Question
Set the following for loop header so that it prints the numbers, 0 2 4 6 8. for (int i = ___; i ____; i
Set the following for loop header so that it prints the numbers, 0 2 4 6 8.
for (int i = ___; i ____; i ___ ) { System.out.print(i + " "); }
2, <= 10, ++
2, < 10, += 2
0, < 10, += 2
0, <= 10, += 2
2, <= 10, += 2
________________________________
The following if statement tests the rainfall in New Yorks Central Park during the months of June, July and August:
if (low <= rain && rain <= high) { System.out.println("Rainfall amount is normal."); } else { System.out.println("Rainfall amount is abnormal."); }
Which of the following code segments would produce the exact same output?
I.
if (rain >= low) { if (rain <= high) { System.out.println("Rainfall amount is normal."); } } else { System.out.println("Rainfall amount is abnormal."); }
II.
if (rain >= low) { if (rain <= high) { System.out.println("Rainfall amount is normal."); } else { System.out.println("Rainfall amount is abnormal."); } } else { System.out.println("Rainfall amount is abnormal."); }
III.
if (rain >= low) { System.out.println("Rainfall amount is normal."); } else if (rain <= high) { System.out.println("Rainfall amount is normal."); } else { System.out.println("Rainfall amount is abnormal."); }
I, II or III
II or III
I only
III only
II only
_____________________________________________
What does the following code do?
if (month == 4) { if (day <= 21) { System.out.println("Aries"); } }
Prints a message if month is 4 or day is less than or equal to 21.
Prints a message if month is 4 or day is greater than or equal to 21.
Doesn't work - you cannot have two if statements together.
Prints a message if month is 4 and day is greater than or equal to 21.
Prints a message if month is 4 and day is less than or equal to 21.
______________________________________
What is output by the following code segment?
int x = 11; int y = 11; if (x != y ) { System.out.print("one"); } else if (x > y) { System.out.print("two"); } else if (y < x) { System.out.print("three"); } else if (y >= x) { System.out.print("four"); } else { System.out.print("five"); }
four
three
one
two
five
____________________________________________
Which option best completes the truth table for A && !B?
A | B | A && !B |
---|---|---|
1 | 1 | (_1_) |
1 | 0 | (_2_) |
0 | 1 | (_3_) |
0 | 0 | (_4_) |
(_1_) 0; (_2_) 1; (_3_) 0; (_4_) 0;
(_1_) 1; (_2_) 1; (_3_) 0; (_4_) 1;
(_1_) 1; (_2_) 0; (_3_) 0; (_4_) 0;
(_1_) 1; (_2_) 1; (_3_) 1; (_4_) 1;
(_1_) 1; (_2_) 0; (_3_) 1; (_4_) 1;
___________________________________________________
When defining a class, it is a best practice to declare ____ as private.
mutators
data
accessors
variables
______________________________
Consider the class below:
public class A { public A() { System.out.print("one"); } public A(int x) { System.out.print("two"); } }
What is output by the following?
A a = new A();
nothing
one
two
twoone
onetwo
__________________________________
Consider the complete class definition below:
public class Die { public static void rollIt() { /* Missing Code */ } }
Which of the following is the proper way to call the function rollIt() from another class?
Die d = new Die(); rollIt(d);
Die d = new Die(); d.rollIt();
Die.rollIt();
None of the above
rollIt();
_________________________
Classes use ______ to define their behavior.
constructors
references
variables
parameters
methods
_____________________________
Questions 10 12 refer to the following classes definitions:
public class Battery { private boolean fullyCharged; private int charge; private String type; public Battery (int ch, String ty) { charge = ch; if (charge == 100) { fullyCharged = true; } type = ty; } public boolean isFullyCharged() { //returns true if the Battery is fully charged, false otherwise //implementation not shown } //other methods not shown } public class Inventory { ArrayListinventory; public Inventory (ArrayList inv) { inventory = inv; } //other methods not shown }
To add a method that can count how many Battery objects in the ArrayList inventory are charged at less than 50%, which of the following is true?
The method could be implemented in Battery or Inventory.
The method should be implemented in Inventory.
The method cannot be written because the ArrayList is declared private.
The method should be implemented in Battery and Inventory.
The method should be implemented in Battery.
_____________________________________
Which accessor method could not be implemented in Battery?
getType() //returns the type of a Battery
getInventory() //returns the ArrayList of all Batteries in the inventory
getCharge() //returns the charge of a Battery
isFullyCharged() //returns true if a Battery is fully charged
equals() //returns true if the type and charge of two Batteries are the same
_____________________________________
The following method in Inventory is intended to count how many batteries are fully charged. What should replace /*Missing Code */ so that the method works as intended?
public int countFullyCharged() { int c = 0; /* Missing Code */ return c; }
-
None of the above
for (Battery b: inventory) { if (b.isFullyCharged()) { c++; } }
for (Battery b: inventory) { c++; }
if (inventory.isFullyCharged()) { c++; }
for (Battery b: inventory) { if (b.charge == 100) { c++; } }
___________________________
Methods used to change variables are called ______.
toString
accessors
void
equals
mutators
____________________
Write the header for the default constructor for a class called Ship.
private void Ship()
private Ship()
public void Ship()
public int Ship()
public Ship()
_________________________
Which of the following is the correct way to declare a static variable called x?
x;
private int x;
int x;
private static int x;
private constant int x;
__________________________
What is printed as a result of executing the following code segment?
ArrayListlist = new ArrayList (); list.add("cookies"); list.add("nachos"); list.add("chips"); list.add("trail mix"); list.add("celery"); for (String s: list) { if (s.length() > 4 && s.length() < 6) { System.out.print(s.toUpperCase() +" "); } }
NACHOS CHIPS
chips
CHIPS
nachos chips
NACHOS CHIPS CELERY
_________________________
Consider the following declaration for an ArrayList:
ArrayListlist = new ArrayList ();
After values have been added to the array, the following segment processes the ArrayList:
list.add(list.get(0)); list.remove(0);
Which of the following best describes what this segment does?
Adds the last letter in the String onto the beginning.
Move the first String in the ArrayList to the end of the ArrayList.
Does not changes the Strings in the ArrayList.
Removes the first letter in each String in the ArrayList.
Adds the first letter in the String onto the end.
____________________
You have written a program to create a grocery list. As each item is placed into your basket you call method called removeItem and it should remove the item from your list. Which of the statements about the code below are true?
public static void removeItem(ArrayListli, String remove) { for (String s: li) { if (s.equals(remove)) { li.remove(s); } } }
An exception will be thrown.
The list will have all of the instances of the word passed in as a parameter removed.
No changes are made to the ArrayList because the if (s.equals(remove)) is never true.
Nothing, changes made to object data types are not preserved after method calls.
All elements in the ArrayList are removed.
_____________________
Consider the following code segment:
ArrayListbulbs = new ArrayList (); bulbs.add(new Light()); bulbs.remove(0); bulbs.add(new Light()); Light b = new Light(); bulbs.add(1, b); bulbs.add(new Light()); bulbs.remove(0); bulbs.add(new Light()); bulbs.remove(2); bulbs.remove(1); bulbs.add(new Light());
What is the size of bulbs after running the code?
3
5
4
2
6
______________________
What goes in between the < > when instantiating a new ArrayList?
A primitive data type
A class data type
A primitive variable
A class variable
Any data type
Step by Step Solution
There are 3 Steps involved in it
Step: 1
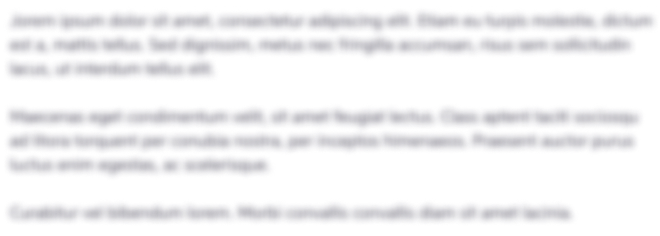
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started