Question
**Requirements** Application Requirements General Requirements This application must be written using the following Java Secure Coding Guidelines (as covered in class): No subclassing allowed (code
**Requirements**
Application Requirements General Requirements This application must be written using the following Java Secure Coding Guidelines (as covered in class):
No subclassing allowed (code reuse is to be done via composition)
Classes can only be instantiated using static constructor methods, e.g. getInstance(). Normal constructors must be declared as private
Objects must be immutable (editing an object means replacing it with a new instance)
User input to the application must be validated. It must not be possible to store invalid attribute values in objects used in the system. Attempting to do so should display an error on-screen, and redisplay the menu to the user
The application must run under the supervision of a SecurityManager with an appropriate policy file to allow data saving and loading
The BaseComputer, LaptopComputer and DesktopComputer classes must be accessed from a sealed .jar file called Domain.jar when the application is run
Exceptions must be sanitized of sensitive data, e.g. file names and paths
Development Platform It is recommended to develop this application in the Kali VM using gedit as the code editor.
(3) Edit a computer To edit the data for an existing computer, enter 6 at the main menu, and then the number of the computer to edit as shown in the computer listing (by entering 1), e.g. to edit the data for computer number 1 (the laptop in the sample data above):
In the example above the user is upgrading the RAM in the laptop to 16GB (but s/he still needs to enter the other data values as well, even if their values are the same as before). Redisplaying the computer data after the laptop has been edited would show the following:
Delete a computer To delete a computer, enter 5 at the main menu, and then the number of the computer to delete, e.g. to delete the desktop computer from our sample data, and show the revised list of computers:
Code I have so far:
package test;
import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.util.ArrayList;
public class Command { //using a arraylist that will be able //to store our items - laptops and desktops static ArrayList
//Using switch to choose appropriate //functions according to choices. switch(choice){ case 1: optionLoad(); break; case 2: optionSave(); break; case 3: optionList(); break; case 4: optionAdd(); break; case 5: //optionDelete(); break; case 6: //optionEdit(); break; case 0: optionLoad(); break; } }while(choice!=0); //Continue showing menu till user exits. } public static void optionLoad(){ } public static void optionSave(){ } //Listing all items in arraylist public static void optionList(){ int sr_no = 1; //If list is empty, display so and exit. if(allItems.size()==0){ System.out.println("Currently, we don't have any items."); return; } //Printing details of each item in list. for(Computer c: allItems){ System.out.println("--------------"); System.out.println("Computer #"+sr_no); sr_no++; c.printDetails(); } } //Adding items to arraylist. public static void optionAdd() throws IOException{ String CPU; int ram,disk; int opt=0; BufferedReader br = new BufferedReader( new InputStreamReader(System.in)); System.out.println("What do you want to add?"); System.out.println("\t1.Laptop \t2.Desktop"); opt = Integer.parseInt(br.readLine()); System.out.println("Enter CPU description:"); CPU = br.readLine(); System.out.println("Enter RAM Size:"); ram = Integer.parseInt(br.readLine()); System.out.println("Enter Disk Space:"); disk = Integer.parseInt(br.readLine()); //Check first what the user wants to add //and create and add items accordingly. if(opt==1){ //user wants to add laptop System.out.println("Enter Screen sixe:"); float scr_size = Float.parseFloat(br.readLine()); allItems.add(new LaptopComputer(CPU,ram,disk,scr_size)); } else{ //user wants to add desktop System.out.println("Enter GPU description:"); String GPU = br.readLine(); allItems.add(new DesktopComputer(CPU,ram,disk,GPU)); } } }
//A base class Computer; it has all the common attributes of // both Laptop and Desktop compters. class Computer{ private String type; private String CPU; private int ram; private int disk;
//Defining constructor, getter and setter functions. public Computer(String type, String CPU, int ram, int disk) { this.type = type; this.CPU = CPU; this.ram = ram; this.disk = disk; } public String getCPU() { return CPU; }
public void setCPU(String CPU) { this.CPU = CPU; }
public int getRam() { return ram; }
public void setRam(int ram) { this.ram = ram; }
public int getDisk() { return disk; }
public void setDisk(int disk) { this.disk = disk; } //Printing details of attributes. public void printDetails(){ System.out.println("CPU: " + getCPU()); System.out.println("RAM: " + getRam() + " GB"); System.out.println("DISK: " + getDisk() + " GB"); }
}
//Class Laptop Computer extends Computer and hence //has all the attributes of Computer class and some // of its own - here, screen_size. class LaptopComputer extends Computer { private float screen_size;
public LaptopComputer(String CPU,int ram, int disk, float screen_size) { //call base class's constructor, // i.e, constructor of Computer class super("DesktoppComputer",CPU,ram,disk); this.screen_size = screen_size; }
public float getScreen_size() { return screen_size; }
public void setScreen_size(int screen_size) { this.screen_size = screen_size; } //Calling base class's printDetails method. @Override public void printDetails(){ System.out.println("Type: LaptopComputer"); super.printDetails(); System.out.println("Screen Size: " + getScreen_size() + " inches"); } }
//Class Desktop Computer extends Computer and hence //has all the attributes of Computer class and some // of its own - here, GPU. class DesktopComputer extends Computer { private String GPU; public DesktopComputer(String CPU,int ram, int disk, String GPU) { //call base class's constructor, // i.e, constructor of Computer class super("LaptopComputer",CPU,ram,disk); this.GPU = GPU; }
public String getGPU() { return GPU; }
public void setGPU(String CPU) { this.GPU = CPU; } @Override public void printDetails(){ System.out.println("Type: DesktopComputer"); //Calling base class's printDetails method. super.printDetails(); System.out.println("GPU: " + getGPU()); } }
2. Exit Enter selection: COMPUTER #1 Type: LaptopComputer CPU: 15 RAM: 8 GB DISK: 250 GB Screen Size: 13 inches COMPUTER #2 Type: Desktop Computer CPU: 17 RAM: 16 GB DISK: 500 GB GPU: nvidia EESMENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit D. Exit Enter selection: EDIT COMPUTER Enter number of computer to edit: 13 Enter CPU type (15/17) : Enter RAM size (8/16): 16 Enter disk size (250/500) : 256 Enter screen size (13/15) : MENUS 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit 9. Exit Enter selection: ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit . Exit Enter selection: COMPUTER #1 Type: Laptop Computer CPU: i5 RAM: 16 GB DISK: 250 GB Screen Size: 13 inches COMPUTER #2 Type: Desktop Computer CPU: 17 RAM: 16 GB DISK: 500 GB GPU: nvidia ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit 0. Exit Enter selection: ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit C. Exit Enter selection: DELETE COMPUTER Enter number of computer to delete: ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit c. Exit Enter selection: COMPUTER #1 Type: Laptop Computer CPU: 15 RAM: 16 GB DISK: 250 GB Screen Size: 13 inches ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit 0. Exit Enter selection: 2. Exit Enter selection: COMPUTER #1 Type: LaptopComputer CPU: 15 RAM: 8 GB DISK: 250 GB Screen Size: 13 inches COMPUTER #2 Type: Desktop Computer CPU: 17 RAM: 16 GB DISK: 500 GB GPU: nvidia EESMENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit D. Exit Enter selection: EDIT COMPUTER Enter number of computer to edit: 13 Enter CPU type (15/17) : Enter RAM size (8/16): 16 Enter disk size (250/500) : 256 Enter screen size (13/15) : MENUS 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit 9. Exit Enter selection: ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit . Exit Enter selection: COMPUTER #1 Type: Laptop Computer CPU: i5 RAM: 16 GB DISK: 250 GB Screen Size: 13 inches COMPUTER #2 Type: Desktop Computer CPU: 17 RAM: 16 GB DISK: 500 GB GPU: nvidia ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit 0. Exit Enter selection: ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit C. Exit Enter selection: DELETE COMPUTER Enter number of computer to delete: ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit c. Exit Enter selection: COMPUTER #1 Type: Laptop Computer CPU: 15 RAM: 16 GB DISK: 250 GB Screen Size: 13 inches ====MENU==== 1. Load 2. Save 3. List 4. Add 5. Delete 6. Edit 0. Exit Enter selectionStep by Step Solution
There are 3 Steps involved in it
Step: 1
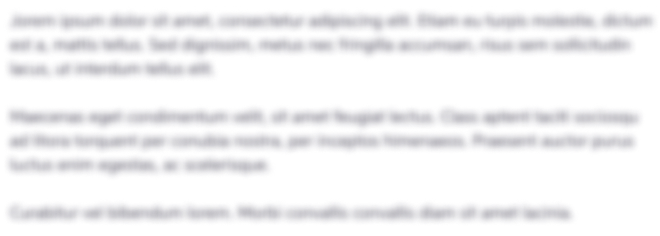
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started