Question
READ CAREFULLY AND FOLLOW THE EXAMPLES ALONG WITH THE CODE PREVIDED EXACTLY Write a Python program to simulate an ecosystem containing two types of animals,
READ CAREFULLY AND FOLLOW THE EXAMPLES ALONG WITH THE CODE PREVIDED EXACTLY
Write a Python program to simulate an ecosystem containing two types of animals, bears and fish.
The ecosystem consists of a river, which is modeled as a relatively large list.
Each element of the list should be a Bear object, a Fish object, or None.
In each time step of the simulation, in order, process each animal decision.
Based on a random process, each animal either attempts to move (e.g., option 0) into an adjacent list location or stay where it is (e.g., option 1).
If two animals of the same type are about to collide in the same cell, then they stay where they are, but they create a new instance of that type of animal, which is placed in a random empty (i.e., previously None) location in the list (i.e., river).
If a bear and a fish collide, however, then the fish dies (i.e., it gets removed from the list) and the bear does not move. If the bear, while moving, collides with a fish, the bear eats the fish at the fish location.
If the animal is at the end of the river and it decides to move, then the animal gets out of the river.
You need to create a parent class Animal, and subclasses for the Bear and Fish.
Initial class skeletons are provided to you. You need to use those skeletons to complete the missing code.
Similarly, you need to work on a class for the Ecosystem that will simulate this scenario. The skeleton for the class is provided to you. You need to complete the missing code.
Every animal has a location and a name that are initialized in the constructor.
Similarly, every animal has at least the following methods:
_move(): to set the new location for the animal
_get_location(): to return its location
__str__() : method to print the type of animal and its current location
get_class_name(): to return the class name
speak(): Allows the animal to speak
You can have other helper methods for this class (although not necessary).
The data and methods are inherited by all animals.
The animal subclasses, Bear and Fish, only assign the animal type (i.e., name) to each new animal.
Animals can speak. However, only the bears can say a different thing. See the examples below.
You need a class representing the Ecosystem.
This class will need to have a constructor to initialize the Ecosystem.
The constructor needs to initialize an empty river and randomly place animals into the river. The size of the initial river is a parameter for the creation of the object.
To initialize this random ecosystem, the constructor calls the method initialize_random_ecosystem().
In this method you need to:
1) initialize a random number of Bears and place a Bear object in a random empty position in the river. You can generate between 1 to 4 bears.
2) initialize a random number of Fish and place a Fish object in a random empty position in the river. You can generate between 1 to 4 Fish.
Use a river size that is larger than the probable number of animals. For example, you could have 4 Bears and 4 Fish, therefore your river size should be larger than 8 positions.
For the simulation, you need to complete the simulate() method.
This method receives a parameter for the number of iterations for traversing the river. This is a parameter passed by the user.
This method is the responsible of simulating the scenario described above for the animals behaviour.
Similarly, this method calls a method animal_creation(), if that is required by the animal scenario. This method receives an animal as parameter, and it creates a new animal of the same type as the received animal. Also, this method needs to assign the new animal randomly in a free space in the river.
Finally, the Ecosystem must have a method show() to show all the information (I.e., name and position) for each animal in the river.
////animal.py
def __init__(self):
"""
Constructor for the animal.
Every animal has a location and name
"""
pass
def __str__(self):
"""Method used to print the name o the animal and its location
"""
pass
def _move(self, new_location):
""" Method to set the new location
"""
pass
def _get_location(self):
""" Method to return the location
"""
pass
def get_class_name(self):
""" Method to return the class name
"""
pass
def speak(self):
"""Method that allows the animal to speak
"""
print("\tIt says: *Silence...*")
//fish.py
from Animal import Animal
class Fish(Animal):
def __init__(self) -> None:
"""Constructor for the Fish
This constructor initializes the features of the parent class
Assigns "Fish" as the name of the animal
"""
pass
//bear.py
from Animal import Animal
class Bear(Animal):
def __init__(self):
"""Constructor for the Bear
This constructor initializes the features of the parent class
Assigns "Bear" as the name of the animal
"""
pass
def speak(self):
print("\tIt says: I am eating!")
//simulation.py
import random
from Animal import Animal
from Bear import Bear
from Fish import Fish
random.seed(42) # Change this number for your different tests.
class Ecosystem():
def __init__(self, size):
""" Constructor for the Ecosystem
It initializes the river (a list with a given size)
It calls the method to initialize the river with animals in random places
"""
# Remove pass and write your code here
pass
self.initialize_random_ecosystem()
def initialize_random_ecosystem(self):
""" Method to randomly initialize the ecosystem
Randomly picks a number between 1 and 4 to decide the number of Bears
Randomly places the Bears in the river in empty positions
Randomly picks a number between 1 and 4 to decide the number of Fish
Randomly places the Fish in the river in empty positions
"""
# Remove pass and write your code here
pass
def animal_creation(self, type_of_animal):
"""Method to create a new type of animal when two animals of the same type collides
The new created animal is placed randomly in the river in any empty position
"""
# Remove pass and write your code here
pass
def show(self):
"""Method to show each position in the river and the animal each position contains
"""
# Remove pass and write your code here
pass
def simulate(self, iterations):
"""Method to simulate the Ecosystem movement
It similates the ecosystem live depending on the number of iterations passe as argument
In each iteration, it processes in order each animal
It randomly picks an action (0=move, 1=stay)
It moves the animal and do all the similation as defined in the scenario.
It checks if the next position is empty to move the animal
If the position is not empty, then it checks what type of animal is in the next position
It decides what action to perform based on the type of animal in the next position
"""
print("~~~ Initializing the Simulation ~~~")
# Write your code here
def create_new_animal(self, a):
"""Optional Method
You can optionally use this method to get the animal's class name
before you use the method animal_creation()
"""
# Remove pass and Write your code here
pass
//ecosystem_tester.py
if __name__ == "__main__":
print(" Initializing Ecosystem")
e = Ecosystem(10)
print(" Ecosystem BEFORE simulation")
e.show()
print(" TESTING")
e.simulate(1)
print(" Ecosystem AFTER simulation")
e.show()
Output must match syntax of below simulations
Example 1 This example shows the simulation when a Bear eats a Fish Initializing Ecosystem Initial number of bears: 3 Initial number of fish: 1 Bear assigned to position 4 Bear assigned to position 0 Bear assigned to position 9 Fish assigned to position 7 Ecosystem BEFORE simulation Bear at location 0 None None None Bear at location 4 None None Fish at location 7 None Bear at location 9 ~~~ Initializing the Simulation ~~~ ~~~ Iteration 1 ~~~~ Processing Animal at location 0 Animal chose to 1 Stay Processing Animal at location 4 Animal chose to 0 Location 5 is empty, so move it Processing Animal at location 5 Animal chose to 0 Location 6 is empty, so move it Processing Animal at location 6 Animal chose to 0 Move Bear at location 6 to 7 Bear eats Fish It says: I am eating! Processing Animal at location 7 Animal chose to 1 Stay Processing Animal at location 9 Animal chose to 1 Stay Ecosystem AFTER simulation Bear at location 0 None None None None None None Bear at location 7 None Bear at location 9
Example 2. This example shows the simulation for two iterations. The river size is 10. Initializing Ecosystem Initial number of bears: 1 Initial number of fish: 4 Bear assigned to position 5 Fish assigned to position 6 Fish assigned to position 0 Fish assigned to position 4 Fish assigned to position 1 Ecosystem BEFORE simulation Fish at location 0 Fish at location 1 None Output from show() Output from simulate() None Fish at location 4 Bear at location 5 Fish at location 6 None None None ~~~ Initializing the Simulation ~~~ ~~~ Iteration 1 ~~~~ Processing Animal at location 0 Animal chose to 0 Stay Fish at location 0 BUT Creating a new Fish New Fish assigned to position 2 Processing Animal at location 1 Animal chose to 1 Stay Processing Animal at location 2 Animal chose to 1 Stay Processing Animal at location 4 Animal chose to 1 Stay Processing Animal at location 5 Animal chose to 0 Move Bear at location 5 to 6 Bear eats Fish It says: I am eating! Processing Animal at location 6 Animal chose to 1 Stay ~~~ Iteration 2 ~~~~ Processing Animal at location 0 Animal chose to 1 Stay Processing Animal at location 1 Animal chose to 0 Stay Fish at location 1 BUT Creating a new Fish New Fish assigned to position 8 Processing Animal at location 2 Animal chose to 1 Stay Processing Animal at location 4 Animal chose to 1 Stay Processing Animal at location 6 Animal chose to 0 Location 7 is empty, so move it Processing Animal at location 7 Animal chose to 0 Move Bear at location 7 to 8 Bear eats Fish It says: I am eating! Processing Animal at location 8 Animal chose to 0 Location 9 is empty, so move it Processing Animal at location 9 Animal chose to 1 Stay Ecosystem AFTER simulation Fish at location 0 Fish at location 1 Fish at location 2 None Fish at location 4 None None None None Bear at location 9
Example 3. This example shows the simulation for one iteration. The river size is 10. Initializing Ecosystem Initial number of bears: 1 Initial number of fish: 3 Bear assigned to position 8 Fish assigned to position 5 Fish assigned to position 1 Fish assigned to position 7 Ecosystem BEFORE simulation None Fish at location 1 None None None Fish at location 5 None Fish at location 7 Bear at location 8 None Output from show() Output from initialize_random_ecosystem() ~~~ Initializing the Simulation ~~~ ~~~ Iteration 1 ~~~~ Processing Animal at location 1 Animal chose to 1 Stay Processing Animal at location 5 Animal chose to 1 Stay Processing Animal at location 7 Animal chose to 0 Move Fish at location 7 to 8 Processing Animal at location 8 Animal chose to 1 Stay Ecosystem AFTER simulation None Fish at location 1 None None None Fish at location 5 None None Bear
Step by Step Solution
There are 3 Steps involved in it
Step: 1
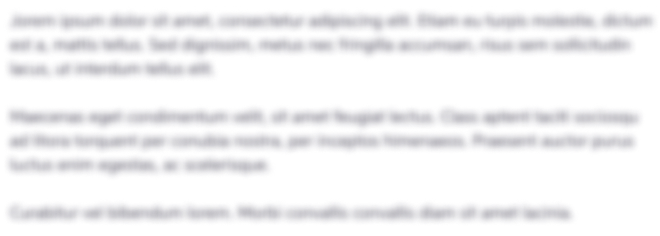
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started