Question
Stacks and Queues Lab Write a java program to simulate job scheduling in an operating system. In this simulation, we will have jobs which need
Stacks and Queues Lab
Write a java program to simulate job scheduling in an operating system.
In this simulation, we will have jobs which need to be run on a processor to complete. Each job will take a varying number of clock cycles to execute. When the required number of clock cycles has passed, the job is removed from the processor and is considered to be completed.
At the start of each clock cycle, the processor checks if it is currently running a job. If it is, it spends another cycle working on that job. If the processor is not running a job, it gets a job from the input queue and begins running it. The input queue contains all jobs which need to be executed.
Jobs have priority as well. A jobs priority is represented by an integer value between 1 and 4, where 1 is the highest priority and 4 is the lowest priority. At the start of each clock cycle, the processor checks the first job in the input queue. If that job has a lower priority that the job currently being run by the processor (or the processor is not currently running a job), then the system behaves as described in the previous paragraph. But if the first job in the input queue has a higher priority than job the processor is currently running, then the job from the queue is run by the processor. The job which was being run by the processor is pushed onto the active job stack. Jobs placed on the active job stack keep all the work that had been completed while being run by the processor. (So if a job needs to run for 5 clock cycles to complete, and had run for 2 clock cycles before being placed on the active job stack, it would only need to be run for 3 more clock cycles before being completed)
At the end of each clock cycle, we determine if the current job has been run for the necessary number of clock cycles. If it has, the job is completed and we remove it from the processor. Once removed, we pop the top element off the active job stack and place it back on the processor. Once placed the clock cycle ends and the process starts over from the start.
The simulation is considered complete when the input queue is empty, the active job stack is empty, and there is no job being run by the processor.
input
To create this simulation in Java, you will create a class named OperatingSystem.java which contains a method named:
public static ArrayList
The JobScheduler method takes a single parameter, an ArrayList of type Job. The Job object is provided. A Job has five instance variables:
jobNumber requiredExecutionTime givenExecutionTime entryTime priority
along with getters and setters for these instance variables. JobScheduler should take this ArrayList of Jobs and place them into Queue of type job which represents our input queue. It should then implement the described simulation. JobScheduler should also implement an ArrayList of Strings for storing the results of the simulation. When a job is completed, the following String should be added to the results ArrayList:
"At CPU time " + cpuTime + " Job Number " + finishedJob.getJobNumber() + " finished processing"
where cpuTime is the current cycle of the CPU and finishedJob is the job which has just completed. When your simulation is completed, JobScheduler should return the results ArrayList.
Also included with this lab is implementations of both Stack and Queue. You should use these implementations for the input queue and the active job stack.
When you have completed your implementation of OperatingSystem.java, use the provided test cases to test your work. When complete, submit your OperatingSystem.java to Blackbaord. If you create a main method for your own testing purposes, you dont need to include it in your submission.
As an example, if your simulator was given an ArrayList containing two jobs, the first job requiring six clock cycles to run and having a priority of 3 and the second job requiring seven clock cycles to run and having a priority of 2, the expected output of your simulator would be:
At CPU time 8 Job Number 2 finished processing At CPU time 13 Job Number 1 finished processing
Here is a suggested algorithm to get you started on your program (note that this is merely a starting point; it isnt necessarily complete!)
initial clock, queue, stack, etc. place all jobs into the inputQueue while all jobs are not completed
if inputQueue is not empty get first item from inputQueue and check if we can place it on the processor
if we need to place the current job on the processor onto the active job stack, do so
endif increment the execution time of the current job by one if the current job has been given all the clock cycles needed to finish
remove job from processor and simulation
place job from active job stack onto processor if applicable endif
increment clock endwhile
____________________________________
public class Stack{ private E[] stack; private int size; @SuppressWarnings("unchecked") public Stack() { stack = (E[]) new Object[500]; size = -1; } public int size() { return size + 1; } public boolean isEmpty() { if(size == -1) { return true; } else { return false; } } public E peek() { if(isEmpty() == true) { return null; } else { return stack[size]; } } public E pop() { if(isEmpty() == true) { return null; } else { E toReturn = stack[size]; stack[size] = null; size--; return toReturn; } } public void push(E anElement) { if(size() == stack.length) { throw new IllegalStateException(); } else { size++; stack[size] = anElement; } } }
___________________________________________________
import static org.junit.Assert.*; import java.util.ArrayList; import org.junit.Test; public class OperatingSystemTest { @Test public void noJobs() { ArrayListtestOne = new ArrayList (); ArrayList results = OperatingSystem.JobScheduler(testOne); assertEquals("When checking the number of elements returned, we",0,results.size()); } @Test public void oneJob() { Job aJob = new Job(1,5,1); ArrayList testOne = new ArrayList (); testOne.add(aJob); ArrayList results = OperatingSystem.JobScheduler(testOne); assertEquals("When checking the number of elements returned, we",1,results.size()); assertEquals("At CPU time 5 Job Number 1 finished processing",results.get(0)); } @Test public void twoJobsNoActiveJobStack() { Job aJob = new Job(1,7,1); Job jobTwo = new Job(2,3,4); ArrayList testTwo = new ArrayList (); testTwo.add(aJob);testTwo.add(jobTwo); ArrayList results = OperatingSystem.JobScheduler(testTwo); assertEquals("When checking the number of elements returned, we",2,results.size()); assertEquals("At CPU time 7 Job Number 1 finished processing",results.get(0)); assertEquals("At CPU time 10 Job Number 2 finished processing",results.get(1)); } @Test public void twoJobsWithActiveJobStack() { Job aJob = new Job(1,6,3); Job jobTwo = new Job(2,7,2); ArrayList testThree = new ArrayList (); testThree.add(aJob);testThree.add(jobTwo); ArrayList results = OperatingSystem.JobScheduler(testThree); assertEquals("When checking the number of elements returned, we",2,results.size()); assertEquals("At CPU time 8 Job Number 2 finished processing",results.get(0)); assertEquals("At CPU time 13 Job Number 1 finished processing",results.get(1)); } @Test public void fourJobsNoActiveJobStack() { Job aJob = new Job(1,6,1); Job jobTwo = new Job(2,7,2); Job jobThree = new Job(3,2,3); Job jobFour = new Job(4,11,4); ArrayList testFour = new ArrayList (); testFour.add(aJob);testFour.add(jobTwo);testFour.add(jobThree);testFour.add(jobFour); ArrayList results = OperatingSystem.JobScheduler(testFour); assertEquals("When checking the number of elements returned, we",4,results.size()); assertEquals("At CPU time 6 Job Number 1 finished processing",results.get(0)); assertEquals("At CPU time 13 Job Number 2 finished processing",results.get(1)); assertEquals("At CPU time 15 Job Number 3 finished processing",results.get(2)); assertEquals("At CPU time 26 Job Number 4 finished processing",results.get(3)); } @Test public void fourJobsWithActiveJobStack() { Job aJob = new Job(1,6,6); Job jobTwo = new Job(2,7,2); Job jobThree = new Job(3,2,1); Job jobFour = new Job(4,11,4); ArrayList testFive = new ArrayList (); testFive.add(aJob);testFive.add(jobTwo);testFive.add(jobThree);testFive.add(jobFour); ArrayList results = OperatingSystem.JobScheduler(testFive); assertEquals("When checking the number of elements returned, we",4,results.size()); assertEquals("At CPU time 4 Job Number 3 finished processing",results.get(0)); assertEquals("At CPU time 10 Job Number 2 finished processing",results.get(1)); assertEquals("At CPU time 21 Job Number 4 finished processing",results.get(2)); assertEquals("At CPU time 26 Job Number 1 finished processing",results.get(3)); } }
_______________________________________________________
//First In First Out FIFO public class Queue{ private SinglyLinkedList linkedList; public Queue() { linkedList = new SinglyLinkedList (); } public int size() { return linkedList.getSize(); } public boolean isEmpty() { return linkedList.isEmpty(); } public E first() { if(isEmpty() == true) { return null; } else { return linkedList.getFirst(); } } public E dequeue() { if(isEmpty() == true) { return null; } else { return linkedList.removeFirst(); } } public void enqueue(E anElement) { linkedList.addLast(anElement); } @SuppressWarnings("hiding") public class SinglyLinkedList { //Instance Variables private Node head; private Node tail; private int size; //Default Constructor public SinglyLinkedList() { size = 0; } //Getter methods public int getSize() { return size; } public boolean isEmpty() { boolean toReturn = false; if(size == 0) { toReturn = true; } return toReturn; } public E getFirst() { if(isEmpty() == true) { return null; } else { return head.getElement(); } } public E getLast() { if(isEmpty() == true) { return null; } else { return tail.getElement(); } } //Setter methods public void addLast(E anElement) { //Create a new node with the given element. Because this is the last node in the linked list, we set the value of the new nodes next node //to null, as there is no next node Node newNode = new Node (anElement,null); //If the linked list is empty we make the new node the head node because it will be both the head and the tail node. if(isEmpty() == true) { head = newNode; } else { //If the linked list has nodes in it already, we link the new node to the current tail of our linked list tail.setNextNode(newNode); } //We update the tail and size instance variables tail = newNode; size++; } public E removeFirst() { if(isEmpty() == true) { return null; } else { E toReturn = head.getElement(); head = head.getNextNode(); size--; if(size == 0) { tail = head; } return toReturn; } } } public class Node { //Instance Variables private G element; private Node next; //Parameterized Constructor public Node(G anElement, Node nextNode) { element = anElement; next = nextNode; } //Getters and setters public G getElement() { return element; } public void setElement(G anElement) { element = anElement; } public Node getNextNode(){ return next; } public void setNextNode(Node nextNode) { next = nextNode; } public String toString() { return element.toString(); } } }
_________________________________________________
public class Job { private int jobNumber; private int requiredExecutionTime; private int givenExecutionTime; private int entryTime; private int priority; public Job(int aJobNumber, int aRequiredExecutionTime, int aPriority) { jobNumber = aJobNumber; requiredExecutionTime = aRequiredExecutionTime; priority = aPriority; givenExecutionTime = 0; entryTime = 0; } public int getJobNumber() { return jobNumber; } public void setJobNumber(int jobNumber) { this.jobNumber = jobNumber; } public int getEntryTime() { return entryTime; } public void setEntryTime(int entryTime) { this.entryTime = entryTime; } public int getPriority() { return priority; } public void setPriority(int priority) { this.priority = priority; } public int getRequiredExecutionTime() { return requiredExecutionTime; } public void setRequiredExecutionTime(int requiredExecutionTime) { this.requiredExecutionTime = requiredExecutionTime; } public int getGivenExecutionTime() { return givenExecutionTime; } public void setGivenExecutionTime(int givenExecutionTime) { this.givenExecutionTime = givenExecutionTime; } public String toString() { return jobNumber +""; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
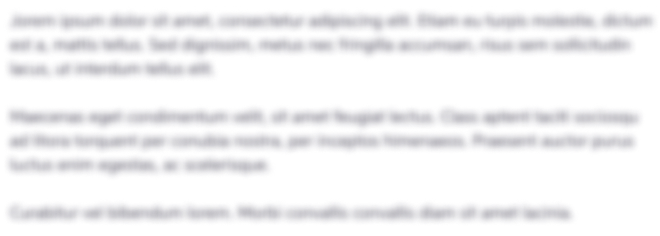
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started