Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Running into issues with running my code. Previously code would note load media list. Was advised to add- private void jMenuItem1ActionPerformed(java.awt.event.ActionEvent evt) { JFileChooser chooser
Running into issues with running my code.
Previously code would note load media list. Was advised to add-
private void jMenuItem1ActionPerformed(java.awt.event.ActionEvent evt) { JFileChooser chooser = new JFileChooser(); int fChooser = chooser.showOpenDialog(null); if (fChooser == JFileChooser.APPROVE_OPTION) { System.out.println("You chose to open this file: " + chooser.getSelectedFile().getName()); File file = chooser.getSelectedFile(); String filename = file.getName(); System.out.println("You have selected: " + filename); manager.LoadMedia(filename); //ISSUE } } Here we need to provide filePath instead of filename only. Fix Issue : Find Path Name using file.getPath(); method then add this path to LoadMedia method as shown below. private void jMenuItem1ActionPerformed(java.awt.event.ActionEvent evt) { JFileChooser chooser = new JFileChooser(); int fChooser = chooser.showOpenDialog(null); if (fChooser == JFileChooser.APPROVE_OPTION) { System.out.println("You chose to open this file: " + chooser.getSelectedFile().getName()); File file = chooser.getSelectedFile(); String path = file.getPath(); String filename = file.getName(); System.out.println("You have selected: " + filename); manager.LoadMedia(path); } }
When I added I now get these errors-
Exception in thread "AWT-EventQueue-0" java.lang.NumberFormatException: For input string: "true" at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:67) at java.base/java.lang.Integer.parseInt(Integer.java:668) at java.base/java.lang.Integer.parseInt(Integer.java:786) at project.Manager.LoadMedia(Manager.java:29) at project.MediaRentalSystem.jMenuItem1ActionPerformed(MediaRentalSystem.java:101) at project.MediaRentalSystem$2.actionPerformed(MediaRentalSystem.java:45) at java.desktop/javax.swing.AbstractButton.fireActionPerformed(AbstractButton.java:1972) at java.desktop/javax.swing.AbstractButton$Handler.actionPerformed(AbstractButton.java:2313) at java.desktop/javax.swing.DefaultButtonModel.fireActionPerformed(DefaultButtonModel.java:405) at java.desktop/javax.swing.DefaultButtonModel.setPressed(DefaultButtonModel.java:262) at java.desktop/javax.swing.AbstractButton.doClick(AbstractButton.java:374) at java.desktop/javax.swing.plaf.basic.BasicMenuItemUI.doClick(BasicMenuItemUI.java:1028) at java.desktop/javax.swing.plaf.basic.BasicMenuItemUI$Handler.mouseReleased(BasicMenuItemUI.java:1072) at java.desktop/java.awt.AWTEventMulticaster.mouseReleased(AWTEventMulticaster.java:297) at java.desktop/java.awt.Component.processMouseEvent(Component.java:6626) at java.desktop/javax.swing.JComponent.processMouseEvent(JComponent.java:3389) at java.desktop/java.awt.Component.processEvent(Component.java:6391) at java.desktop/java.awt.Container.processEvent(Container.java:2266) at java.desktop/java.awt.Component.dispatchEventImpl(Component.java:5001) at java.desktop/java.awt.Container.dispatchEventImpl(Container.java:2324) at java.desktop/java.awt.Component.dispatchEvent(Component.java:4833) at java.desktop/java.awt.LightweightDispatcher.retargetMouseEvent(Container.java:4948) at java.desktop/java.awt.LightweightDispatcher.processMouseEvent(Container.java:4575) at java.desktop/java.awt.LightweightDispatcher.dispatchEvent(Container.java:4516) at java.desktop/java.awt.Container.dispatchEventImpl(Container.java:2310) at java.desktop/java.awt.Window.dispatchEventImpl(Window.java:2780) at java.desktop/java.awt.Component.dispatchEvent(Component.java:4833) at java.desktop/java.awt.EventQueue.dispatchEventImpl(EventQueue.java:773) at java.desktop/java.awt.EventQueue$4.run(EventQueue.java:722) at java.desktop/java.awt.EventQueue$4.run(EventQueue.java:716) at java.base/java.security.AccessController.doPrivileged(AccessController.java:399) at java.base/java.security.ProtectionDomain$JavaSecurityAccessImpl.doIntersectionPrivilege(ProtectionDomain.java:86) at java.base/java.security.ProtectionDomain$JavaSecurityAccessImpl.doIntersectionPrivilege(ProtectionDomain.java:97) at java.desktop/java.awt.EventQueue$5.run(EventQueue.java:746) at java.desktop/java.awt.EventQueue$5.run(EventQueue.java:744) at java.base/java.security.AccessController.doPrivileged(AccessController.java:399) at java.base/java.security.ProtectionDomain$JavaSecurityAccessImpl.doIntersectionPrivilege(ProtectionDomain.java:86) at java.desktop/java.awt.EventQueue.dispatchEvent(EventQueue.java:743) at java.desktop/java.awt.EventDispatchThread.pumpOneEventForFilters(EventDispatchThread.java:203) at java.desktop/java.awt.EventDispatchThread.pumpEventsForFilter(EventDispatchThread.java:124) at java.desktop/java.awt.EventDispatchThread.pumpEventsForHierarchy(EventDispatchThread.java:113) at java.desktop/java.awt.EventDispatchThread.pumpEvents(EventDispatchThread.java:109) at java.desktop/java.awt.EventDispatchThread.pumpEvents(EventDispatchThread.java:101) at java.desktop/java.awt.EventDispatchThread.run(EventDispatchThread.java:90)
The previous code was this
When I run the below code I get "File cannot be opened: Could not load, no such directory".
public class Media { // Attributes int id; String title; int year; boolean available; // Constructor public Media(int id, String title, int year, boolean available) { this.id = id; this.title = title; this.year = year; this.available = available;} // Get methods public int getId() { return id;} public void setId(int id) { this.id = id;} public String getTitle() { return title;} public void setTitle(String title) { this.title = title;} public int getYear() { return year;} public void setYear(int year) { this.year = year;} public boolean isAvailable() { return available;} public void setAvailable(boolean available) { this.available = available;} // Calculate rental fee; flat fee $3.00 public double calculateRentalFee() { return 3.00;} }
import java.util.Calendar; public class EBook extends Media { // Local Attributes private int numChapters; // Constructor public EBook(int id, String title, int year, boolean available, int numChapters) { super(id, title, year, available); this.numChapters = numChapters;} // Get Method public int getNumChapters() { return numChapters;} // Set Method public void setNumChapters(int numChapters) { this.numChapters = numChapters;} @Override public double calculateRentalFee() { double fee = numChapters * 0.10; int currYear = Calendar.getInstance().get(Calendar.YEAR); if (this.getYear() == currYear) { fee += 1;} return fee;} @Override public String toString() { return "EBook [id:" + this.id + " title:" + this.title + " chapter:" + this.numChapters + " year:" + this.year + " available:" + this.available + "]n";} }
public class MovieDVD extends Media { // Local Attributes private double size; // Constructor public MovieDVD(int id, String title, int year, boolean available, double size) { super(id, title, year, available); this.size = size;} //Get Method public double getSize() { return size;} //Set Method public void setSize(double size) { this.size = size;} @Override public double calculateRentalFee() { return super.calculateRentalFee();} @Override public String toString() { return "MovieDVD [id:" + this.getId() + " title:" + this.getTitle() + " size:" + this.getSize()+ " year:" + this.getYear() + " available:" + this.isAvailable() + "]n";} }
import java.util.Calendar; public class MusicCD extends Media { // Local Attributes private int length; // Constructor public MusicCD(int id, String title, int year, boolean available, int length) { super(id, title, year, available); this.length = length;} // Get Method public int getLength() { return length;} // Set Method public void setLength(int length) { this.length = length;} @Override public double calculateRentalFee() { double fee = length * 0.02; int currYear = Calendar.getInstance().get(Calendar.YEAR); if (this.getYear() == currYear) { fee += 1;} return fee;} @Override public String toString() { return "MusicCD [id:" + this.id + " title:" + this.title + " length:" + this.getLength() + " year:" + this.year + " available:" + this.available + "]n"; } }
import java.io.File; import java.io.FileNotFoundException; import java.util.ArrayList; import java.util.List; import java.util.Scanner; import javax.swing.JOptionPane; public class Manager { static List mediaList = new ArrayList<>(); Manager() {} public boolean LoadMedia(String path) { try { File Selection = new File(path); try (Scanner File = new Scanner(Selection)) { while (File.hasNextLine()) { String data = File.nextLine(); String[] str = data.split(","); switch (str[0]) { case "EBook": mediaList.add(new EBook(Integer.parseInt(str[1]), str[2], Integer.parseInt(str[3]), Boolean.parseBoolean(str[4]), Integer.parseInt(str[5]))); break; case "MusicCD": mediaList.add(new MusicCD(Integer.parseInt(str[1]), str[2], Integer.parseInt(str[3]), Boolean.parseBoolean(str[4]), Integer.parseInt(str[5]))); break; default: mediaList.add(new MovieDVD(Integer.parseInt(str[1]), str[2], Integer.parseInt(str[3]), Boolean.parseBoolean(str[4]), Double.parseDouble(str[5]))); break;} } JOptionPane.showMessageDialog(null, mediaList);} return true;} catch (FileNotFoundException e) { JOptionPane.showMessageDialog(null, "File cannot be opened: Could not load, no such directory"); return false;} } public void findMedia(String title) { String result = ""; boolean inStock = false; for (int i = 0; i < mediaList.size(); i++) { if (mediaList.get(i).getTitle().equals(title)) { result += mediaList.get(i).toString(); System.out.print(mediaList.get(i).toString()); inStock = true;} } if (!result.isEmpty()) { JOptionPane.showMessageDialog(null, result);} if (!inStock) { JOptionPane.showMessageDialog(null, "There is no media with this title: " + title);} } public void rentMedia(int id) { boolean inStock = false; for (Media m : mediaList) { if (m.getId() == id) { inStock = true; if (m.isAvailable()) { JOptionPane.showMessageDialog(null, "Media was successfully rented. Rental Fee = $" + m.calculateRentalFee()); m.available = false;} else { JOptionPane.showMessageDialog(null, "Media with id=" + id + " is not available for rent "); break;} } } if (!inStock) { JOptionPane.showMessageDialog(null, "The media object id=" + id + " is not found ");} } }
import java.io.File; import javax.swing.*; public class MediaRentalSystem extends javax.swing.JFrame { /** * */ private static final long serialVersionUID = -50037594406635831L; public MediaRentalSystem() { initComponents(); } Manager manager = new Manager(); //Constructor private void initComponents() { jMenuBar1 = new javax.swing.JMenuBar(); jMenu3 = new javax.swing.JMenu(); jMenuItem1 = new javax.swing.JMenuItem(); jMenuItem2 = new javax.swing.JMenuItem(); jMenuItem3 = new javax.swing.JMenuItem(); jMenuItem4 = new javax.swing.JMenuItem(); setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); setTitle("Welcome To Media Rental System "); jMenu3.setText("Menu"); jMenuItem1.setText("Load Media object..."); jMenuItem1.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseClicked(java.awt.event.MouseEvent evt) { jMenuItem1MouseClicked(evt); } }); jMenuItem1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem1ActionPerformed(evt); } }); jMenu3.add(jMenuItem1); jMenuItem2.setText("Find Media object..."); jMenuItem2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem2ActionPerformed(evt); } }); jMenu3.add(jMenuItem2); jMenuItem3.setText("Rent Media object..."); jMenuItem3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem3ActionPerformed(evt); } }); jMenu3.add(jMenuItem3); jMenuItem4.setText("Quit "); jMenuItem4.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jMenuItem4ActionPerformed(evt); } }); jMenu3.add(jMenuItem4); jMenuBar1.add(jMenu3); setJMenuBar(jMenuBar1); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGap(0, 400, Short.MAX_VALUE)); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGap(0, 300, Short.MAX_VALUE)); pack(); setLocationRelativeTo(null); } private void jMenuItem1MouseClicked(java.awt.event.MouseEvent evt) { } private void jMenuItem1ActionPerformed(java.awt.event.ActionEvent evt) { JFileChooser chooser = new JFileChooser(); int fChooser = chooser.showOpenDialog(null); if (fChooser == JFileChooser.APPROVE_OPTION) { System.out.println("You chose to open this file: " + chooser.getSelectedFile().getName()); File file = chooser.getSelectedFile(); String filename = file.getName(); System.out.println("You have selected: " + filename); manager.LoadMedia(filename); } } private void jMenuItem2ActionPerformed(java.awt.event.ActionEvent evt) { String title = JOptionPane.showInputDialog(null, "Enter the title"); //CODE ADDED if (title != null) { manager.findMedia(title); } else { JOptionPane.showMessageDialog(null, "Invalid title Value"); } } private void jMenuItem3ActionPerformed(java.awt.event.ActionEvent evt) { String id = JOptionPane.showInputDialog(null, "Enter The id"); //CODE ADDED if (id != null) { manager.rentMedia(Integer.parseInt(id)); } else { JOptionPane.showMessageDialog(null, "Invalid id Value"); } } private void jMenuItem4ActionPerformed(java.awt.event.ActionEvent evt) { System.exit(0); } public static void main(String args[]) { try { for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) { if ("Nimbus".equals(info.getName())) { javax.swing.UIManager.setLookAndFeel(info.getClassName()); break; } } } catch (ClassNotFoundException ex) { java.util.logging.Logger.getLogger(MediaRentalSystem.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (InstantiationException ex) { java.util.logging.Logger.getLogger(MediaRentalSystem.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (IllegalAccessException ex) { java.util.logging.Logger.getLogger(MediaRentalSystem.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } catch (javax.swing.UnsupportedLookAndFeelException ex) { java.util.logging.Logger.getLogger(MediaRentalSystem.class.getName()).log(java.util.logging.Level.SEVERE, null, ex); } java.awt.EventQueue.invokeLater(new Runnable() { public void run() { new MediaRentalSystem().setVisible(true); } }); } private javax.swing.JMenu jMenu3; private javax.swing.JMenuBar jMenuBar1; private javax.swing.JMenuItem jMenuItem1; private javax.swing.JMenuItem jMenuItem2; private javax.swing.JMenuItem jMenuItem3; private javax.swing.JMenuItem jMenuItem4; }
I'm using the below text file
EBook,1,GhostFleet,2016,true,15 MusicCD,2,True,2013,true, 47 MovieDVD,3,PulpFiction,1994,true,4096 EBook,4,GameofThrones,1997,true,41 MusicCD,5,Burnt,2015,true,53 MovieDVD,6,FightClub,1999,true,4024
Code requirements
Design and implement Java program as follows: 1) Media hierarchy: Create Media, EBook, MovieDVD, and MusicCD classes from Week 3 -> Practice Exercise - Inheritance solution. Add an attribute to Media class to store indication when media object is rented versus available. Add code to constructor and create get and set methods as appropriate. Add any additional constructors and methods needed to support the below functionality 2) Design and implement Manager class which (Hint: check out Week 8 Reading and Writing files example): stores a list of Media objects has functionality to load Media objects from files creates/updates Media files has functionality to add new Media object to its Media list has functionality to find all media objects for a specific title and returns that list has functionality to rent Media based on id (updates rental status on media, updates file, returns rental fee) 3) Design and implement MediaRentalSystem which has the following functionality: user interface which is either menu driven through console commands or GUI buttons or menus. Look at the bottom of this project file for sample look and feel. (Hint: for command-driven menu check out Week 2: Practice Exercise - EncapsulationPlus and for GUI check out Week 8: Files in GUI example) selection to load Media files from a given directory (user supplies directory) selection to find a media object for a specific title value (user supplies title and should display to user the media information once it finds it - should find all media with that title) selection to rent a media object based on its id value (user supplies id and should display rental fee value to the user) selection to exit program 4) Program should throw and catch Java built-in and user-defined exceptions as appropriate 5) Your classes must be coded with correct encapsulation: private/protected attributes, get methods, and set methods and value validation 6) There should be appropriate polymorphism: overloading, overriding methods, and dynamic binding 7) Program should take advantage of the inheritance properties as appropriate Style and Documentation: Make sure your Java program is using the recommended style such as: Javadoc comment up front with your name as author, date, and brief purpose of the program Comments for variables and blocks of code to describe major functionality Meaningful variable names and prompts Class names are written in upper CamelCase Constants are written in All Capitals Use proper spacing and empty lines to make code human readable
Step by Step Solution
There are 3 Steps involved in it
Step: 1
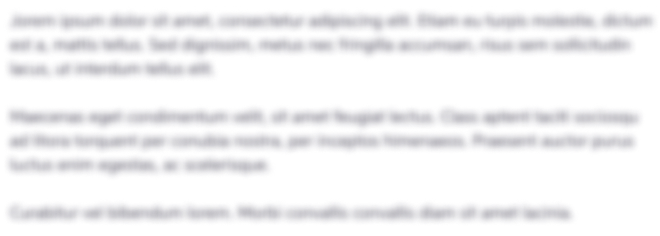
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started