Question
Source: https://github.com/Seneca-244200/OOP-Workshops/tree/main/WS09 PART 1 (100%) The Person class and Utils module (fully implemented) The Person class encapsulates a person with first, middle and last name
Source: https://github.com/Seneca-244200/OOP-Workshops/tree/main/WS09
PART 1 (100%)
The Person class and Utils module (fully implemented)
The Person class encapsulates a person with first, middle and last name and is instantiated in an empty state.
All the name fields are stored dynamically and are read from istream through comma-separated data entry.
The C-string manipulation and dynamic memory allocation logic are provided by the Utils module.
A Person is valid if it has valid first and last names. The middle name is optional, therefore the data entry for a Person can be done in the following two ways:
1- A Person with a middle name Data entry:
Homer,Jay,Simpson
Displayed as follows:
Homer Jay Simpson
2- A Person without a middle name Data entry:
Lisa,,Simpson
Displayed as follows
Lisa Simpson
Walk through the Person class using the nameTester.cpp program; study and understand it.
The Contact class
Create a Class Module called Contact, derived from the Person class. A contact is a person with an address.
The Contact class stores the address in four attributes:
- Address (Dynamic, unknown length)
- City (Dynamic, unknown length)
- Province: stored by two characters (for the province code, like ON, AL, BC, etc...)
- Postal code: stored in six characters.
Like a Person, Contact is instantiated in an empty state and later is populated from istream using comma-separated values. All the attributes defined in Contact are mandatory and can not be empty or not provided.
A Contact is read from istream as follows:
Homer,Jay,Simpson 70 the pond road,North York,ON,M3J3M6 OR Homer,,Simpson 70 the pond road,North York,ON,M3J3M6
And it is displayed as follows:
Homer Jay Simpson 70 the pond road North York ON M3J 3M6 OR Homer Simpson 70 the pond road North York ON M3J 3M6
If any data other than the middle name is missing or exceeds the field length, the Contact should be put in an invalid state.
Displaying an invalid Contact object will be quietly ignored and no action will be taken.
the Contact class implementation
- Initialize all the attributes to their default empty states in the class declaration. (as a result your defualt constructor should be empty)
- Use the Utils functions for your C-string work and dynamic memory allocation. (do not include
) - Invoke the base class's constructors and Methods in copy construction and copy assignment to make sure the base class's resources are managed properly.
- Override all the virtual functions and virtual operators of the Person class and implement the rule of three.
- Apart from the overridden methods and operators, you can create any additional method to help you implement this class.
Tester program
/*********************************************************************** // OOP244 Workshop 9: // File w9_tester.cpp // Version 1.0 // Date 2021/11/19 // Author Fardad Soleimanloo // Description // // Revision History // ----------------------------------------------------------- // Name Date Reason ///////////////////////////////////////////////////////////////// ***********************************************************************/ #include
output
Empty Contact: >< Enter the following: Homer,Jay,Simpson 70 the pond road,North York,ON,M3J3M6 Name and address > Homer,Jay,Simpson 70 the pond road,North York,ON,M3J3M6 OK! Contact: Homer Jay Simpson 70 the pond road North York ON M3J 3M6 Enter the following: Homer,Jay,Simpson 70 the pond road,North York,ONT,M3J3M6 Name and address > Homer,Jay,Simpson 70 the pond road,North York,ONT,M3J3M6 Empty Contact: >< Enter the following: Homer,Jay,Simpson 70 the pond road,North York,ON,M3J 3M6 Name and address > Homer,Jay,Simpson 70 the pond road,North York,ON,M3J 3M6 Empty Contact: >< Enter the following: Homer,Jay,Simpson 70 the pond road,,ON,M3J3M6 Name and address > Homer,Jay,Simpson 70 the pond road,,ON,M3J3M6 Empty Contact: >< Enter the following: Homer,Jay,Simpson ,North York,ON,M3J3M6 Name and address > Homer,Jay,Simpson ,North York,ON,M3J3M6 Empty Contact: >< Name and addresses in file: Homer Jay Simpson 70 the pond road North York ON M3J 3M6 Fred Soley 1 York Gate Blvd North York ON M3N 3A1 John Al Doe 1750 Finch Ave E North York ON M2J 2X5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
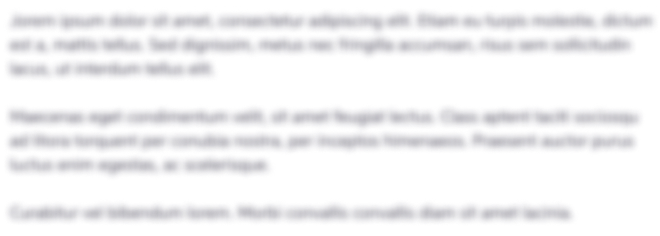
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started