Question
Read files SalaryLevel.java, Employee.java, and Manager.java in the assignment project, then add the missing code so that 1) the tests in EmployeeTest.java and ManagerTest.java will
Read files SalaryLevel.java, Employee.java, and Manager.java in the assignment project, then add the missing code so that 1) the tests in EmployeeTest.java and ManagerTest.java will all pass and 2) the classes meet all the requirements specified in the Java files.
What to Do: [Task 1] Add necessary code in SalaryLevel.java, Employee.java, and Manager.java
SalaryLevel.java
package hk.edu.polyu.comp.comp2021.assignment3.employee; /** * Levels of salary. */ enum SalaryLevel { ENTRY(1), JUNIOR(1.25), SENIOR(1.5), EXECUTIVE(2); // Add missing code here. }
Employee.java
package hk.edu.polyu.comp.comp2021.assignment3.employee; /** * An employee in a company. */ public class Employee{ /** * Name of the employee. */ private final String name; /** * Level of salary of the employee. */ private SalaryLevel salaryLevel; /** * Return the name of the employee. */ public String getName(){ return name; } /** * Return the salary level of the employee. */ public SalaryLevel getSalaryLevel(){ return salaryLevel; } /** * Set the salary level. */ public void setSalaryLevel(SalaryLevel salaryLevel){ this.salaryLevel = salaryLevel; } /** * Initialize an employee object. */ public Employee(String name, SalaryLevel level){ // Add missing code here. } /** * Return the salary of the employee. */ public double salary(){ // The salary of an employee is computed as the multiplication // of the base salary (2000.0) and the scale of the employee's salary level. // Add missing code here. } /** * Base salary of all employees. */ public static final double BASE_SALARY = 2000.0; }
Manager.java
package hk.edu.polyu.comp.comp2021.assignment3.employee; /** * A manager in a company. */ public class Manager extends Employee{ private double bonusRate; /** * Initialize a manager object. */ public Manager(String name, SalaryLevel level, double bonusRate){ // Add missing code here. } public double getBonusRate(){ return bonusRate; } public void setBonusRate(double bonusRate){ this.bonusRate = bonusRate; } // Override method Employee.salary to calculate the salary of a manager. // The salary of a manager is computed as the multiplication // of his/her regular salary as an employee and his/her bonusRate plus 1. public double salary(){ // Add missing code here. } }
Employee.testcode
package hk.edu.polyu.comp.comp2021.assignment3.employee; import org.junit.Test; import static org.junit.Assert.*; public class EmployeeTest { public static final double DELTA = 1E-6; @Test public void testSalaryLevel01() { assertEquals(SalaryLevel.ENTRY.getScale(), 1, DELTA); assertEquals(SalaryLevel.JUNIOR.getScale(), 1.25, DELTA); assertEquals(SalaryLevel.SENIOR.getScale(), 1.5, DELTA); assertEquals(SalaryLevel.EXECUTIVE.getScale(), 2, DELTA); } @Test public void testEmployee02(){ Employee employee1 = new Employee("A", SalaryLevel.ENTRY); assertEquals(employee1.salary(), 2000, DELTA); Employee employee2 = new Employee("B", SalaryLevel.JUNIOR); assertEquals(employee2.salary(), 2500, DELTA); } }
Manager.test code
package hk.edu.polyu.comp.comp2021.assignment3.employee; import org.junit.Test; import static org.junit.Assert.*; public class ManagerTest { @Test public void testManager01(){ Manager manager1 = new Manager("A", SalaryLevel.EXECUTIVE, 0.5); assertEquals(manager1.salary(), 6000, EmployeeTest.DELTA); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
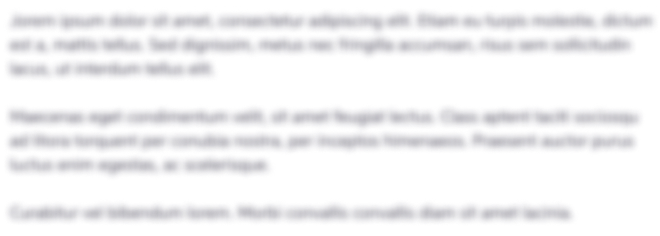
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started