Question
************ Stack ADT Implementation ************ ************ CODE THAT IS TO BE USED AS REFERENCE/USED. ************ public class ArrayStack implements BoundedStackInterface { private int top; private
************ Stack ADT Implementation ************
************ CODE THAT IS TO BE USED AS REFERENCE/USED. ************
public class ArrayStack
private int top; private T[] stack; private static final int DEFCAP = 20; public ArrayStack() { this(DEFCAP); } public ArrayStack(int capacity) { stack = (T[]) new Object[capacity]; top = -1; } @Override public T pop() throws StackUnderflowException { // TODO Auto-generated method stub T element; if (isEmpty()) throw new StackUnderflowException("Pop attempted on an empty stack"); else { element = stack[top]; stack[top] = null; top--; return element; } }
@Override public T peek() throws StackUnderflowException { T element; if (isEmpty()) throw new StackUnderflowException("Peek attempted on an empty stack"); else { element = stack[top]; return element; } }
@Override public boolean isEmpty() { // TODO Auto-generated method stub return (top == -1); }
@Override public int size() { // TODO Auto-generated method stub return 0; }
@Override public void push(T element) throws StackOverflowException { // TODO Auto-generated method stub if (isFull()) throw new StackOverflowException("Push attempted on a full stack"); else { top++; stack[top] = element; } }
@Override public boolean isFull() { // TODO Auto-generated method stub return (top == stack.length-1); } }
-------------------------------------------------------------------------------------------------------
public interface BoundedStackInterface
public boolean isFull(); }
-------------------------------------------------------------------------------------------------------
import java.util.Stack;
public class JavaStack {
public static void main(String[] args) {
Stack
-------------------------------------------------------------------------------------------------------
public interface StackInterface
-------------------------------------------------------------------------------------------------------
public class StackOverflowException extends RuntimeException { public StackOverflowException() { super(); } public StackOverflowException(String message) { super(message); } }
-------------------------------------------------------------------------------------------------------
public class StackUnderflowException extends RuntimeException { public StackUnderflowException() { super(); } public StackUnderflowException(String message) { super(message); } }
-------------------------------------------------------------------------------------------------------
public class StackTester {
public static void main(String[] args) {
ArrayStack
System.out.println("Pop: " + letterStack.pop()); System.out.println("Pop: " + letterStack.pop()); System.out.println("Pop: " + letterStack.pop()); System.out.println("Pop: " + letterStack.pop()); System.out.println("Pop: " + letterStack.pop()); System.out.println("Pop: " + letterStack.pop());
System.out.println("The element at the top of the stack is: " + letterStack.peek()); } }
1. Utilizing your Stack ADT implementations: peek() should return the element at the top or throw the StackUnderflowException this method should not change the state of the stack at all ** The Stacklnterface and ArrayStack files are updated to reflect the throwing of the exception size() should return the number of elements in the stack - see below for the method capacity(), they are not the same . Implement additional methods specified for the bounded stack (ArrayStack) Add an inspector method inspect(int loc). The method will return the element found at the location loc. Return null if the location is invalid (outside of the range of your ArrayStack) . Add a method capacity() that returns the total capacity of the stack (how many elements can be stored in total) . Implement popSome(int count). The method will remove the top count items from the stack. The method should throw a StackUnderflowException as needed. This method should return an ArraylList with the elements "popped . ** The three additional methods will not be part of the Stacklnterface or the BoundedStacklnterface the interfaces already defined are not to be changed, just add the new methods to the ArrayStack. 2. Update the StackTester to test each of the methods. a. Tester does not have to be exhaustive, but make sure to test the different possible scenariOSStep by Step Solution
There are 3 Steps involved in it
Step: 1
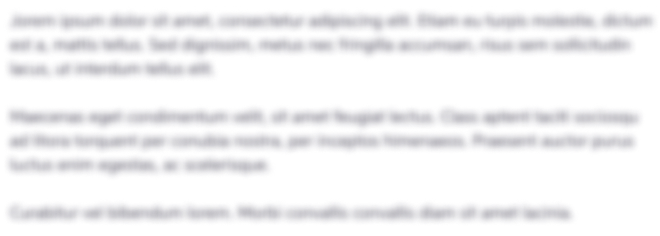
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started