Rock Paper Scissors Game in Java This may seem incredibly simple at first, but it's not quite as simple as you might think. Think about
Rock Paper Scissors Game in Java
This may seem incredibly simple at first, but it's not quite as simple as you might think. Think about how a "Rock, Paper, Scissors" game is played:
Two players give one of three choices (Rock, Paper, or Scissors) at roughly the same time.
A winner is determined for that instance:
o Rock beats Scissors o Scissors beats Paper o Paper beats Rock o A tie happens if both players chooses the same thing
Both players repeat until a winner is decided to conclude the game o Commonly, a game is decided by a "best two out of three"rule:
A player must win at least two "rounds"
A round happens when a choice a player makes is compared to a choice
the opposing player makes The winner must win at least one more round than the other player
o The simplest scenario is when a player wins the first two rounds o Note that if a player doesn't win the first two rounds, the players must play at least four
rounds to determine the winner
This is intended to be a simplified version of the game so you don't have to worry about random number generators. However, you do need to enforce invariants (e.g. is it possible for a player to make a "Shotgun beats all!" choice?).
Class Design: Player (Suggested Time Spent: < 10 minutes)
The Player class is intended to be an abstract and simplified representation of someone playing a "Rock, Paper, Scissors" game.
Class Properties (MUST be private)
Name or nickname of player
Array storing the player's choices for all rounds in a game
Class Invariants
Player name must be at least three characters long.
Choices can only be "Rock," "Paper," or "Scissors"
Class Components
Constructor that takes in as argument the player's name (enforce invariants)
Public Getters for name and choices
o Optional: Private Setter for name and choices
Public method that takes in a choice and stores it in the array of choices (enforce invariants)
Class Design: Game (Suggested Time Spent: < 30 minutes)
The Game class is intended to be an abstract and simplified representation of a "Rock, Paper, Scissors" game. This object should keep track of players and outcomes for the game.
Class Properties (MUST be private)
First player
Second player
Class Invariants
Players must have different names (otherwise ambiguous as to who the winner is)
Players must each have made at least one choice (at least one round played)
Class Components
Constructor that takes in as arguments two players (enforce invariants)
NO public getters or setters (private getters/setters acceptable)
Public method that returns the name of the winner of the round
Player 1
o You will need to compare the choices that the players have made and determine who the winner is - assume "best 2 out of 3" rule
If a player only made two choices and won those two choices, they won regardless of how many choices the other player made
If both players only played enough rounds to end in a tie, you must determine what to return in the case of a tie If a player did not make enough choices to win, that player is considered to have forfeit the game and the other player wins. Examples:
Rock Rock Paper
2
Scissors Paper Paper Rock
Player This results in "Player 2" winning because it was a tie when "Player 1" gave up.
Player Player
Driver Class: GameDriver (Suggested Time Spent: < 5 minutes)
The GameDriver class is intended to be a simple driver to test the Game object:
Make two players (e.g. player 1 and player 2)
Add some choices to each player such that player 1 wins
Make a Game
Print out the winner of the game
Make another game that results in a tie
Step by Step Solution
There are 3 Steps involved in it
Step: 1
The detailed answer for the above question is provided below Heres a Java implementation according to the specifications provided java import javautil...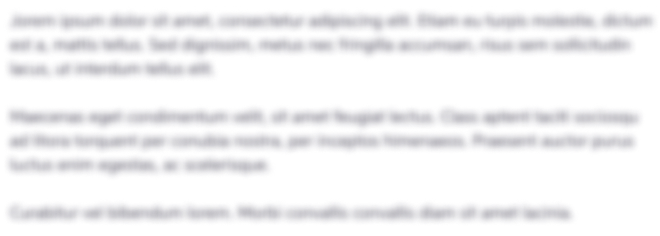
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started