Question
Represent polygons using ordered arrays of points. Each point is itself an array of doubles, where the doubles represent the coordinates of the point. A
Represent polygons using ordered arrays of points. Each point is itself an array of
doubles, where the doubles represent the coordinates of the point. A point may have varying
numbers of coordinates, depending on how many dimensions the polygon is in. For example, a
2D polygon has points with 2 coordinates (x, y), a 3D polygon has points with 3 coordinates (x, y,
z) and an n-dimensional polygon has points with n coordinates (x0, x1, x2, ... , xn).
Below is a triangle, which we can consider 2-dimensional polygon consisting of 3 points.
For this 2D polygon, we have 3 points, {A, B, C} = { {2, 1}, {7, 1}, {7, 4} }.
The bounding box for this polygon can be represented as { {2, 1}, {7, 4} }.
The total length of the lines connecting the points, which we will refer to as the length of the
polygon, is ~13.8 units.
Make the following changes to the file Asso1.java and Polygon.java which are given below:
a. (1 Mark) we need to Complete the constructor and setter for the Polygon class. These methods should
both accept an array of points and save it to the corresponding field on the Polygon class.
b. (3 Marks) Write the code for the calculateDistance() method. This method will accept two
points as input, with each point being represented by a 1-dimensional array of doubles. You
can calculate the distance between two points using Euclidean Distance.
c. (3 Marks) Complete the calculateLength() method. This method will determine the total
length of the polygon if we were to connect each point to the next point in order. Note that
our polygon is considered to be closed. This means that the last point should connect back
to the first point.
d. (3 Marks) Write the calculateBoundingBox() method. This method should return an array of
points that can be used to represent the maximum extents of the polygon. A bounding box
for a shape can be determined using only two points. The first point will represent the
minimum coordinates for the polygon in each dimension, while the second point represents
the maximums.
e. (3 Marks) Write the code for the append() method. The append method of a Polygon should
accept another Polygon as input. The method will then combine the Polygons together by
adding all of the points from the input polygon to the array of the second polygon.
f. (3 Marks) Complete the checkDimensions() method. This method will accept an array of
points as input. The method will then check that there is at least one point in the array, and
that all of the points in the array exist in the same number of dimensions. (Eg: one array of
points should not have points that are 2D as well as points that are 3D) Return true if the
array passes the check.
g. (2 Marks) Finally, we are going to add some validation to our class. If a validation ever fails,
an IllegalArgumentException should be thrown. Here are our validation rules:
a. Anywhere that the points field on the Polygon class may be changed, we must check
that all of the points in the array have the same number of dimensions.
b. If we are to append two polygons together, we must ensure the two polygons are in
the same number of dimensions.
c. To calculate the distance between two points, the points must have the same
number of dimensions.
Asso1.java :
import java.util.Arrays;
/**
* This is a driver class for Assignment 01
* It will instantiate and run some operations on Polygon objects
*/
public class Ass01
{
public static void main(String[] args){
// A 2 dimensional square
System.out.println("Square:");
double[][] sqPoints = {{0.0, 1.0}, {1.0, 1.0}, {1.0, 0.0}, {0.0, 0.0}};
Polygon square = new Polygon(sqPoints);
System.out.println("\tPoints: " + square);
System.out.println("\tLength: " + square.calculateLength());
System.out.println("\tBounding Box: " + Arrays.deepToString(square.calculateBoundingBox()));
// A 2 dimensional triangle
System.out.println("Triangle:");
double[][] trPoints = {{-1.0, 0.0}, {-2.0, 0.0}, {-1.0, 1.0}};
Polygon triangle = new Polygon(trPoints);
System.out.println("\tPoints: " + triangle);
System.out.println("\tLength: " + triangle.calculateLength());
System.out.println("\tBounding Box: " + Arrays.deepToString(triangle.calculateBoundingBox()));
// Combine the square and triangle into 1 2D poly
System.out.println("Square append Triangle:");
square.append(triangle);
System.out.println("\tPoints: " + square);
System.out.println("\tLength: " + square.calculateLength());
System.out.println("\tBounding Box: " + Arrays.deepToString(square.calculateBoundingBox()));
// A polygon in 3 dimensions
System.out.println("3D Poly:");
double[][] poly3Points = {{0.0, 1.0, 1.0}, {1.0, 1.0, 2.0}, {1.0, 0.0, 2.0}, {0.0, 0.0, 1.0}};
Polygon poly3D = new Polygon(poly3Points);
System.out.println("\tPoints: " + poly3D);
System.out.println("\tLength: " + poly3D.calculateLength());
System.out.println("\tBounding Box: " + Arrays.deepToString(poly3D.calculateBoundingBox()));
// A polygon in n-dimensions (n=5)
System.out.println("n-Dimensional Poly:");
double[][] polyNPoints = {{-6.5, 1.0, 1.0, 10.2, 5.0}, {-3.0, 1.0, 2.0, 10.0, 5.2}, {1.0, 0.0, 2.0, 8.8, 4.4}, {0.0, 0.0, 1.0, 8.0, 4.0}};
Polygon polyN = new Polygon(polyNPoints);
System.out.println("\tPoints: " + polyN);
System.out.println("\tLength: " + polyN.calculateLength());
System.out.println("\tBounding Box: " + Arrays.deepToString(polyN.calculateBoundingBox()));
// A polygon where the points don't have a consistent dimension
System.out.println("Broken Dimension Poly:");
double[][] brokenPoints = {{-6.5, 1.0, 1.}, {-3.0}, {1.0, 0.0}};
try{
Polygon polyBroken = new Polygon(brokenPoints);
System.out.println("\tPoints: " + polyN);
System.out.println("\tLength: " + polyN.calculateLength());
System.out.println("\tBounding Box: " + Arrays.deepToString(polyN.calculateBoundingBox()));
}catch(IllegalArgumentException e){
System.out.println("\tInvalid polygon");
}
// Try to merge a 2d poly with a 3d poly
System.out.println("Append different dimensions:");
try{
triangle.append(poly3D);
System.out.println("\tPoints: " + triangle);
System.out.println("\tLength: " + triangle.calculateLength());
System.out.println("\tBounding Box: " + Arrays.deepToString(triangle.calculateBoundingBox()));
}catch(IllegalArgumentException e){
System.out.println("\tInvalid polygon");
}
}
}
Polygon.java :
import java.util.Arrays;
public class Polygon
{
private double[][] points;
public Polygon(double[][] points){
// Your code goes here
}
private boolean checkDimensions(double[][] points){
/* This method will check that all of the points in an array have the same dimensions (length) */
// Your code goes here
}
public void append(Polygon p){
/* The points of a second polygon to this polygon */
// Your code goes here
}
public double[][] getPoints(){return this.points;}
public void setPoints(double[][] points){
// Your code goes here
}
public double calculateLength(){
/* Calculate the total length of all the polygon's sides */
// Your code goes here
}
public static double calculateDistance(double[] p1, double[] p2){
/* Calculate the euclidean distance between two given points */
// Your code goes here
}
public double[][] calculateBoundingBox(){
/* to create a bounding box which contains the minimum values for each dimension, and the maximum values */
// Your code goes here
}
public String toString(){
return Arrays.deepToString(this.points);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
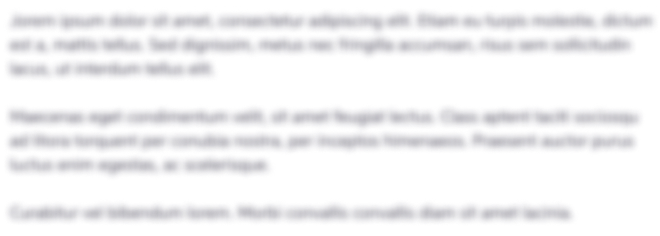
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started