Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Separated compilation: Makefile template for C++ projects: Object Oriented Approach For an optimal compilation of a general cpp project, using separated compilation, you can write
Separated compilation:
Makefile template for C++ projects: Object Oriented Approach For an optimal compilation of a general cpp project, using separated compilation, you can write a makefile script like the template below. We assume that you have splitted the project in the following modules; main.cpp, Class_01.cpp, Class_01.h, Class_02.cpp, Class_02.h, ... , Class_0n.cpp, Class_0n.h. A makefile is a text file named "makefile" with the following content; update: main main: main.o Class_01.o Class_02.o ... Class_0n.o g++ main.o Class_01.o Class_02.o ... Class_0n.o -o main main.o : main.cpp g++ -c main.cpp Class_01.o : Class_01.cpp Class_01.h g++ -c Class_01.cpp Class_02.o : Class_02.cpp Class_02.h g++ -c Class_02.cpp ... Class_0n.o : Class_0n.cpp Class_0n.h g++ -c Class_0n.cpp clean: rm -f *.o main all: rm -f *.o main make update Note 1: Identation of commands associated to each target are defined only with the tab key. Note 2: If the output in the execution of a target is a binary file, the name of the target must be the name of the file, in such a way that the target is executed only if the file associated was modified or is missing. Note 3: To avoid conflicts of class redefinition in the compilation process, you can write every class declaration inside the following conditional preprocessor directive; #ifndef CLASSNAME_H_ #define CLASSNAME_H_ class classname{ //here declaration of class members }; #endif Note 4: Regarding the implementation of Class_xx.cpp files just write the following #include "Class_xx.h" //other libraries used in the implementation of the member methods. //Implementation of member methods list of the class here below data_type Class_xx::method01("formal parameters list here"){ //Implementation of the method here } Note 5: In order to build the project, just type in the command line "make update" or just "make". To rebuild (clean and build) the project, just type "make all". If we type make without target, the target executed by default is the first target in the script, so update in this particular makefile template. Note 6: For testing the right performance of the makefile script, just change the content of any file in the project, save the changes and on command line type "make update" or just "make", and if everything is right only the modules affected by the changes will be compiled before the execution of the linking process. If no project file was changed, the linking process is not executed. In order to force the compilation of all modules, just type "make all". Makefile template for C projects: Modular Approach For an optimal compilation of a general c project based on functions and using separated compilation, you can write a makefile script like the template below. We assume that you have splitted the project in the following modules; main.c, prototypes.h, module_01.c, module_02.c, ... , module_0n.c. A makefile is a text file named "makefile" with the following content; update: main main: main.o module_01.o module_02.o ... module_0n.o gcc main.o module_01.o module_02.o ... module_n.o -o main main.o: main.c gcc -c main.c module_01.o: module_01.c module_01.h gcc -c module_01.c module_02.o: module_02.c module_02.h gcc -c module_02.c ... module_0n.o: module_0n.c module_n.h gcc -c module_n.c clean: rm -f *.o main all: rm -f *.o main make update Note 1: Identation of commands associated to each target are defined only with the tab key. Note 2: If the output in the execution of a target is a binary file, the name of the target must be the name of the file, in such a way that the target is executed only if the file associated was modified or is missing. Note 3: Regarding the implementation of *.c files just write the following #include "prototypes.h" //other libraries used in the implementation of the function. //Implementation of function here data_type func("formal parameters list here"){ //Implementation of the function here } Note 4: In order to build the project, just type in the command line "make update" or just "make". To rebuild (clean and build) the project, just type "make all". Note 5: For testing the right performance of the makefile script, just change the content of any file in the project, save the changes and on command line type "make update" or just "make", and if everything is right only the modules affected by the changes will be compiled before the execution of the linking process. If no project file was changed, the linking process is not executed. In order to force the compilation of all modules, just type "make all". Note 6: If you are interested in writing the makefile in a more general way in function of script variables, you can. The inclusion of script variable allows you to change the configuration easily just changing the value of the variable at the beginning of the script. Below we write the previous makefile script but now using script variables. #This script allows separated compilation and parametric configuration CC=g++ CFLAGS=-c -Wall CFF=-rf update: main main: main.o func_01.o func_02.o ... func_0n.o $(CC) main.o func_01.o func_02.o ... func_0n.o -o main main.o: main.cpp prototypes.h $(CC) $(CFLAGS) main.cpp func_01.o: func_01.cpp prototypes.h $(CC) $(CFLAGS) func_01.cpp func_02.o: func_02.cpp prototypes.h $(CC) $(CFLAGS) func_02.cpp ... func_0n.o: func_0n.cpp prototypes.h $(CC) $(CFLAGS) func_0n.cpp clean: rm $(CFF) *.o main all: rm $(CFF) *.o main make update
Crt.cpp:
#include #include using namespace std; const float PI=3.141592654; class Circle{ float radius; public: Circle(); void setRadius(float radius); float area(); float perimeter(); }; class Rectangle{ float base; float height; public: Rectangle(); void setBase(float); void setHeight(float); float area(); float perimeter(); }; class Triangle{ float base; float height; public: Triangle(); void setBase(float); void setHeight(float); float area(); float perimeter(); }; int main(){ Circle c; Rectangle r; Triangle t; float tmp; //Input for Circle cout>tmp; c.setRadius(tmp); //Input for Rectangle cout>tmp; r.setBase(tmp); cout>tmp; r.setHeight(tmp); //Input for Triangle cout>tmp; t.setBase(tmp); cout>tmp; t.setHeight(tmp); //Process-output coutHello32.asm:
global main ;must be declare for the linker ld section .text main: ;tells linker entry point mov edx,len ;message length mov ecx,msg ;message to write mov ebx,1 ;file descriptor (stdout) mov eax,4 ;system call number (sys_write) int 0x80 ;call kernel mov eax,1 ;system call number (sys_exit) int 0x80 ;call kernel section .data msg db 'Hello, world!', 0xa ;string to be printed len equ ($ - msg) ;length of the stringWrite a makefile for the compilation of the project crt.c. Read the document below for writing the makefile of the project crt.c in order to allow separated compilation of the modules in the project. In order to do that follow the next step 1.- Rewrite the project crt.cpp used in Lab 02 to its procedural version crt.c in a single file. 2.- Split the project crt.c in the following modules; circle.c, circle.h, rectangle.c, rectangle.h triangle.c, triangle.h and main.c 3.- Write the makefile for an efficient compilation of the project crt.c. 4.- Additionally write a makefile for assembling and linking the program hello32.asm seen in Lab 02. Concepts in practice: Unix Programming Environments, makefile. Due date: 02/14/2018(mm/dd/yyyy) Files to be used: Separated compilation crt.cpp hello32.Cpp Files to be delivered: crt.c, crt.c_project.zip, makefile_hello32. Write a makefile for the compilation of the project crt.c. Read the document below for writing the makefile of the project crt.c in order to allow separated compilation of the modules in the project. In order to do that follow the next step 1.- Rewrite the project crt.cpp used in Lab 02 to its procedural version crt.c in a single file. 2.- Split the project crt.c in the following modules; circle.c, circle.h, rectangle.c, rectangle.h triangle.c, triangle.h and main.c 3.- Write the makefile for an efficient compilation of the project crt.c. 4.- Additionally write a makefile for assembling and linking the program hello32.asm seen in Lab 02. Concepts in practice: Unix Programming Environments, makefile. Due date: 02/14/2018(mm/dd/yyyy) Files to be used: Separated compilation crt.cpp hello32.Cpp Files to be delivered: crt.c, crt.c_project.zip, makefile_hello32
Step by Step Solution
There are 3 Steps involved in it
Step: 1
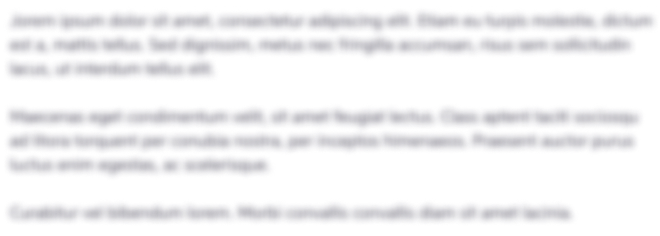
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started