Question
* Removes all 0 elements from an ArrayList */ import java.util.ArrayList; public class RemoveZeroes { public static void main(String[] args) { ArrayList a = new
* Removes all 0 elements from an ArrayList
*/
import java.util.ArrayList;
public class RemoveZeroes
{
public static void main(String[] args)
{
ArrayList a = new ArrayList();
// Add some integers to the array list
a.add(14); a.add(0); a.add(19); a.add(3);
a.add(15); a.add(0); a.add(18); a.add(0);
a.add(44); a.add(0); a.add(51); a.add(78);
// You can also create an Integer wrapper explicitly and add it to the array list
a.add(new Integer(83));
// Print the array list - Note the use of the size() method and the get() method
System.out.println("Before removing the 0 elements:");
for (int i = 0; i < a.size(); i++)
{
System.out.print(a.get(i) + " ");
}
System.out.println();
// Remove the 0 elements
ArrayList aNoZeros = removeZeros(a);
// Print ArrayList a again to see new elements.
System.out.println("After removing the 0 elements:");
for (int i = 0; i < aNoZeros.size(); i++)
{
System.out.print(aNoZeros.get(i) + " ");
}
System.out.println();
System.out.println("Expected:14 19 3 15 18 44 51 78 83");
}
public static ArrayList removeZeros(ArrayList p)
{
// The best way: Create a new empty integer array list and
// only copy the non-zero numbers of parameter array list p into it.
// Use a for loop
//-----------Start below here. To do: approximate lines of code = 5
//
// Return the reference to the newly created array list
//-----------------End here. Reminder: no changes outside the todo regions.
}
}
P.S: Please include screenshots too for formatting.
Step by Step Solution
3.53 Rating (153 Votes )
There are 3 Steps involved in it
Step: 1
Removes all 0 elements from an ArrayList import javautilArrayList public class RemoveZeroes public s...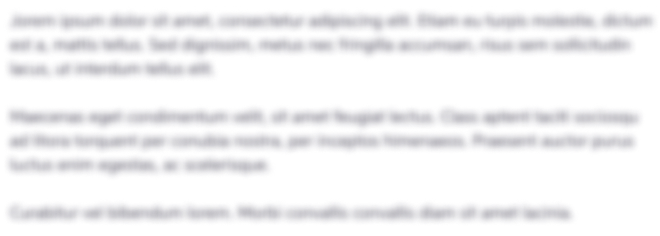
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started