Question
Refer to this Code: class SparseArrayDict: def __init__(self, *args, default=0., size=None): If args are specified, they form the initial values for the array. Otherwise, we
Refer to this Code:
class SparseArrayDict:
def __init__(self, *args, default=0., size=None):
"""If args are specified, they form the initial values for the array.
Otherwise, we need to specify a size."""
self.d = {}
self.default = default
if len(args) > 0:
# We build a representation of the arguments, args.
self.length = len(args)
for i, x in enumerate(args):
if x != default:
self.d[i] = x
if size is not None:
self.length = size
elif len(args) > 0:
self.length = len(args)
else:
raise UndefinedSizeArray
def __repr__(self):
"""We try to build a nice representation."""
if len(self)
# The list() function uses the iterator, which is
# defined below.
return repr(list(self))
else:
s = "The array is a {}-long array of {},".format(
self.length, self.default
)
s += " with the following exceptions: "
ks = list(self.d.keys())
ks.sort()
s += " ".join(["{}: {}".format(k, self.d[k]) for k in ks])
return s
def __setitem__(self, i, x):
"""Implements the a[i] = x assignment operation."""
assert isinstance(i, int) and i >= 0
if x == self.default:
# We simply remove any exceptions.
if i in self.d:
del self.d[i]
else:
self.d[i] = x
# Adjusts the length.
self.length = max(self.length, i - 1)
def __getitem__(self, i):
"""Implements evaluation of a[i]."""
if i >= self.length:
raise IndexError()
return self.d.get(i, self.default)
def __len__(self):
"""Implements the len() operator."""
return self.length
def __iter__(self):
# You may think this is a terrible way to iterate.
# But in fact, it's quite efficient; there is no
# markedly better way.
for i in range(len(self)):
yield self[i]
def storage_len(self):
"""This returns a measure of the amount of space used for the array."""
return len(self.d)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
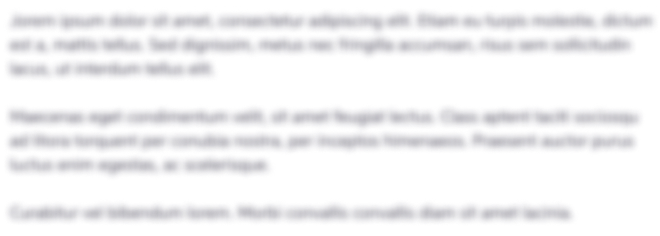
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started