Question
Sort Student array in increasing order of classification (1-freshman, 2-sophomore, 3-junior, 4-senior). If classifications are the same, name with lower alphabetical order should be first.
Sort Student array in increasing order of classification (1-freshman, 2-sophomore, 3-junior, 4-senior). If classifications are the same, name with lower alphabetical order should be first.
TODOs: (1) Make Student class a Comparable class (2) Add code in Main.java to sort.
Your program should display the following:
Student: Zac 1 Student: Edward 2 Student: Heather 2 Student: Linda 3 Student: Aaron 4 Student: Jane 4
Grading:
Making Student Comparable
- method header 5
- compare by classification 5
- compare by name 5
main method
- sort 10
/** * @author YOUR NAME HERE */
import java.util.ArrayList; import java.util.Arrays; import java.util.Collections; import java.util.Comparator;
public class Main { public static void main(String[] args) { Student[] array = new Student[6]; array[0] = new Student("Linda", 3); array[1] = new Student("Aaron", 4); array[2] = new Student("Jane", 4); array[3] = new Student("Zac", 1); array[4] = new Student("Edward", 3); array[5] = new Student("Heather", 3); //TODO: ------- Add Code Below to sort the array
// -------------Your code ends here-------- System.out.println("The students in the order of GPA and then of name:"); for (Student x: array) { System.out.println(x); }
} }
/* TODO 1: Make the class Comparable */ public class Student { private String name; private int classification;
public Student(String name, int classification) { this.name = name; this.classification = classification; } public String getName() { return name; }
public int getClassification() { return classification; }
@Override public String toString() { return String.format("Student: %s %d", name, classification); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
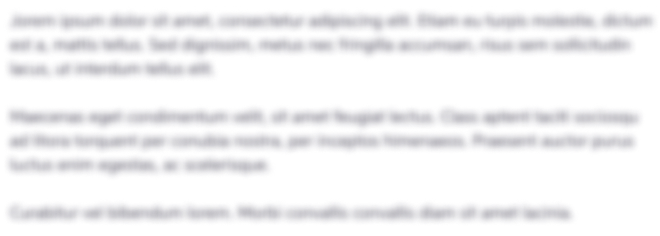
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started