Question
Rewrite this java program into MIPS assembly language. This program takes the array 10, 3, 5, 2, 11, 232, 2, 0 and displays it in
Rewrite this java program into MIPS assembly language. This program takes the array 10, 3, 5, 2, 11, 232, 2, 0 and displays it in descending order using bubblesort.
public class Bubblesort {
public static void bubbleSort( int [ ] num ){
int j;
boolean flag = true; // set flag to true to begin first pass
int temp; //holding variable
while ( flag ){
flag= false; //set flag to false awaiting a possible swap
for( j=0; j < num.length -1; j++ ){
if ( num[ j ] < num[j+1] ) // change to > for ascending sort
{
temp = num[ j ]; //swap elements
num[ j ] = num[ j+1 ];
num[ j+1 ] = temp;
flag = true; //shows a swap occurred
}
}
}
}
public static void main(String [] args) {
int intArray[] = { 10, 3, 5, 2, 11, 232, 2, 0};
bubbleSort(intArray);
for(int i: intArray){
System.out.print(i + " ");
}
System.out.println(" ");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
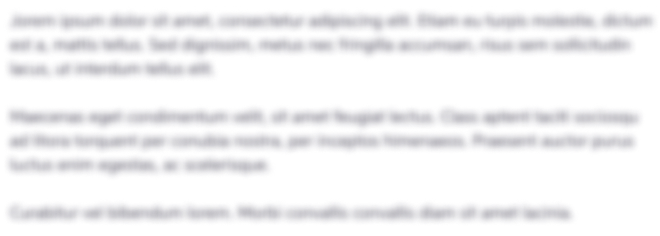
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started