Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Short Answers Short Answers Section 4.1 Pointers and Dynamic Memory Suppose that p is an int* variable. Write several lines of code that will make
Short Answers
Short Answers Section 4.1 Pointers and Dynamic Memory |
- Suppose that p is an int* variable. Write several lines of code that will make p point to an array of 100 integers, place the numbers 0 through 99 into the array components, and return the array to the heap. Do not use malloc.
- What happens if you call new but the heap is out of memory?
- Draw a picture of memory after these statements:
int i = 42; int k = 80; int* p1; int* p2; p1 = &i; p2 = &k;
- Suppose that we execute the statements from the previous question, and then we execute these statements:
*p1 = *p2;
Draw a picture of memory after this additional statement. - Draw a picture of memory after these statements:
int i = 42; int k = 80; int* p1; int* p2; p1 = &i; p2 = &k; p1 = p2;
Short Answers Section 4.2 Pointers and Arrays as Parameters - Here is a function prototype:
void exam(const double data[]);
Write two or three sentences to tell me all the information that you know about the parameter. - Consider the following prototype:
function foo (const int * p);
What restriction does the const keyword provide within the implementation of foo?Short Answers Section 4.3 The Bag ADT with a Dynamic Array - Chapter 4 provides a bag class that stores the items in a dynamic array. Use an example with pictures to explain what goes wrong if the automatic assignment operator is used for this bag.
- Consider the bag from Chapter 4 (using a dynamic array). If the insert function is activated, and the bag is full, then the bag is resized with the statement:
reserve(used + 1);
Describe a problem with this approach, and provide a better solution. - Consider the bag from Chapter 4 (using a dynamic array). The pointer to the dynamic array is called data, and the size of the dynamic array is stored in a private member variable called capacity. Write the following new member function:
void bag::triple_capacity( ) // Postcondition: The capacity of the bag's dynamic array has been // tripled. The bag still contains the same items that it previously // had.
Do not use the reserve function, do not use realloc, and do not cause a heap leak. Do make sure that both data and capacity have new values that correctly indicate the new larger array.Short Answers Section 4.6 The Polynomial - Implement functions to meet the following specificaions. In each case, you should make an appropriate choice for the kind of parameter to use (a value parameter, a reference parameter, or a const reference parameter). These functions are neither member functions nor friends.
- A. A void function with one parameter (a polynomial p). The function prints the value of p evaluated at x=1, x=2, x=3,..., up to x=10.
- B. A void function with one parameter (a polynomial p). The function deletes the largest term in p, and this change should affect the actual argument.
- This question is relevant if you implemented the polynomial class with a dynamic array. Which of the polynomial operators needed a nested loop in its implementation?
- This question is relevant if you implemented the polynomial class with a dynamic array. Draw a picture of the values in the first five elements of your dynamic array and tell me the value of the current degree after these statements:
polynomial p(3); p.assign_coef(4.1, 4); p.assign_coef(2.1, 2);
- Suppose that a main function has executed the three statements shown in the previous problem. Give an example of one statement that can now be executed by the main function, causing the degree of p to drop to 2.
- This question is relevant if you implemented a polynomial that included some calculus-based operations such as derivative and integral. Write a new function that meets the following specification:
double slope(const polynomial& p, double x) // POSTCONDITION: The return value is equal to the slope of the // polynomial p, evaluated at the point x.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
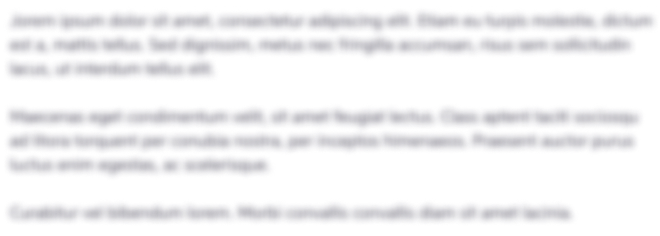
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started