Question
So, working on code and files I've been helped with and I'm struggling to figure out where I should be using System.out.printf( %.1f ); or
So, working on code and files I've been helped with and I'm struggling to figure out where I should be using System.out.printf( %.1f ); or such in order for the results to be printed out with only the first decimal rounded up. I can't mess with Main, DataSet, or CSVReader so please do not add anything there.
Given the following CSV file
1,2,3,4,5,6,7 10,20,30,40,50,60 10.1,12.2,13.3,11.1,14.4,15.5
The output of the provided main is:
Dataset Results (Method: AVERAGE) Row 1: 4.0 Row 2: 35.0 Row 3: 12.8 Dataset Results (Method: MIN) Row 1: 1.0 Row 2: 10.0 Row 3: 10.1 Dataset Results (Method: MAX) Row 1: 7.0 Row 2: 60.0 Row 3: 15.5
Note: the extra line between Set results is optional. All other spacing must be exact!
CSVReader.java
import java.io.File; import java.io.IOException; import java.util.ArrayList; import java.util.List; import java.util.Scanner; /** * This is a slightly more advanced CSV reader that can handle quoted tokens. */ public class CSVReader { private static final char DELIMINATOR = ','; private Scanner fileScanner; /** * Basic constructor that assumes the first line should be skipped. * @param file name of file to read */ public CSVReader(String file) { this(file, true); } /** * A constructor that requires the name of the file to open * @param file filename to read * @param skipHeader true to skip header, false if it is to be read */ public CSVReader(String file, boolean skipHeader) { try { fileScanner = new Scanner(new File(file)); if(skipHeader) this.getNext(); }catch (IOException io) { System.err.println(io.getMessage()); System.exit(1); } } /** * Reads a line (nextLine()) and splits it into a String array by the DELIMINATOR, if the line is * empty it will also return null. This is the proper way to check for CSV files as compared * to string.split - as it allows for "quoted" strings ,",",. * @return a String List if a line exits or null if not */ public List
DataSet.java
import java.util.ArrayList; import java.util.List; /** * A simple container that holds a set of Data, by row/col (matrix) * @version 20200627 */ public class DataSet { private final List
> data = new ArrayList<>(); /** * Reads the file, assuming it is a CSV file * @param fileName name of file */ public DataSet(String fileName) { this(new CSVReader(fileName, false)); } /** * Takes in a CSVReader to load the dataset * @param csvReader a csvReader */ public DataSet(CSVReader csvReader) { loadData(csvReader); } /** * Returns the number of rows in the data set * @return number of rows */ public int rowCount() { return data.size(); } /** * Gets a specific row based on the index. Throws exception if out of bounds * @param i the index of the row * @return the row as a List of doubles */ public List
Main.java
*/ public class Main { public static void main(String[] args) { String testFile = "sample.csv"; DataSet set = new DataSet(testFile); AverageDataCalc averages = new AverageDataCalc(set); System.out.println(averages); MinimumDataCalc minimum = new MinimumDataCalc(set); System.out.println(minimum); MaximumDataCalc max = new MaximumDataCalc(set); System.out.println(max); } }
AbstractData.java
import java.util.List; public abstract class AbstractDataCalc { protected DataSet dataSet; public AbstractDataCalc(DataSet set) { setAndRun(set); } public void setAndRun(DataSet set) { this.dataSet = set; if (set != null) { runCalculations(); } } private void runCalculations() { // Build an array (or list) of doubles with an item for each row in the dataset. // The item is the result returned from calcLine. } @Override public String toString() { StringBuilder result = new StringBuilder("Dataset Results (Method: " + getType() + ") "); for (int i = 0; i < dataSet.rowCount(); i++) { result.append("Row ").append(i + 1).append(": ").append(calcLine(dataSet.getRow(i))).append(" "); } return result.toString(); } public abstract String getType(); public abstract double calcLine(List line); }
AverageData.java
import java.util.List; public abstract class AbstractDataCalc { protected DataSet dataSet; public AbstractDataCalc(DataSet set) { setAndRun(set); } public void setAndRun(DataSet set) { this.dataSet = set; if (set != null) { runCalculations(); } } private void runCalculations() { // Build an array (or list) of doubles with an item for each row in the dataset. // The item is the result returned from calcLine. } @Override public String toString() { StringBuilder result = new StringBuilder("Dataset Results (Method: " + getType() + ") "); for (int i = 0; i < dataSet.rowCount(); i++) { result.append("Row ").append(i + 1).append(": ").append(calcLine(dataSet.getRow(i))).append(" "); } return result.toString(); } public abstract String getType(); public abstract double calcLine(List line); }
MaximumDataCalc.java
import java.util.List; public abstract class AbstractDataCalc { protected DataSet dataSet; public AbstractDataCalc(DataSet set) { setAndRun(set); } public void setAndRun(DataSet set) { this.dataSet = set; if (set != null) { runCalculations(); } } private void runCalculations() { // Build an array (or list) of doubles with an item for each row in the dataset. // The item is the result returned from calcLine. } @Override public String toString() { StringBuilder result = new StringBuilder("Dataset Results (Method: " + getType() + ") "); for (int i = 0; i < dataSet.rowCount(); i++) { result.append("Row ").append(i + 1).append(": ").append(calcLine(dataSet.getRow(i))).append(" "); } return result.toString(); } public abstract String getType(); public abstract double calcLine(List line); }
MinimumDataCalc.java
import java.util.List; public abstract class AbstractDataCalc { protected DataSet dataSet; public AbstractDataCalc(DataSet set) { setAndRun(set); } public void setAndRun(DataSet set) { this.dataSet = set; if (set != null) { runCalculations(); } } private void runCalculations() { // Build an array (or list) of doubles with an item for each row in the dataset. // The item is the result returned from calcLine. } @Override public String toString() { StringBuilder result = new StringBuilder("Dataset Results (Method: " + getType() + ") "); for (int i = 0; i < dataSet.rowCount(); i++) { result.append("Row ").append(i + 1).append(": ").append(calcLine(dataSet.getRow(i))).append(" "); } return result.toString(); } public abstract String getType(); public abstract double calcLine(List line); }
The output has been:
Dataset Results (Method: AVERAGE) Row 1: 4.0 Row 2: 35.0 Row 3: 12.766666666666666 Dataset Results (Method: MIN) Row 1: 1.0 Row 2: 10.0 Row 3: 10.1 Dataset Results (Method: MAX) Row 1: 7.0 Row 2: 60.0 Row 3: 15.5
I would like not only Row 3 of Average to round correctly, but all of them and I can't mess with Main, DataSet, or CSVReader so please do not add anything there
Step by Step Solution
There are 3 Steps involved in it
Step: 1
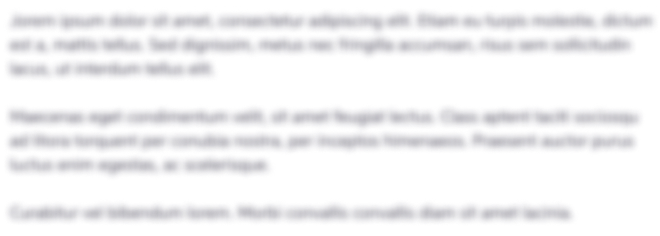
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started