Question
Retail stores need to maintain an accurate record of their inventorythe items available for purchase and the quantity of each item in stock. For this
Retail stores need to maintain an accurate record of their "inventory"the items available for purchase and the quantity of each item in stock. For this project, you will write a program to help a small store manage their inventory. The program will read in the inventory from a file, and allow the user to list items, search for items, and make purchases, which will decrease the item quantities. When the user decides to quit, the updated inventory will be output to a file.
File Format
A common format for data files is one in which the fields on each line are separated by commas. This type of file is known as a Comma-Separated Value (CSV) file and is the type of file used in this project. Excel spreadsheet files, with which you may be familiar, can be exported as CSV files.
The user will provide the initial inventory as a text file in CSV format. When the program ends, the updated inventory will be output as a text file using the same CSV format. Each line of the CSV inventory files used in the project will contain three fields separated by commasthe item identifier (id), the item name, and the item quantity.
Your program will need to determine if the input file is valid, i.e., it contains three values on each line, that the quantity for each item is an integer that is greater than or equal to 0, and that the item ids are unique, i.e, no two items have the same id.
Below is an example of a file that contains the items in an office supply store inventory:
PE0195,pencil,34 MP8917,mechanical pencil,8 DP8768,drawing pencils,5 NP1800,notepad,4 P19076,black pen,35 P90557,blue pen,16 PM1889,black permanent marker,12 DE6785,black dry erase marker,8 DE8079,red dry erase marker,13 BS3045,box of staples,15 PC8866,small paper clips,23 PC9876,large paper clips,12 T91180,tape,20 MT4987,masking tape,45
User Interface Error Checking
You must check for errors in the order given below.
If there are not exactly two arguments on the command line, then the following usage message must be displayed and the program must immediately exit. For example,
If the input file on the command line cannot be accessed, then the following message must be displayed and the program must immediately exit. For example,
If the output file already exists, the user should be asked if it is OK to overwrite the file. If they answer anything that begins with y or Y, the file should be overwritten. Otherwise, it should not be overwritten and the program must exit. For example,
If a FileNotFoundException occurs when attempting to open an output file, the program must output the error message, "Cannot create output file" and exit. For example,
If the input file (a) does not contain three items on each line as desribed above, (b) the quantity for an item is not an integer that is greater than or equal to 0, or (c) two or more items have the same item number, the program must output the error message, "Invalid input file" and exit. For example,
$ java -cp bin Sales test-files/badinventory.txt test-files/updatedInventory.txt Invalid input file
$ java -cp bin Sales test-files/inventory.txt notadirectory/updatedInventory.txt Cannot create output file
$ java -cp bin Sales test-files/inventory.txt test-files/existingfile.txt test-files/existingfile.txt exists - OK to overwrite (y,n)?: yep
$ java -cp bin Sales test-files/notafile.txt test-files/output.txt Unable to access input file: test-files/notafile.txt $
$ java -cp bin Sales Usage: java -cp bin Sales infile outfile $
inventory.txt
PE0195,pencil,34 MP8917,mechanical pencil,8 DP8768,drawing pencils,5 NP1800,notepad,4 P19076,black pen,35 P90557,blue pen,16 PM1889,black permanent marker,12 DE6785,black dry erase marker,8 DE8079,red dry erase marker,13 BS3045,box of staples,15 PC8866,small paper clips,23 PC9876,large paper clips,12 T91180,tape,20 MT4987,masking tape,45
candyInventory.txt
LH8869,Lemonheads,20 AH1289,Airheads,15 GB9806,Gummy Bears,18 JR6578,Jolly Ranchers,12
exp undatedCandyInventory.txt
LH8869,Lemonheads,20 AH1289,Airheads,15 GB9806,Gummy Bears,18 JR6578,Jolly Ranchers,8
Menu
After the inventory has been successfully read in from the file, the program must continually prompt the user with the following menu until they desire to quit.
Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option:
The program must accept and use both upper and lower case letters for the options. If the user enters an invalid option, the program shall output an error message and redisplay the menu. For example,
Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: search Invalid option Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option:
Sample input/output for each of the options is provided below. For the search and list options, each item must be displayed on its own line and in the same order as it occurs in the input file. If no items meet the search criterion, nothing is output for that search.
The file inventory.txt contains the inventory information used in the first two option examples given below. The candyInventory.txt file contains the inventory information used in the last example and exp_updatedCandyInventory.txt contains the expected content of the output file when the example is complete. However, your program must work for any properly formatted file of inventory information. Be sure to download and work with these files as a text files.
List items
The file inventory.txt contains the inventory information this example.
Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: l ID Name Quantity PE0195 pencil 34 MP8917 mechanical pencil 8 DP8768 drawing pencils 5 NP1800 notepad 4 P19076 black pen 35 P90557 blue pen 16 PM1889 black permanent marker 12 DE6785 black dry erase marker 8 DE8079 red dry erase marker 13 BS3045 box of staples 15 PC8866 small paper clips 23 PC9876 large paper clips 12 T91180 tape 20 MT4987 masking tape 45 Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option:
Search by item name
The file inventory.txt contains the inventory information this example.
Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: S Item name (is/contains): pen ID Name Quantity PE0195 pencil 34 MP8917 mechanical pencil 8 DP8768 drawing pencils 5 P19076 black pen 35 P90557 blue pen 16 Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: s Item name (is/contains): ck p ID Name Quantity P19076 black pen 35 PM1889 black permanent marker 12 Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: S Item name (is/contains): xyz ID Name Quantity Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option:
Purchase item
The candyInventory.txt file contains the inventory information used in this example, and exp_updatedCandyInventory.txt contains the expected content of the output file when the example is complete.
If the item id does not exist, the program must output the error message "Invalid item" and redisplay the menu. If the quantity is not an integer with a value greater than or equal to 0, the program must output the error message "Invalid quantity" and redisplay the menu. If the quantity is not less than or equal to the item quantity in stock, the program must output the error message "Insufficient quantity" and redisplay the menu, otherwise, the message "Successful purchase" is output and the menu is redisplayed.
Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: l ID Name Quantity LH8869 Lemonheads 20 AH1289 Airheads 15 GB9806 Gummy Bears 18 JR6578 Jolly Ranchers 12 Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: P Item id: xyz Invalid item Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: p Item id: JR6578 Quantity: abc Invalid quantity Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: p Item id: JR6578 Quantity: -5 Invalid quantity Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: p Item id: JR6578 Quantity: 100 Insufficient quantity Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: l ID Name Quantity LH8869 Lemonheads 20 AH1289 Airheads 15 GB9806 Gummy Bears 18 JR6578 Jolly Ranchers 12 Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: p Item id: JR6578 Quantity: 4 Successful purchase Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: L ID Name Quantity LH8869 Lemonheads 20 AH1289 Airheads 15 GB9806 Gummy Bears 18 JR6578 Jolly Ranchers 8 Sales Program - Please choose an option. L - List items S - Search by item name P - Purchase item Q - Quit Option: Q
The program must contain and use the completed version of the methods below. The methods must work correctly for all valid parameter values, not just the ones used in the project. You are free to (and encouraged to) define and use additional methods, if you like - be sure to provide Javadoc for them.
NOTE: Each method below that has a Scanner parameter for an input file assumes that the Scanner is starting at the beginning of the file - you will need to create a new Scanner for the input file before the method is called!
Replace the comments below with appropriate Javadoc.
// Returns the number of lines in the file // // Throws an IllegalArgumentException with the message // "Null file" if in is null public static int getNumberOfLines(Scanner in) { }
// Reads each line of the input file, and stores the item number, name, quantity // in the appropriate array parameter // Returns true, if successful // Returns false, if a line of the input file // (a) does not contain exactly three values as described above, // (b) the quantity for an item is not an integer that is greater than or equal to 0, // (c) or two or more items have the same id // // Throws an IllegalArgumentException with the message // "Null file" if in is null // // Throws an IllegalArgumentException with the message // "Null array" if itemIds, itemNames, and/or itemQuantities are/is null // // Throws an IllegalArgumentException with the message "Invalid array length" if // (a) the lengths of itemIds, itemNames, and/or itemQuantities are not the same OR // (b) if the number of lines in the file is not the same as the length of the arrays, // which may be detected when reading the lines of the file // // NOTE: You must check for invalid parameters (arguments) in the order given above. public static boolean inputInventory(Scanner in, String[] itemIds, String[] itemNames, int[] itemQuantities) { }
// Returns a String with each item id, name, and quantity formatted as shown above // with a newline character after the String for each item. // // Use the provided toString() method below to ensure // that the formatting of each line is correct. // // Throws an IllegalArgumentException with the message // "Null array" if itemIds, itemNames, and/or itemQuantities are/is null // // Throws an IllegalArgumentException with the message // "Invalid array length" if the lengths of itemIds, itemNames, and itemQuantities arrays // are not the same // // NOTE: You must check for invalid parameters (arguments) in the order given above. public static String getList(String[] itemIds, String[] itemNames, int[] itemQuantities) { }
// Returns a String with each item whose name is/contains itemName, disregarding case, // with the item id, name, and quantity formatted as shown above // with a newline character after the String for each item. // // Use the provided toString() method below to ensure that the formatting of each line is // correct. // // If none of the items have a name that is or contains itemName, // the empty String ("") is returned. // // Throws an IllegalArgumentException with the message // "Null array" if itemIds, itemNames, and/or itemQuantities are/is null // // Throws an IllegalArgumentException with the message // "Invalid array length" if the lengths of itemIds, itemNames, and itemQuantities arrays // are not the same // // NOTE: You must check for invalid parameters (arguments) in the order given above. public static String searchByName(String[] itemIds, String[] itemNames, int[] itemQuantities, String itemName) { }
// Returns formatted string for item // DO NOT CHANGE METHOD HEADER OR METHOD CONTENTS! public static String toString(String itemId, String itemName, int itemQuantity) { return String.format("%6s %-25s %4d", itemId, itemName, itemQuantity); }
// If the item's quantity is < itemQuantity, "Insufficient quantity" is returned. // else the item's quantity is decreased by itemQuantity and "Successful purchase" is returned. // // Throws an IllegalArgumentException with the message // "Null array" if itemIds or itemQuantities is null // // Throws an IllegalArgumentException with the message // "Invalid array length" if the lengths of itemIds and itemQuantities arrays // are not the same // // Throws an IllegalArgumentException with the message // "Invalid item" if the itemId does not exist // // Throws an IllegalArgumentException with the message // "Invalid quantity" if itemQuantity is < 0 // // NOTE: You must check for invalid parameters (arguments) in the order given above. public static String makePurchase(String[] itemIds, int[] itemQuantities, String itemId, int itemQuantity) { }
// Outputs the inventory data to the file in CSV format as described above. // // Throws an IllegalArgumentException with the message // "Null file" if out is null // // Throws an IllegalArgumentException with the message // "Null array" if itemIds, itemNames, and/or itemQuantities are/is null // // Throws an IllegalArgumentException with the message // "Invalid array length" if the lengths of itemIds, itemNames, and itemQuantities arrays // are not the same // // NOTE: You must check for invalid parameters (arguments) in the order given above. public static void outputInventory(PrintWriter out, String[] itemIds, String[] itemNames, int[] itemQuantities) { }
The following hints will help you with the implementation of your software.
Scanners Hints
- Create only one Scanner for keyboard input (System.in) for use throughout your program. You will be penalized if your program creates more than one Scanner for keyboard input.
- Each method that takes a file Scanner as a parameter assumes the Scanner starts at the beginning of the file. You may need to create a new Scanner before calling the method.
- Because the input file is a CSV file in which fields are separated by commas, you will need to set the delimiter for a line scanner to a comma, as follows:
You can then use the line scanner with the usual methods. This will allow you to read an item name with scan.next() even though it contains more than one token.
PrintWriter Hint
- Be sure to close the output PrintWriter.
Exceptions Hints
- Do NOT use throws clause(s) in method headers within your program. Instead use try/catch blocks within your methods.
- Check for invalid parameters and throw an IllegalArgumentException, if necessary, at the beginning of methods that require them, as much as possible.
Windows Line Breaks Hint
- If you are working on a Windows computer, the lines in your output file may end with the Windows standard CR LF (Carriage Return Line Feed) characters instead of the single Linux standard LF (Line Feed) character. One way to get around this is to use the print() method with a newline character rather than the println() method. For example,
Menu Hint
Below is a structure that you may find helpful for the menu processing part of your program:
String option = ""; while (!option.equalsIgnoreCase("Q")) { displayMenu(); option = scnr.next(); if (option.equalsIgnoreCase("L")) { //Add code here } else if (option.equalsIgnoreCase("S")) { //Add code here } else if (option.equalsIgnoreCase("P")) { //Add code here } else if (!option.equalsIgnoreCase("Q")) { //Add code here } } //Add code here } public static void displayMenu() { //Add code here }
out.print(line + " ");
Scanner scan = new Scanner(line); scan.useDelimiter(",");
Step by Step Solution
There are 3 Steps involved in it
Step: 1
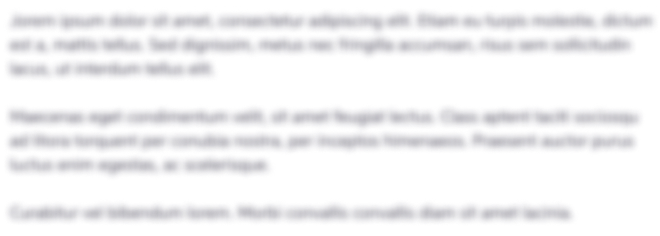
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started