Solution Description: For this assignment, create files with the following names: Superhero.java ChargedSuperhero.java FlyingSuperhero.java Creating a Superhero 1.Create a class called Superhero that has the
Solution Description:
For this assignment, create files with the following names:
•Superhero.java
•ChargedSuperhero.java
•FlyingSuperhero.java
Creating a Superhero
1.Create a class called Superhero that has the following instance data:
a. name: All superheroes have a code name! This protected variable should be called name and it should be a String.
b. health: superheroes have health so they should have a set amount of health points. Their health can only be represented through whole numbers. This variable should be protected.
c. damage: superheroes do a set amount of attack damage in each attack! (More on this later) This attack can only be represented through whole numbers. This variable should be protected.
2.Superhero should have the following constructors:
a. Include a no-argument constructor that sets the following values
•name: “Anonymous Superhero”
•health:100
•damage: 8
b .Include a constructor that takes in name, health, and damage values in this order.
c. Make sure the constructor parameters have the same type as their corresponding fields.
d. NOTE: Constructor chaining MUST be used where possible.
3.Superhero should have the following instance methods: Below you are given the method signatures and specification for the Superhero class. Make sure these are instance methods and the signatures match exactly.
a. toStringmethod
•Must properly override Object’s toString() method.
•Should return:
SUPERHERO
Name:{name}
Health: {health}
Strength: {damage}
•Hint: Use new line characters and don’t include the brackets!
b. equalsmethod.
•Should override Object’s equals()method.
•Two superheroes are equal if they have the same name, health, and damage.
c. attack(Superhero other)method:
•Deals damage to the superhero that is taken in as a parameter.
•Only deals damage if the attacking superhero has more than 0 health.
•The amount of damage dealt to the other superhero should be equal to damage.
•Other’s health should go down by the value of damage (this Superhero’s damage, not the
other Superhero’s).
•Their health should not go below 0 because of the damage, if it does, set it to 0.
•This method should return nothing.
Creating a ChargedSuperhero
This class is a child of Superhero and should inherit from it. An example of this kind of superhero is Black Panther.
1.Create a class called ChargedSuperhero that has the following instance data:
a. charged: ChargedSuperheroes have a unique suit that allows them to charge energy and unleash a burst
of energy. Create a private variable that represents whether their suit is charged up or not named charged.
2.ChargedSuperheroshould have the following constructors:
a. Include a constructor that takes in name, health, damage, and charged in this order.
b. Include a default no-arguments constructor that creates a ChargedSuperhero with the following default
values:
•name is “Anonymous ChargedSuperhero",
•health is 30,
•damage is 10,
•charged is false.
•Use constructor chaining to call the other constructor in this class with default values.
c. Ensure that you are calling the parent constructor appropriately.
3.ChargedSuperhero should have the following instance methods:
a.toStringmethod
•Must properly override Object’s toString() method.
•Should return:
SUPERHERO
Name: {name}
Health: {health}
Strength: {damage}
Suit Charged: {charged}
•Must utilize Superhero’s toString()method to optimize code.
b. equals()method
•Should override Object’s equals()method.
•Two ChargedSuperhero are equal if they have the same name, health, damage, and
charged values.
•Must reuse code using Superhero’s equals() method.
c. attack(Superhero other)method:
•Overrides the Superhero attack method.
•Deals damage to the superhero that is taken in as a parameter.
•Only deals damage if the attacking superhero has more than 0 health.
•If the suit of the ChargedSuperhero that called this method is charged, the ChargedSuperhero will
deal damage equal to twice the amount of the value of damage(this ChargedSuperhero’s
damage, not the other Superhero’s).
•If the suit of the ChargedSuperhero is NOT charge d(charged equals false), deal damage equal to the value of damage(this ChargedSuperhero’s damage, not the other Superhero’s).
•The defending superhero’s health should not go below 0. If it does, set it back to 0.
Creating a FlyingSuperhero
This class is a child of Superhero and should inherit from it. An example of this kind of superhero is Captain Marvel.
4.Create a class called FlyingSuperhero that has the following instance data:
a. flying: a FlyingSuperhero has a unique suit that allows them to fly. Create a private variable that represents whether they are in flight or not called flying
5.FlyingSuperhero should have the following constructors:
a. Include a constructor that takes in name, health, damage, and flying in this order.
b .Include a default no-arguments constructor that creates a FlyingSuperhero with the following default values:
•name is “Anonymous FlyingSuperhero",
•health is 40,
•damage is 7,
•flying is false.
•Use constructor chaining to call the other constructor in this class with default values.
c .Ensure that you are calling the parent constructor appropriately.
6.FlyingSuperheroshould have the following instance methods:
a. toStringmethod
•Must properly override Object’s toString() method.
•Should return:
SUPERHERO
Name: {name}
Health: {health}
Strength: {damage}
Flying: {flying}
•Must utilize Superhero’s toString()method to optimize code.
b. equals()method
•Should override Object’s equals()method.
•Two FlyingSuperhero are equal if they have the same name, health, damage, and flying values.
•Must reuse code using Superhero’s equals()method.
c. attack(Superhero other)method:
•Overrides the Superhero’s attack method.
•Deals damage to the superhero that is taken in as a parameter.
•Only deals damage if the attacking superhero has more than 0 health.
•If FlyingSuperhero is flying, there is a 50% chance that FlyingSuperhero will deal damage equal to 3x the amount of the value of damage. If chance fails, deal damage equal to the value of damage(in both cases, use this FlyingSuperhero’s damage, not the other Superhero’s). o
HINT: Use Random or Math methods to calculate this chance.(HINT: there is a nextBoolean method in the Random class but you can also use numbers)
•If FlyingSuperhero is not flying, deal damage equal to the value of damage
.•The defending superhero’s health should not go below 0. If it does, set it back to 0.
NOTES & HINTS:
•You must use the exact method signatures provided
.•If we did not explicitly specify what a method should return you can assume it returns nothing
•Make sure that you call any parent constructors super.
•Make sure that you are overriding methods correctly and reusing code when possible.
•When overriding a method, if you use the @Override tag, you do NOT need to javadoc that method (javadocs NOT required for this assignment, but feel free to practice using them)!
•Unless specified, you are free to choose access modifiers for your variables. Just ensure they follow the rules of encapsulation! And, add any necessary getters and setters.
•Test your code often! Write you own driver class with a main method that creates objects with different constructors and calls methods on them
Step by Step Solution
3.44 Rating (167 Votes )
There are 3 Steps involved in it
Step: 1
Please refer the below code implemented as per the directions given in the question SuperHerojava import javautilScanner public class SuperHero private static int numberOfHeroes private String heroNam...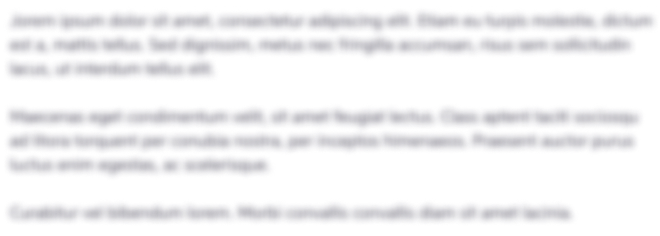
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started