Question
//************************ SLLNode.java ******************************* // node in a generic singly linked list class public class SLLNode { public T info; public SLLNode next; public SLLNode() {
//************************ SLLNode.java ******************************* // node in a generic singly linked list class
public class SLLNode
*******
package LinkedList;
//************************** SLL.java ********************************* // a generic singly linked list class
public class SLL
Q- WRITE JAVA METHOD public void insertBefore(T newElem, T existingElem) that inserts an element newElem before the element existingElem. If no existingElem exists, then the method prints existingElem does not exist and returns. If more than one instance of exisingElem exists, then the methods inserts before the first instance of existingElem.
For example, suppose your linked list (of integers) is: [ 3 5 4 2 9 ],
Then a call to insertBefore(new Integer(5), new Integer(9)) would result in the following linked list: [ 3 5 4 2 5 9 ]
A call to insertBefore(new Integer(7), new Integer(5)) would result in [ 3 7 5 4 2 5 9 ]
A call to insertBefore(new Integer(8), new Integer(10)) would result in
WARNING: Element 10 does not exist in the linked list. Insertion failed.
(b) Add the following methods with the same methodology as insertBefore:
- public void deleteBefore(T elem)
Make sure you test for cases where the list has only one element.
(c) Write a test class to test these methods.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
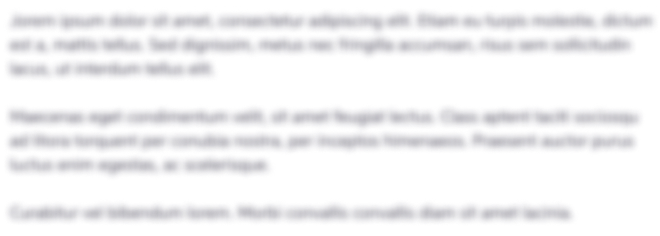
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started