Question
Ruby classes can be altered during the execution of a program. We can modify our Student class to add, modify, or overwrite existing methods. In
Ruby classes can be altered during the execution of a program. We can modify our Student class to add, modify, or overwrite existing methods. In this example well create 7 pairs of accessors for properties name @user_name, @password, @uid, @gid,@gcos_field, @home_directory, and @shell:
class Student attr_accessor :user_name, :password, :uid, :gid, :gcos_field, :home_directory, :shell end
We'll use course CS 132A Section 831 with a CRN of 36318 as an example for this progranm.
In this lab you will use an ERB template named lab4.txt.erb to display each students data as formatted text.
Create an alternating_case method for the String class
Ruby allows us to open any class, even system classes, to modify existing, or add new methods. Heres an example that shows how to add a method to the a built-in String class.
# Open the class and add the method humanize # String#humanize converts underscores to spaces; used to make # database table names more human friendly class String def humanize # self indicates the current instance self.gsub('_', ' ') end end inhuman = 'user_name' puts "\"#{inhuman}\" becomes \"#{inhuman.humanize.upcase}\"."
"user_name" becomes "USER NAME".
For this lab you will add a new method named alternating_case to the String class. This method will return a string in which the characters alternate between lower and upper case.
When your print the output of all the student data, use the alternating_case String method to print each students GCOS field.
Example output
str = 'This is a string.' puts str.alternating_case #=> tHiS Is a sTrInG.
TODO: Create a Ruby class named Student to model the information contained in each users line in /etc/passwd. The /etc/passwd file contains basic user information and is public information on all Unix systems.
Your script will read the /etc/group file to get the user names of the students in our class. Use the CRN number for your section of the course.
User your modified String class in your lab4.rb file.
Create a class named Student. If youve completed the Week 7 Exercises you already have this class and can make a few modifications for this lab.
The Student class will have an instance variable and accessors for each of the 7 fields in each students line in /etc/passwd. The instance variables for the data will be:
@user_name
@password
@uid
@gid
@gcos_field
@directory
@shell
Create a class variable named @@count to keep a count of the number of student instances. Each time a new instance is created, increment @@count by one.
Each instance of Student will also have an instance variable named @count which will contain the value of @@count when the instance was created. Create a read accessor for @count. You do not need a write accessor.
Assign the value in the class variable @@count to each new instances @count variable. Print @countin the first column of the listing for each student, following the example.
Using the Student class and the information derived from the /etc/group file, create an instance for each class member. The Hills /etc/group file contains a line for each course. The group line for our course begins with a name what looks like this: cNNNN,c followed by four digits. Your script must extract this line each time it runs. Do not copy the class group line from /etc/group into your script. You must use Ruby to extract the data from the system files.
Populate each instances attributes with data derived from the students unique line in /etc/passwd. Those attributes are listed above. See the example Student class below for one way of creating new Student instances.
Create an ERB template file named lab4.txt.erb. Display each students formatted data using this ERB page.
Use the lab4.txt.erb ERB template to print each students formatted data. Look carefully at friendly_letter template for guidelines.
When printing the students GCOS data, use the alternating_case method you added to the String class. Consult the example output to see how this should look (it looks weird): Example output for Lab 4.
lab4.rb must read the correct line from /etc/passwd file for each student to extract the user information.
Create the Student information as formatted plain text using columns.
Print the elapsed time it takes for your script to run. Use the Time class to accomplish this. Start timing at the beginning of your script and print the elapsed time at the end of the script.
Example Output:
http://hills.ccsf.edu/~dputnam/cs132a/lab4_plain_text.cgi
Step by Step Solution
There are 3 Steps involved in it
Step: 1
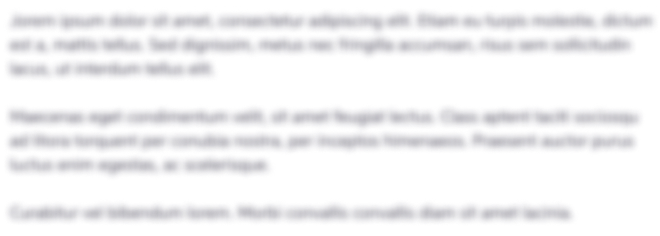
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started