Answered step by step
Verified Expert Solution
Question
1 Approved Answer
So I am able to get my sudoku to check for correct and wrong answer but how do I get the player to be able
So I am able to get my sudoku to check for correct and wrong answer but how do I get the player to be able to play the game?
public class Sudoku
Method to check if placing a number at a particular position is safe
public boolean isSafeint b int r int c int n
for int d ; d blength; d
Check if the number is already present in the row or column
if brd n bdc n
return false;
int sqt int Math.sqrtblength;
int boxRowStart r r sqt;
int boxColStart c c sqt;
Check if the number is already present in the x box
for int r boxRowStart; r boxRowStart sqt; r
for int d boxColStart; d boxColStart sqt; d
if brd n
return false;
return true;
Method to solve the Sudoku puzzle using backtracking
public boolean solveSudokuint b int num
int r ;
int c ;
boolean isVacant false;
Find the first vacant position in the puzzle
for int i ; i num; i
for int j ; j num; j
if bij
r i;
c j;
isVacant true;
break;
if isVacant
break;
If there are no vacant positions, the puzzle is solved
if isVacant
return true;
Try placing numbers from to num at the vacant position
for int no ; no num; no
if isSafeb r c no
brc no;
Recursively try to solve the rest of the puzzle
if solveSudokub num
return true;
else
If the current placement does not lead to a solution, backtrack
brc;
No valid number can be placed at the current position
return false;
Method to display the Sudoku grid
public void displayint b int n
for int i ; i n; i
for int d ; d n; d
System.out.printbid;
System.out.println;
Main method to test the Sudoku solver
public static void mainString args
Example Sudoku puzzle
int b
;
Sudoku obj new Sudoku;
int size blength;
System.out.printlnThe grid is: ;
obj.displayb size;
Solve the Sudoku puzzle and display the solution
if objsolveSudokub size
System.out.printlnThe solution of the grid is: ;
obj.displayb size;
System.out.printlnCorrect;
else
System.out.printlnThere is no solution available.";
Step by Step Solution
There are 3 Steps involved in it
Step: 1
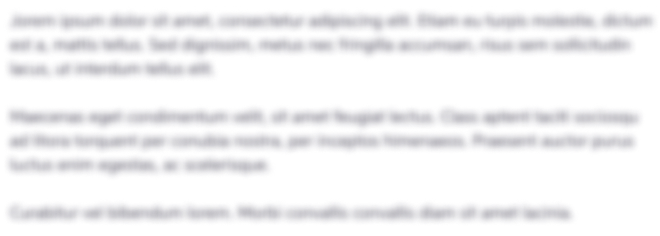
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started