Question
robotsMain.s # A proper program header goes here... # # .data x: .word 0:4 # x-coordinates of 4 robots y: .word 0:4 # y-coordinates of
robotsMain.s # A proper program header goes here... # # .data x: .word 0:4 # x-coordinates of 4 robots y: .word 0:4 # y-coordinates of 4 robots
str1: .asciiz "Your coordinates: 25 25 " str2: .asciiz "Enter move (1 for +x, -1 for -x, 2 for + y, -2 for -y):" str3: .asciiz "Your coordinates: " sp: .asciiz " " endl: .asciiz " " str4: .asciiz "Robot at " str5: .asciiz "AAAARRRRGHHHHH... Game over " #i $s0 #myX $s1 #myY $s2 #move $s3 #status $s4 #temp,pointers $s5,$s6 .text .globl inc .globl getNew
main: li $s1,25 # myX = 25 li $s2,25 # myY = 25 li $s4,1 # status = 1
la $s5,x la $s6,y
sw $0,($s5) # x[0] = 0; y[0] = 0; sw $0,($s6) sw $0,4($s5) # x[1] = 0; y[1] = 50; li $s7,50 sw $s7,4($s6) sw $s7,8($s5) # x[2] = 50; y[2] = 0; sw $0,8($s6) sw $s7,12($s5) # x[3] = 50; y[3] = 50; sw $s7,12($s6)
la $a0,str1 # cout << "Your coordinates: 25 25 "; li $v0,4 syscall bne $s4,1,exitw # while (status == 1) { while: la $a0,str2 # cout << "Enter move (1 for +x, li $v0,4 # -1 for -x, 2 for + y, -2 for -y):"; syscall li $v0,5 # cin >> move; syscall move $s3,$v0
bne $s3,1,else1 # if (move == 1) add $s1,$s1,1 # myX++; b exitif else1: bne $s3,-1,else2 # else if (move == -1) add $s1,$s1,-1 # myX--; b exitif else2: bne $s3,2,else3 # else if (move == 2) add $s2,$s2,1 # myY++; b exitif else3: bne $s3,-2,exitif # else if (move == -2) add $s2,$s2,-1 # myY--; exitif: la $a0,x # status = moveRobots(&x[0],&y[0],myX,myY); la $a1,y move $a2,$s1 move $a3,$s2 jal moveRobots move $s4,$v0
la $a0,str3 # cout << "Your coordinates: " << myX li $v0,4 # << " " << myY << endl; syscall move $a0,$s1 li $v0,1 syscall la $a0,sp li $v0,4 syscall move $a0,$s2 li $v0,1 syscall la $a0,endl li $v0,4 syscall
la $s5,x la $s6,y li $s0,0 # for (i=0;i<4;i++) for: la $a0,str4 # cout << "Robot at " << x[i] << " " li $v0,4 # << y[i] << endl; syscall lw $a0,($s5) li $v0,1 syscall la $a0,sp li $v0,4 syscall lw $a0,($s6) li $v0,1 syscall la $a0,endl li $v0,4 syscall add $s5,$s5,4 add $s6,$s6,4 add $s0,$s0,1 blt $s0,4,for
beq $s4,1,while # } exitw: la $a0,str5 # cout << "AAAARRRRGHHHHH... Game over "; li $v0,4 syscall li $v0,10 #} syscall
robots.cc
#include
int moveRobots(int *, int *, int, int ); int getNew(int, int);
int main() { int x[4], y[4], i, j, myX = 25, myY = 25, move, status = 1;
// initialize positions of four robots x[0] = 0; y[0] = 0; x[1] = 0; y[1] = 50; x[2] = 50; y[2] = 0; x[3] = 50; y[3] = 50;
cout << "Your coordinates: 25 25 ";
while (status == 1) { cout << "Enter move (1 for +x, -1 for -x, 2 for + y, -2 for -y):"; cin >> move;
// process user's move if (move == 1) myX++; else if (move == -1) myX--; else if (move == 2) myY++; else if (move == -2) myY--;
// update robot positions status = moveRobots(&x[0],&y[0],myX,myY);
cout << "Your coordinates: " << myX << " " << myY << endl; for (i=0;i<4;i++) cout << "Robot at " << x[i] << " " << y[i] << endl;
} cout << "AAAARRRRGHHHHH... Game over "; }
int moveRobots(int *arg0, int *arg1, int arg2, int arg3) { int i, *ptrX, *ptrY, alive = 1;
ptrX = arg0; ptrY = arg1; for (i=0;i<4;i++) { *ptrX = getNew(*ptrX,arg2); // update x-coordinate of robot i *ptrY = getNew(*ptrY,arg3); // update y-coordinate of robot i
// check if robot caught user if ((*ptrX == arg2) && (*ptrY == arg3)) { alive = 0; break; } ptrX++; ptrY++; } return alive; }
// move coordinate of robot closer to coordinate of user int getNew(int arg0, int arg1) { int temp, result;
temp = arg0 - arg1; if (temp >= 10) result = arg0 - 10; else if (temp > 0) result = arg0 - 1; else if (temp == 0) result = arg0; else if (temp > -10) result = arg0 + 1; else if (temp <= -10) result = arg0 + 10;
return result; }
For this project, you are given a program that implements a game where a human user tries to escape from four robots. The user and four robots are on an x-y grid. On every step, you enter a move for the human. The robots will attempt to get closer to the human. When a robot has the same x-y coordinates as the human, the game is over. You will translate this program faithfully, following all function call guidelines and MIPS register use conventions.
Two arrays x[4] and y[4] keep track of the x- and y-coordinates of four robots. The positions of the human and the four robots are initialized in the program. On each step, the user enters a move; the positions of the human and the robots are updated. This continues until the human dies.
In the main loop, the user is prompted to enter a move. The position of the user is updated. Then the program calls a function moveRobots()to update the position of the robots as they try to catch the human. The new positions of the human and the robots are then displayed.
The function moveRobots() has prototypeint moveRobots(int *arg0, int *arg1, int arg2, int arg3)
arg0 is the base address of array that contains the x-coordinates of the four robots, arg1 is the base address of array that contains the y-coordinates of the four robots, arg2 is the x- coordinate of the human, arg3 is the y-coordinate of the human. moveRobots()updates the positions of the four robots, and returns a 1 if the human is alive, and a 0 if the human is dead (i.e., the human has the same coordinates as a robot). Each coordinate of a robot is updated by calling the function getNew(), which returns the new coordinate based on the current coordinate of the robot and the current coordinate of the human.
When you translate moveRobots() to MIPS assembly language, arg0 through arg3 are in $a0 through $a3; the return value is in $v0.
The function getNew() uses simple rules to move a robot closer to the human. If the difference in the coordinates is >=10, the robot's coordinate will move 10 units closer to the human. If the difference in the coordinates is < 10, the robot's coordinate willmove one unit closer to the human. (See program listings.) getNew() has prototype
int getNew(int arg0, int arg1)
arg0 is the coordinate (x or y) of a robot, arg1 is the coordinate (x or y) of the human. getNew() returns the new coordinate of the robot, based on the position of the human.
When you translate getNew() to MIPS assembly language, arg0 and arg1 are in $a0 and $a1 respectively, and the return value is in $v0.
A copy of the C++ program robots.cc can be found on iLearn. The file robotsMain.s contains the main program, already translated into MIPS assembly language. Your functions will go in a separate file. To run the main program and functions together, all you have to do is load them separately into spim. Suppose the main program is in p1main.s and the functions in p1.s:
(spim) load "p1main.s" (spim) load "p1.s" (spim) run
Write the functions exactly as described in this handout. Do not implement the program using other algorithms or tricks. Do not even switch the order of the arguments in function calls; you must follow the order specified in the C++ code. The purpose of this program is to test whether you understand nested functions. If you wish to make changes to the algorithm, you must first check with the instructor.
Your functions should be properly commented. Each function must have its own header block, including the prototype of the function, the locations of all arguments and return values, descriptions of the arguments and how they are passed, and a description of what the function does. Paste in the C++ code as inline comments for your MIPS assembly code. Refer to the Programming Style handout from Chapter 2 slides and
http://unixlab.sfsu.edu/~whsu/csc256/PROGS/4.2for guidelines on how to comment your code.
You should try to make your code efficient. For example, your loops should follow the efficient form presented in class. Points will be deducted for obvious inefficiencies.
Submission: submit your code via iLearn. All your code (two functions) should be in a single plain text file. Do not include the main program!
80% of your grade is for correctness. 20% is for clarity/documentation.
#include
int moveRobots(int *, int *, int, int ); int getNew(int, int);
int main() {
int x[4], y[4], i, j, myX = 25, myY = 25, move, status = 1;
// initialize positions of four robots
x[0] = 0; y[0] = 0; x[1] = 0; y[1] = 50; x[2] = 50; y[2] = 0; x[3] = 50; y[3] = 50;
cout << "Your coordinates: 25 25 ";
while (status == 1) {cout << "Enter move (1 for +x, -1 for -x, 2 for + y, -2 for -y):"; cin >> move;
// process user's move
if (move == 1) myX++;
else if (move == -1) myX--;
else if (move == 2) myY++;
else if (move == -2) myY--;
// update robot positions
status = moveRobots(&x[0],&y[0],myX,myY);
cout << "Your coordinates: " << myX << " " << myY << endl;
for (i=0;i<4;i++)cout << "Robot at " << x[i] << " " << y[i] << endl;
}
cout << "AAAARRRRGHHHHH... Game over "; }
int moveRobots(int *arg0, int *arg1, int arg2, int arg3) {
int i, *ptrX, *ptrY, alive = 1; ptrX = arg0;
ptrY = arg1;
for (i=0;i<4;i++) {*ptrX = getNew(*ptrX,arg2); // update x-coordinate of robot i *ptrY = getNew(*ptrY,arg3); // update y-coordinate of robot i
// check if robot caught user
if ((*ptrX == arg2) && (*ptrY == arg3)) { alive = 0;break;
}
ptrX++;
ptrY++;
}
return alive; }
// move coordinate of robot closer to coordinate of user
int getNew(int arg0, int arg1) {
int temp, result;
temp = arg0 - arg1; if (temp >= 10)
result = arg0 - 10; else if (temp > 0)
result = arg0 - 1; else if (temp == 0)
result = arg0; else if (temp > -10) result = arg0 + 1;
else if (temp <= -10) result = arg0 + 10;
return result; }
libra% a.outYour coordinates: 25 25Enter move (1 for +x, -1 for -x, 2 for + y, -2 for -y):2 Your coordinates: 25 26Robot at 10 10Robot at 10 40Robot at 40 10Robot at 40 40Enter move (1 for +x, -1 for -x, 2 for + y, -2 for -y):1 Your coordinates: 26 26Robot at 20 20Robot at 20 30Robot at 30 20Robot at 30 30Enter move (1 for +x, -1 for -x, 2 for + y, -2 for -y):2 Your coordinates: 26 27Robot at 21 21Robot at 21 29Robot at 29 21Robot at 29 29Enter move (1 for +x, -1 for -x, 2 for + y, -2 for -y):1 Your coordinates: 27 27Robot at 22 22Robot at 22 28Robot at 28 22Robot at 28 28Enter move (1 for +x, -1 for -x, 2 for + y, -2 for -y):2 Your coordinates: 27 28Robot at 23 23Robot at 23 28Robot at 27 23Robot at 27 28AAAARRRRGHHHHH... Game overlibra%
Step by Step Solution
There are 3 Steps involved in it
Step: 1
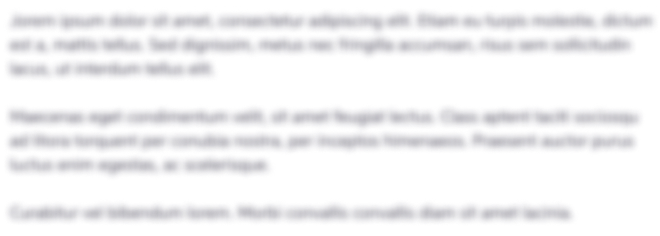
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started