Question
Start with an Order class with two instance variables, item a String and price a double; include two constructors, a no-parameter constructor that passes default
- Start with an Order class with two instance variables, item a String and price a double; include two constructors, a no-parameter constructor that passes default values empty String and zero (0) to the second constructor which then calls the set methods for item and price; the set method for price validates that price is greater than zero (0); include get methods for both instance variables; there is no need for a toString() method
- Instantiate ten objects from the Order class in the start() method of the JavaFX application from which two ObservableList objects should be instantiated: (a) an ObservableList of Strings populated from the get method for the item field; and (b) an ObservableList of Doubles (use the wrapper class as the sub type to instantiate) populated from the get method for the price field
- The JavaFX form will consist of (a) a ListView of Strings from the ObservableList of items; (b) a Slider that represents tip percentage as an int between 15% and 25% (show the current value of the tip percent slider in a matching Label); (c) a Button that is clicked to calculate the total bill; and (d) a result Label
- Include an event handler method for the Button that calculates the total bill as follows: the price field from the ObservableList that matches the selected item from the ListView (the user may purchase only one item at a time) times sales tax (8.625%) plus the tip which is the matching price from the ObservableList times the percentage from the Slider; display with text labels in the result Label the selected item, its matching price, the sales tax, the amount of the tip and the total bill
literally, all i need help with is the last part with the event handler, here is my code:
package tipcalculator;
/** * * @author */ public class Order { String item; double price; public Order() { this("",0.0); } public Order(String item, Double price) { setItem(item); setPrice(price); } /** * updates value of item * @param item */ public void setItem(String item) { this.item = item; } /** * updates value of price * @param price */ public void setPrice(double price) { if(price > 0) { this.price = price; } } public String getItem() { return item; } public double getPrice() { return price; } }
package tipcalculator;
import java.text.DecimalFormat; import javafx.application.Application; import javafx.scene.input.MouseEvent; import javafx.collections.ObservableList; import javafx.collections.FXCollections; import javafx.geometry.HPos; import javafx.geometry.Insets; import javafx.geometry.Pos; import javafx.scene.Scene; import javafx.scene.control.Button; import javafx.scene.control.Label; import javafx.scene.control.ListView; import javafx.scene.layout.VBox; import javafx.scene.layout.GridPane; import javafx.stage.Stage; import javafx.scene.control.Slider; import javafx.scene.layout.FlowPane;
/** * * @author */ public class TipCalculator extends Application { private DecimalFormat twoDecimals; private Label lblHeader; private Label lblResults; private Label lblTip; private Label lblTipHeader; private Slider sliderTip; private ObservableList
vBoxResults = new VBox(35, lblResults, btnCalculate); vBoxResults.setPadding( new Insets(15) ); vBoxResults.setPrefWidth(175); vBoxResults.setStyle("-fx-background-color: cornsilk; -fx-border-color: black; -fx-border-radius: 10;"); GridPane grid = new GridPane();
grid.addRow(0, lblHeader); GridPane.setColumnSpan(lblHeader, 2); GridPane.setHalignment(lblHeader, HPos.CENTER); grid.addRow(1, vBoxListView, vBoxResults,vBoxSlider); Scene scene = new Scene(grid, 1000, 200); primaryStage.setTitle("Book Viewer"); primaryStage.setResizable(false); primaryStage.setScene(scene); primaryStage.show(); }
//event handler to update values for slider private void updateTipSlider(MouseEvent e) { lblTip.setText( twoDecimals.format( sliderTip.getValue() ) ); } private void updateSelectedItem(MouseEvent e) { int index = listView.getSelectionModel().getSelectedIndex(); lblResults.setText( prices.get(index).toString() ); } /** * @param args the command line arguments */ public static void main(String[] args) { launch(args); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
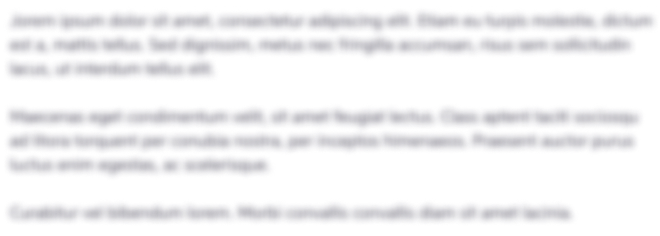
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started