discard ' ' chars attached to input name[strlen(name) - 1] = '\0'; struct student* result = search_student(name); if (result == NULL) printf(" That student is not on the list "); else if (c == 's') printf(" Standard: %s ", result->standard); else if (c == 'r') { remove_one(name); printf(" Student removed from the list "); } else if (c == 'c') { char date[30]; printf(" Please enter the date of absence: "); fgets(date, sizeof(date), stdin); // discard ' ' chars attached to input date[strlen(date) - 1] = '\0'; add_absent(name, date); printf(" Absent date added "); } else if (c == 'l') { char* result = last_absent(name); if (result == NULL) printf(" No absence documented. "); else printf(" Last absent on: %s ", result); } } else if (c == 'b') { char standard[30]; printf(" Please enter the standard: "); fgets(standard, sizeof(standard), stdin); // discard ' ' chars attached to input standard[strlen(standard) - 1] = '\0'; struct container* result = list_of_standard(standard); printf(" List of students with standard %s: ", standard); display(result); remove_all(result); result = NULL; } else // c = 'n' { struct container* result = list_by_name(); printf(" List of students sorted by name: "); display(result); remove_all(result); result = NULL; } } // This function recursively removes all students from the linked list of containers // Notice that all of the absents for all of the students must be removed as well void remove_all(struct container* students) { struct absent* temp; if (students != NULL) { remove_all(students->next); while (students->student->absents != NULL) { temp = students->student->absents; students->student->absents = students->student->absents->next; free(temp); } free(students->student); free(students); } } // This function prints the list of students in an organized format // It may be useful to trace this code before you get started void display(struct container* students) { struct container* container_traverser = students; if (container_traverser == NULL) { printf(" There are no students on this list! "); return; } while (container_traverser != NULL) // traverse list of students { printf("Name: %s ", container_traverser->student->name); printf("Standard: %s ", container_traverser->student->standard); printf("Absence on file: "); struct absent* ptr = container_traverser->student->absents; if (ptr == NULL) { printf("No absence documented."); } else { while (ptr != NULL) // traverse list of absents { printf(" %s", ptr->date); ptr = ptr->next; } } printf(" "); // formatting container_traverser = container_traverser->next; } } // hw07 Q1 : add (5 points) // This function should add student to the head of the list of containers. // The function search_student() is called before calling this function, // therefore you can assume that the student is not already on the list. void add_student(char* name, char* standard) { } // hw07 Q2 : search (5 points) // In this function, you are passed the name of a student to find his/her standard. // If the student exists on the list, return a pointer to the requested student. If not, return NULL. // (You must return a pointer to a node in your list. Do not create a pointer that just includes the standard) // (Remember that it is enough to search for a student by only their name since no 2 students will have the same name) struct student* search_student(char* name) { return NULL; } // hw07 Q3: add_absent (10) // In this function, you are passed the name of a student and a date of absence. // You should add the date to the tail of the linked list of the students "absents". // You can assume that all absents will be added in chronological order. // The function search_student() is called before calling this function, // therefore you can assume that the student is already on the student list and // the new absence date is not on the absents list. void add_absent(char* name, char* date) { } // hw07 Q4: last_absent (15) // In this function, you are passed the name of a student to find the date of its last absent. // Remember that absents are stored in chronological order, // therefore the last checkup will be at the tail of the linked list of absents. // If the student has not yet had an anbsent added to its list of absents, return NULL. // The function search_student() is called before calling this function, // therefore you can assume that the student is not already on the list. char* last_absent(char* name) { } // hw07 Q5: remove_one (15) // In this function, you are passed the name of a dog to remove the corresponding dog from the list. // The search function is called before this function so you can assume that the dog is on the list. // You will need to find the dog and remove it using proper memory management to ensure no memory leaks. void remove_one(char* name) { } /// hw08 Q1: list_of_standard (25) // This function is used to construct a new linked list of containers from the global list of containers. // The returned list should only contain students which are of the standard type parameter (container->student->standard). // No sorting is required for this list. // The list that you return will be cleaned up for you by the remove_all() function (see registration() function), // however you will need to make sure that you leave no dangling references (those cause memory leaks too). // Notice that the returned list will need to contain all student and checkup information to be displayed. // This function should NOT modify the global linked list. struct container* list_of_standard(char* standard) { return NULL; } // hw08 Q2: list_by_name (25) // This function is used to construct a new linked list of containers from the global list of containers. // The returned list should be sorted alphabetically by each container's student's name (container->student->name). // The list that you return will be cleaned up for you by the remove_all() function (see registration() function), // however you will need to make sure that you leave no dangling references (those cause memory leaks too). // Notice that the returned list will need to contain all student and absence information to be displayed. // You can again assume that for this assignment, no 2 students on the list will have the same name. // You may want to use the function that you have written above as a blueprint for this function. // This function should NOT modify the global linked list. struct container* list_by_name() { return NULL; } ![]()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
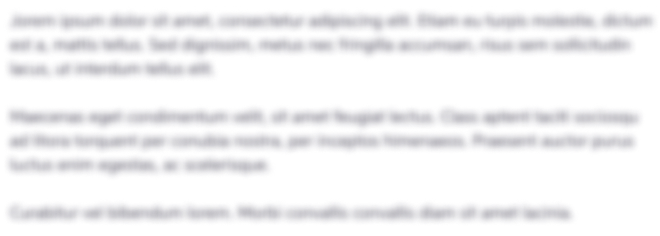
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started