Question
So I'm getting confused on how to set up my insert function to sort the numbers while in the function. I posted the output text,
So I'm getting confused on how to set up my insert function to sort the numbers while in the function. I posted the output text, so when a number is added, the print_forward and print_backwards will have the list sorted
Here is what my code is:
#ifndef LINKEDLIST_H #define LINKEDLIST_H #include#include #include using namespace std; template class LinkedList { public: LinkedList();// creates an empty list ~LinkedList(); // deletes all allocated memory void insert(const T value); // adds a value to the list bool remove(const T value); // removes a value from the list bool contains(const T value) const; // tests if value is in the list size_t length() const; // returns the number of list elements void print_forwards() const; // prints the list from head to tail void print_backwards() const; // prints the list from tail to head T getLast();// find last element recursively private: struct Node { T data; Node *prev; Node *next; }; Node *head; Node *tail; T getLast(Node* node); //recursive function to return last element }; template LinkedList ::~LinkedList() { // loop over the list deleting the nodes of list while(head != nullptr) { Node* temp = head; // set temp to head // update head to next node in list head = head->next; // deleting temp temp->next = nullptr; temp->prev = nullptr; delete(temp); temp = nullptr; } // updating tail to null tail = nullptr; } template LinkedList ::LinkedList() { // creating an empty list // by setting head and tail to null head = nullptr; tail = nullptr; } template void LinkedList ::insert(const T value) { //function to insert the value at the // end of the list // create a new node to store value Node* node = new Node(); node->data = value; node->next = nullptr; // set next of node to null (since it is the last node) node->prev = tail; // set prev of node to tail if(head == nullptr) // first node inserted, make head point to node head = node; else // not the first node, make next of tail to node tail->next = node; tail = node; // update tail to node } template bool LinkedList ::remove(const T value) { Node* curr = head; // set curr to head // loop over the list until value is found or end of list is reached while(curr != nullptr) { if(curr->data == value) // value found, exit the loop break; curr = curr->next; } // value in list, delete curr if(curr != nullptr) { if(curr == head) // curr is first node { head = head->next; // update head to next node if(head != nullptr) // non-empty list after removal, set prev of head to null head->prev = nullptr; else // empty list, set tail to null tail = nullptr; } else if(curr == tail) // curr is last node { tail = tail->prev; // update tail to previous node tail->next = nullptr; // set next of tail to null } else // middle node { // update next of node prev to curr to node next to curr curr->prev->next = curr->next; // update prev of node next to curr to node prev to curr curr->next->prev = curr->prev; } // delete the curr curr->next = nullptr; curr->prev = nullptr; delete(curr); curr = nullptr; return true; } return false; //if not found } template bool LinkedList ::contains(const T value) const { Node* curr = head; // set curr to head // this will loop over the list to search for value or until // end of list is reached while(curr != nullptr) { if(curr->data == value) // value found return true; curr = curr->next; } return false; //return if value was not found } template size_t LinkedList ::length() const { size_t count = 0; // initialize count to 0 Node* curr = head; // set curr to head // loop over the list, incrementing count by 1 // until end of list is reached while(curr != nullptr) { count++; curr = curr->next; } return count; } template void LinkedList ::print_forwards() const { Node* curr = tail; // set curr to tail // loop to display values of list separated by space // moving backward in list while(curr != nullptr) { cout< data; if(curr != head) cout<<" "; curr = curr->prev; } cout << endl; } template void LinkedList ::print_backwards() const { Node* curr = head; // set curr to head // loop to display values of list separated by a space moving // forward in list while(curr != nullptr) { cout< data; if(curr != tail) cout <<" "; curr = curr->next; } cout << endl; } template T LinkedList ::getLast() { return getLast(head); // call recursive function passing // head of the list } template T LinkedList :: getLast(Node* node) { if(node == nullptr) // empty list, return default value for T return T(); else if(node == tail) // tail node reached, return value return node->data; // at node else // tail node not reached, call getLast passing next node return getLast(node->next); } #endif LINKEDLIST_H
Here is the test.cpp
#include#include #include #include "LinkedList.h" using namespace std; int main() { LinkedList ll; for (int i = 0; i < 10; i++) { ll.insert(i); } cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "rm 5 " << ll.remove(5) << endl; cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "rm 0 " << ll.remove(0) << endl; cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "rm 9 " << ll.remove(9) << endl; cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); for (int i = 0; i < 10; i++) { cout << "rm " << i << " " << ll.remove(i) << endl; } cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); for (int i = 9; i >= 0; i--) { ll.insert(i); } cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "add -1 " << endl; ll.insert(-1); cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "add 100 " << endl; ll.insert(100); cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "add 4 " << endl; ll.insert(4); cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "rm 4 " << ll.remove(4) << endl; cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "rm 4 " << ll.remove(4) << endl; cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "rm 4 " << ll.remove(4) << endl; cout << ll.length() << endl; ll.print_forwards(); ll.print_backwards(); cout << "last elem "<< ll.getLast() < *pll = new LinkedList ; for (int i = 0; i < 10000; i++) { pll->insert(i*17.4); } delete pll; pll = NULL; }
This is what the output should look like:
10 0 1 2 3 4 5 6 7 8 9 9 8 7 6 5 4 3 2 1 0 rm 5 1 9 0 1 2 3 4 6 7 8 9 9 8 7 6 4 3 2 1 0 rm 0 1 8 1 2 3 4 6 7 8 9 9 8 7 6 4 3 2 1 rm 9 1 7 1 2 3 4 6 7 8 8 7 6 4 3 2 1 rm 0 0 rm 1 1 rm 2 1 rm 3 1 rm 4 1 rm 5 0 rm 6 1 rm 7 1 rm 8 1 rm 9 0 0
10 0 1 2 3 4 5 6 7 8 9 9 8 7 6 5 4 3 2 1 0 add -1 11 -1 0 1 2 3 4 5 6 7 8 9 9 8 7 6 5 4 3 2 1 0 -1 add 100 12 -1 0 1 2 3 4 5 6 7 8 9 100 100 9 8 7 6 5 4 3 2 1 0 -1 add 4 13 -1 0 1 2 3 4 4 5 6 7 8 9 100 100 9 8 7 6 5 4 4 3 2 1 0 -1 rm 4 1 12 -1 0 1 2 3 4 5 6 7 8 9 100 100 9 8 7 6 5 4 3 2 1 0 -1 rm 4 1 11 -1 0 1 2 3 5 6 7 8 9 100 100 9 8 7 6 5 3 2 1 0 -1 rm 4 0 11 -1 0 1 2 3 5 6 7 8 9 100 100 9 8 7 6 5 3 2 1 0 -1 last elem 100
Step by Step Solution
There are 3 Steps involved in it
Step: 1
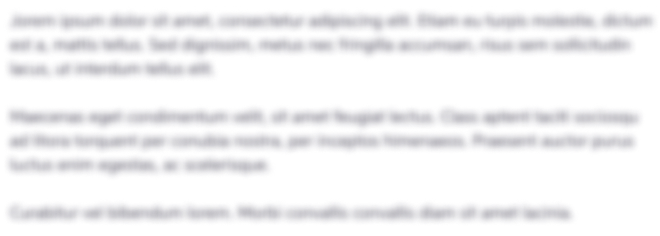
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started