Question
Solve the Towers of Hanoi problem in C++ (described in Shaffer pages 36-38) with 7 disks. Your solution should utilize a stack data structure (like
Solve the "Towers of Hanoi" problem in C++ (described in Shaffer pages 36-38) with 7 disks.
Your solution should utilize a stack data structure (like vector) and be recursive. Implement a function printPeg() to display the contents of the 3 pegs before and after the recursive solution has executed.
Implement a recursive function hanoi() to solve the puzzle. hanoi() should return the number of moves taken for the solution. Your program should output the number of moves to solve the problem with 7 disks.
Implement a function moveDisk() to move a single disk from one peg to another (the moveDisk() function will be called by hanoi()). Only use pop and push like operations to manipulate your stacks. Use assertions to enforce the rule that a larger disk can not be placed over a smaller disk. Output each move as it is being taken.
This is the output and the pegs are also suppose to be printed. PLEASE HELP!
Starting condition of the three pegs: Peg1 has 3 discs: 321 Peg2 has 0 discs: Peg3 has e discs: Moves required to move 3 discs from Pegi to Peg3: Move disc 1 from Pegi to Peg3 Move disc 2 from Peg1 to Peg2 Move disc 1 from Peg3 to Peg2 Move disc 3 from Pegi to Peg3 Move disc 1 from Peg2 to Peg1 Move disc 2 from Peg2 to Peg3 Move disc 1 from Peg1 to Peg3 Ending condition of the three pegs: Peg1 has e discs: Peg2 has e discs: Peg3 has 3 discs: 321 A stack of 3 discs can be transferred in 7 movesStep by Step Solution
There are 3 Steps involved in it
Step: 1
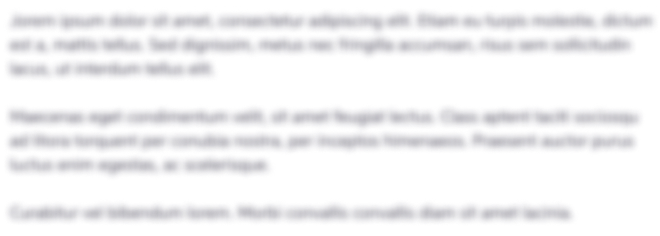
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started