Question
Start with a pillbox having 10 empty slots. Your job is to put 10 pills (lettered A, B, C... J) by selecting a slot randomly
Start with a pillbox having 10 empty slots. Your job is to put 10 pills (lettered A, B, C... J) by selecting a slot randomly for each pill until finding an empty slot. For each pill, record the number of attempts it took to find an empty slot for that pill.
Step 1: Include the iostream, iomanip, memory, and ctime libraries.
Step 2: Create a structure named Pill which will hold two variables:
- the letter of the pill occupying a slot in the pillbox (A - J)
- the number of attempts it took to find an available slot for this pill
The structure also should have a constructor which sets the initial values for each of the variables above.
Step 3: In main, Set the seed of the C++ random number generator to create a different set of numbers each time your program is run.
srand(time(NULL)) ;
Step 4: Create an array of 10 unique pointers to Pill structures
unique_ptr slots[10] ;
By using unique pointers, we guarantee that each Pill can be referenced by one and only one slot in the pillbox.
Step 5: Create a loop for which processes a single pill (lettered A, B, C... through J).
Step 6: For that pill, generate another loop that creates a random number between 0 and 9. Make sure that you track the number of attempts it takes to find an open slot in the pillbox.
Note: To test if a slot is available, say slot[index], use:
if (!slot[index]) ...
Step 6a: If the slot at that index is not available, go back and try another random number, increasing the number of attempts by 1.
Step 6b: If the slot is available, then create a new Pill structure for this pill letter and the number of attempts it took to find an available slot for this pill.
unique_ptr new_pill(new Pill(pill_letter, attempts)) ; slots[index] = move(new_pill) ;
Notice the use of the move function, instead of using an assignment statement,
Exit the loop generating random numbers, and start looking for a slot for the next pill..
Step 7: When all 10 pills have been placed into a slot in the pillbox, print a report showing:
- The slot number (0 - 9)
- The pill letter assigned to that slot (slots[i]->pill_number)
- The number of attempts it took to find an available slot for that pill letter (slots[i]->attempts)
- A grand total of the number of attempts
Notice that pill A (the first pill) should take only one attempt, since all ten slots are available when placing that pill. For pill B, the number of attempts should also be a very low number, since 9 of the 10 slots are still available.
However, as the pill numbers climb, so should the number of attempts. For example when trying to place pill J, 9 of the 10 slots are already filled.
Your report might look like the following (the numbers will be different).
Slot Pill Attempts ---- ---- -------- 0 G 4 1 A 1 2 H 4 3 I 9 4 B 1 5 E 3 6 F 1 7 J 7 8 C 2 9 D 1 Total Attempts 31
Run your program at least twice. Submit your program along with Printscreens show the results of both runs.
Sort the array of pointers used in the above solution by pill letter. (Do not create any additional pointers.)
You may have to add a new variable to the Pill structure for the original slot the pill was in, since the index into the slots array will no longer correspond to the slot in the pillbox in which the pill was initially placed.
Print the report again showing the results by pill letter.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
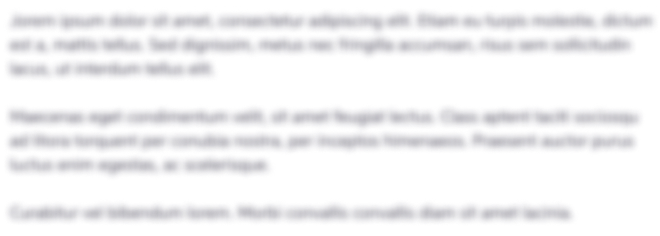
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started