Question
SSH to courses.brockport.edu and create a folder named myipc under the directory of ~/CSC414. Message Queue is a queue of messages used for InterProcess communication,
SSH to courses.brockport.edu and create a folder named myipc under the directory of ~/CSC414.
Message Queue is a queue of messages used for InterProcess communication, which is stored within the kernel and identified by a message queue identifier.
The following code is an implementation of message queue. Two source files, msgQ_write.c and msgQ_read.c, need to be created.
//msgQ_write.c
// C Program for Message Queue (Writer Process)
#include
#include
#include
#define MAX 10
// structure for message queue
struct mesg_buffer {
long mesg_type;
char mesg_text[100];
} message;
int main()
{
key_t key;
int msgid;
// ftok to generate unique key
key = ftok("progfile", 65);
// msgget creates a message queue
// and returns identifier
msgid = msgget(key, 0666 | IPC_CREAT);
message.mesg_type = 1;
printf("Write Data : ");
fgets(message.mesg_text,MAX,stdin);
// msgsnd to send message
msgsnd(msgid, &message, sizeof(message), 0);
// display the message
printf("Data send is : %s ", message.mesg_text);
return 0;
}
//msgQ_read.c
// C Program for Message Queue (Reader Process)
#include
#include
#include
// structure for message queue
struct mesg_buffer {
long mesg_type;
char mesg_text[100];
} message;
int main()
{
key_t key;
int msgid;
// ftok to generate unique key
key = ftok("progfile", 65);
// msgget creates a message queue
// and returns identifier
msgid = msgget(key, 0666 | IPC_CREAT);
// msgrcv to receive message
msgrcv(msgid, &message, sizeof(message), 1, 0);
// display the message
printf("Data Received is : %s ",
message.mesg_text);
// to destroy the message queue
msgctl(msgid, IPC_RMID, NULL);
return 0;
}
System calls used for message queues:
ftok(): is use to generate a unique key. msgget(): either returns the message queue identifier for a newly created message queue or returns the identifiers for a queue which exists with the same key value. msgsnd(): Data is placed on to a message queue by calling msgsnd(). msgrcv(): messages are retrieved from a queue. msgctl(): It performs various operations on a queue. Generally it is use to destroy message queue. |
1a) Please compile them using gcc sourcecode -o output. Run them respectively. Is it successful?
1b) Run msgQ_write.out three times with different inputs. And run msgQ_read.out twice. What are the results? Please paste the screenshots and explain why?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
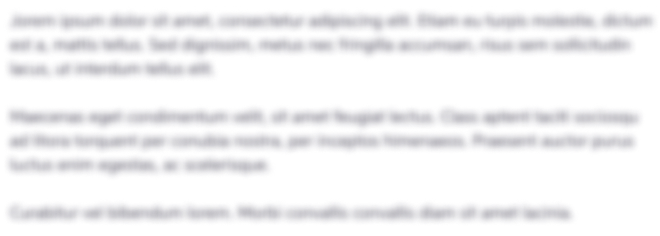
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started