Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Solve using Python ................... import random ##################################### # HELPER FUNCTIONS TO HELP PLAY THE # GAME def generate_deck(): (None) -> [suit, number], [suit, number], ...]
Solve using Python ...................
import random ##################################### # HELPER FUNCTIONS TO HELP PLAY THE # GAME def generate_deck(): (None) -> [suit, number], [suit, number], ...] Create a standard deck of cards with which to play Suits are: spades, clubs, diamonds, hearts Numbers are: 1 -13 where the numbers represent the 1 - Ace 11 - Jack 12 - Queen 13 - King 2-10 - Number cards cards = [] suits = ['spades', 'clubs', 'diamonds', 'hearts'] for suit in suits: for number in range(1,14): cards.append([suit,number]) return cards def shuffle(deck): (list) -> list Produce a shuffled version of the deck of cards Note, this function should return a new list containing the shuffled deck and not directly reorder the elements in the input list. That is, the list contained in 'deck' should be unchanged after the function returns. shuffled_deck = random sample(deck, len(deck)) return shuffled_deck #################################################### # PART 1 - Complete the following helper functions that will be used to create our game def deal_card(deck, hand): (list, list) -> None def score_hand(hand): (list) -> int Calculate the score of the player's hand. Points for each card are calculated as follows: Face Cards (jack, queen, king) = 10 points Number Cards = face value (i.e. a 2 would be worth 2 points, a 6 would be worth 6 points) Ace = Either 1 or 11 points depending what gives the hand a higher score without going over 21 ## TODO YOUR CODE HERE ############################################### # Part 2 - Write the Function that executes the game using the helper functions def play(shuffled_deck): I H11 (list) -> list Play our game and return a list containing the following elements: winner - str indicating the winner, either "player" or "dealer" winner_hand - list containing the cards in the winner's hand loser_hand - list containing the cards in the loser's hand # define the player and dealer hands player_hand = [] dealer_hand = [] ## TODO YOUR CODE HERE Problem For this lab you will complete a program to play a fictional two-player card game between a player and dealer. The game is played with a standard 52-card deck. During the game, the player and dealer are both dealt' cards from the deck according to the rules (see below). The player that has the hand with the most points, without exceeding 21, at the end of the game is the winner. For this lab, you will need to complete 3 functions: deal_card (deck, hand) score_hand (hand) play (shuffled_deck) You will be provided two additional functions to help you complete the lab: generate_deck() shuffle (deck) You do not need to edit these functions. They are provided to help you test and use your code. generate deck will create and return a list representing a standard 52-card deck. Each element of the returned list will be a two-element list. The first element containing a string representing the suit (spades, clubs, diamonds, or hearts) and the second element will be an integer between 1 and 13 inclusive, representing the card value. Values 2-10 represent number cards, 1 represents an ace, 11 a jack, 12 a queen, and 13 a king. Calling the shuffle function will randomly sort and return the list of cards. We'll break down how to complete the different functions in the steps below. Part 1: Deal Card Both deal_card and score_hand are helper functions that you will call from within play to execute the game. deal_card accepts two lists as arguments. The lists represent the game's deck of cards and one of the player's hand. The function should remove the first card from the deck list and append it to list representing the player's hand. The function modifies these lists in place and returns None. That is, this function only changes to number of elements in each input list. Consider the following code snippet to understand the behaviour of the function. deck = [['spades', 10), ('hearts',2],['clubs', 811 # deck with 3 cards player_hand = [['diamonds', 3]) # list representing a player's hand, currently with a single card print("Deck and hand before deal_hand function call") print("\tdeck : ", deck) Dealing a card refers to the act of removing the card currently at the top of the deck and assigning it to one player's hand. 2 A player's hand is the collection of cards dealt to them during the game print("\thand : ",player_hand) deal card (deck,player hand) print(" Deck and hand after deal hand function call") print("\tdeck : ", deck) print("\thand : ",player hand) Printed Output Deck and hand before deal hand function call deck : [['spades', 10), ('hearts', 2], ['clubs', 8]] hand : [['diamonds', 3]] Deck and hand after deal hand function call deck : [['hearts', 2], ['clubs', 8]] hand : [['diamonds', 3), ('spades', 101] Notice that after the function call, the first element from the deck (the 10 of spades) list has been removed and has been appended to the end of the hand list. You may assume that the deck list will always have a minimum of one element. Part 2: Score Hand Is this part, you'll complete the function score_hand (hand) which calculates the score for list of cards in a player's hand. The object of the game is to achieve a higher score than the other player without exceeding a score of 21. The scoring of a hand for our game is the same as the game of Blackjack. That is, numbered cards (2-10) are worth their numerical value (e.g. a 4 is worth 4 points), face cards (jack, queen, and king) are each worth 10 points, and aces can be worth either 1 or 11 points, set depending on what gives a higher score without exceeding 21. The sample inputs below are provided with details of the score breakdown for further clarification. Sample Inputs Example 1 >>> hand = [['spades',7],['clubs', 13], ['spades', 9]] >>> score_hand (hand) 26 Explanation: here the 7 and 9 are worth their numeric values and the king (13) is worth 10 points. So 7 + 9 + 10 = 26 Example 2 >>> hand = [['clubs',8], ['clubs',6], ['spades',1]] >>> score_hand (hand) 15 Explanation: here the 8 and 6 are worth their numeric values and the ace is worth 1 point. It is worth 1 point rather than 11 because 8 + 6 + 11 > 21. That is, assigning the ace a value of 11 would cause the score to be greater than 21. So, the score is 8 + 6 +1 = 15. Example 3 >>> hand = [['spades',2], ['clubs',1],['spades',1],['hearts',5] >>> score hand (hand) 19 Explanation: In this scenario, there are three potential scores because of the presence of two aces. These scenarios are: count both aces as 11, 2 + 11 + 11 +5 = 29 > 21 count one ace as 11, 2+ 11 +1 +5 = 19 21 In this case, the function returns 19 because it is the highest score without exceeding 21. Part 3: Implement the Game For the final part of the lab, you will need to complete the play (shuffled_deck). This function should utilize both the functions you completed in parts 1 and 2. The rules for playing the game are as follows: 1. Deal 4 cards. Both the player and dealer get 2 cards to start. The player receives the first and third card, the dealer receives the second and fourth card. 2. If the player's hand is worth less than 15 points, deal the player another card. Otherwise, skip to step 4. 3. If the player's score now exceeds 21, they automatically lose, and the game is over. 4. If the dealer has fewer than 3 cards in their hand, deal the dealer another card. Otherwise skip to step 6. 5. If the dealer's score now exceeds 21, they automatically lose, and the game is over. 6. If the player's score is less than 15 or the dealer has fewer than 3 cards in their hand, go back to step 2. 7. The player wins if their hand's score is greater than the dealer's hand's score. Otherwise the dealer wins. The function will return a three-element list. The first element will be a string identifying the winner of the game (either player" or "dealer"). The second element will be the list containing the hand of the winner and the third element will be the list containing the hand of the loser. Remember to complete a flow chart illustrating execution of this function in your engineering notebook for a mark. You can use the four basic flowchart symbols described here to complete your diagram. You're flow chart should the decisions and high-level actions (such as calling a helper function) of your program. Example deck = generate deck() deck = shuffle (deck) print("deck: ", deck[:10]) # just print the first cards in the deck result = play (deck) print("result: ", result) Below are example outputs generated by running the above code 4 different times with different randomly generated decks. Example Output 1: deck: [['diamonds', 7], ['hearts', 6], ['clubs', 8], ['spades', 5], ['spades', 1], ['hearts', 1], ['spades', 6), ('diamonds', 3], ['spades', 10), ('hearts', 11]] result: ['player', [['diamonds', 7], ['clubs', 8]], [['hearts', 6), ['spades', 5), ('spades', 1]]] Example Output 2: deck: [['spades', 1], ['hearts', 8], ['spades', 8], ['clubs', 13], ['hearts', 13], ['diamonds', 12], ['spades', 11), ['diamonds', 7], ['diamonds', 10], ['diamonds', 8]] result: ['player', [['spades', 1], ['spades', 8]], [['hearts', 8], ['clubs', 13), ('hearts', 13]]] Example Output 3: deck: [['clubs', 1], ['hearts', 8], ['diamonds', 8), ('hearts', 12], ['spades', 2], ['spades', 8], ['clubs', 4], ['diamonds', 12], ['diamonds', 2], ['clubs', 5]] result: ['dealer', [['hearts', 8], ['hearts', 12], ['spades', 2]], [['clubs', 1], ['diamonds', 8]]] Example Output 4 deck: [['hearts', 3], ['diamonds', 11], ['spades', 12), ['spades', 9), ('hearts', 71, ['hearts', 1], ['diamonds', 12), ['clubs', 3], ['diamonds', 4], ['clubs', 10]] result: ['dealer', [['diamonds', 11], ['spades', 9), ('hearts', 1]], [['hearts', 3), ('spades', 12), ('hearts', 711) Tips for developing test cases and testing part 3 Because the above code snippet uses a randomly generated deck to test the play function, it is not ideal for debugging your code when you do find an error. This is because the code won't produce the same deck multiple times in a row and therefore may not result in the same error. There are two potential options to overcome this and test your code using consistent decks. 1. Hardcode your own ~10 card deck and pass this to the play function rather than using the generate_deck and shuffle functions. This will let you develop explicit test cases to ensure your function behaves properly. 2. You can use utilize the seed function from the random module with different input arguments. If you call this function prior to calling the shuffle function, shuffle will always return the same randomly shuffled deck. You can generate different random decks by changing the input argument to the seed function. It is recommended that you use approach 1 to develop a few specific test cases first. After testing these test cases, you can use approach 2 to further test your code. Examples of both approaches are given below. Example of test approach 1 - Hardcode a deck deck = [['diamonds', 7), ('hearts', 6], ['clubs', 8], ['spades', 51, ['spades', 1), ('hearts', 1], ['spades', 6], ['diamonds', 3], [' spades', 10), ['hearts', 111] print("deck: ", deck[:10]) # just print the first cards in the deck result = play (deck) print("result: ", result) Example of test approach 2 - Use seed to generate consistent deck random.seed (3) # change '3' to different integers to produce different shuffled deck deck = generate deck () deck = shuffle (deck) print("deck: ", deck[:10]) # just print the first cards in the deck result = play (deck) print("result: ", result) IMPORTANT: Do not change the file name or function names. Do not use input() or print () inside the functions. Five test cases are provided on MarkUs. You should test your code before your final submission. These test cases will be used in grading with an additional five test casesStep by Step Solution
There are 3 Steps involved in it
Step: 1
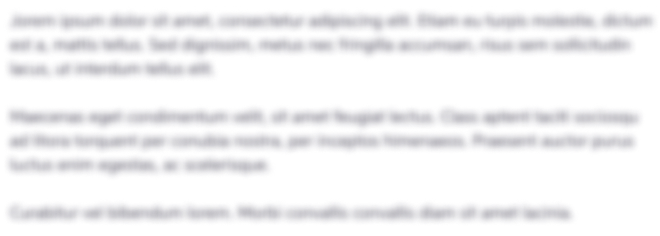
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started