Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Starter code // STEP 2: IDENTIFICATION COMMENTS GO HERE // STEP 2: THE IMPORT STATEMENTS GO HERE public class FLast_Lab05 // STEP 2: CHANGE THIS
Starter code
// STEP 2: IDENTIFICATION COMMENTS GO HERE // STEP 2: THE IMPORT STATEMENTS GO HERE public class FLast_Lab05 // STEP 2: CHANGE THIS TO MATCH YOUR FILE NAME { public static void main(String[] args) // STEP 2: MODIFY HEADER FOR EXCEPTIONS { /* STEP 3: DECLARE THREE PRIMITIVE VARIABLES DECLARE TWO CONSTANTS (OPTIONAL) */ /* STEP 4: DECLARE FILE OBJECT, CHECK FOR EXISTENCE DECLARE SCANNER OBJECT DECLARE TWO PRINTWRITER OBJECTS */ /* STEP 5: LOOP AS LONG AS THERE IS MORE DATA TO READ READ THREE VALUES FROM THE FILE WRITE TO FIRST OUTPUT FILE IF HIGH IS HOT WRITE TO SECOND OUTPUT FILE IF LOW IS COLD */ /* STEP 6: CLOSE ALL THREE FILES */ } }
Input file
1 78 52 2 79 55 3 86 62 4 87 62 5 85 61 6 89 65 7 69 60 8 72 59 9 67 62 10 70 63 11 74 58 12 58 48 13 52 38 14 58 35 15 59 42 16 48 34 17 42 27 18 46 24 19 53 37 20 44 28 21 41 27 22 46 37 23 59 46 24 67 54 25 64 37 26 45 31 27 54 31 28 48 39 29 44 38 30 46 30 31 57 28CIS 255 - Java Programming Laboratory Assignment 5: File Input and Output In this laboratory assignment you will work with Java file input and output syntax to generate lists of hot days and cold nights in a given month. Background A local weather office stores information about the high and low temperatures each day for a month in the file MonthTemps.txt, where each line has information about the temperatures for one day: day high low An example line would be this (on the 28th, the high was 59, and the low was 28): 28 59 28 The weather office needs a new file named HotDays.txt that contains a line with all three values for each day in which the high temperature was at least 80 degrees; it also needs a new file named ColdNights.txt that contains a line with all three values for each day in which the low temperature was below 30 degrees. The number of lines in the original file varies, so you will not know ahead of time whether the number of days is 28, 29, 30, 31, or a smaller number for a partial month. Step 1: Download the Files and Open the Starter File in a Text Editor On Blackboard, right-click the link for the starter file to begin to download it. Make sure you change the file name to include your first initial and last name where indicated (FLast_Lab05.java) and that you specify the file location. Additionally, download the sample MonthTemps.txt file to the same location where you saved the starter file. Once you have downloaded both files, open the starter file by launching your text editor and using the Open command or by right-clicking the file in the folder where you saved it and selecting Open With > (name of your text editor) You need to save the input file to the same folder where you save your source code. Although writing code that opens an input file in a different location is possible, your instructor will not have the same folder setup as you have on your own computer Step 2: Customize Your Code and Add Import Statements Edit the comments at the top of the code to include your name, the course number, the laboratory assignment number, and a brief description of the contents / purpose of the program. You may also wish to include the date (perhaps after the laboratory assignment number). Also, modify the class name to match your file name. As you continue your code, you may go ahead and add comments to the statements or groups of statements that you believe deserve additional explanation, or you may wait until after you have written your entire program to add comments In dealing with file input and output, you will need to add the wildcard import statement for all of the classes in the java.io package and the import statement for the Scanner class used for the input file. The program should display an error message if the input file does not exist if you want the program to display this error message in a dialog box, udd the import statement for optionPane as well. Also, modify the header of the main method to indicate that exceptions of type IOException should be thrown so that you will not have to write more complicated exception-handling syntax. A program that involves file input and output will not compile without some indication as to how A the exceptions are to be handled. This is because the exceptions in the TOException family are checked exceptions; the other exceptions mentioned this semester have been unchecked exceptions. Step 3: Declare Variables for Primitive Values Declare variables for the following quantities (note that your program does not need to initialize these variables, as the program will assign values to them later): Description Day High temperature Low temperature Data Form Whole number Whole number Whole number Since the program writes the values to the appropriate output file when necessary, it does not need to maintain these values throughout the program, so it can reuse the variables to go from one record to the next; in other words, there is no need for 31 day variables, 31 high temperature variables, and 31 low temperature variables. You may also want to declare constants for the criteria for a hot day and a cold night, respectively. Step 4: Declare Objects to Open the Files For the input file, declare an object of type File that uses the file name Month Temps.txt (in quotation marks) as the argument to the new File object. Make sure that the program exits with an error message if it is unable to open the file. After the program confirms that the file does exist, pass the File object to a new Scanner object. A Do not declare the Scanner object until after you check for the existence of the File! An attempt to create a Scanner for a File that does not exist generates an exception message: exiting the program before it tries to create the Scanner prevents the exception message from appearing For the output files, declare two objects of type Printwriter: one using the file name HotDays.txt as the argument to its object, and the other using the file name ColdNights.txt instead. Assume that your program should overwrite the contents of each file if it already exists (do not append to the file). A You must have a separate Printwriter object for each output file, you cannot use the same object for both files. The object variable names must be different. If you want to add to contents of an existing file instead of overwriting it in a different program, not in this one), create a Filewriter object for that file first; then, place the Filewriter variable name in the parentheses of the statement that declares the Printwriter object. CIS 255 (Java Programming). Laboratory Assignments Instructions Page 2 of 4 Step 5: Use a Loop to Read the Contents of the Input Fllo Since you do not know how many days of data will be in the input file, your program needs a loop that continues reading data as long as there are more unread contents to be processed. You can accomplish this by invoking the method hasNext on your Scanner object as the Boolean expression for your loop. The calling object for hasNext() should be the Scanner, not the File. Inside the loop body, use three calls to nextInt() to read the next three values from the file into the variables for the day, high temperature, and low temperature. Then, use an if statement to determine if the data represents a hot day; if so, call the println method to send all three values for that day to the first output file. Write another if statement to determine if the data represents a cold night, with another println method call that sends all three values to the second output file. If the data represents neither a hot day nor a cold night, it should not be written to either output file. To send data to an output file instead of the console, use the name of the Printwriter object for that file instead of system.out as the calling object for the printin method call. A statement that begins with System.out will not send any output to a file. Although highly unlikely, a day might have both a high temperature of at least 80 and a low temperature below 30. Use separate if statements instead of else if to make this work. You must include spaces (in quotation marks) between the values to be written on a line of output to a file; otherwise, the values will run together, Step 6: Close the Files Invoke the close() method on all three file-related objects (the Scanner object and the two Printwriter objects) to ensure that the program terminates properly. Af you do not close an output file, the text to be sent to the file may remain in the output buffer before Step 7: Compile Your Code and Execute the Byte Code To determine if there are any syntax errors in your source code, and to generate the byte code once your source code is free of syntax errors, open the Command Prompt (Windows), the Terminal (macOS), or a similar program on your computer, and run the Java compiler using the javac command as shown below: javac Flast_Lab05.java The results of the build process will appear in the window where you executed the command. If this window indicates that there are syntax errors in your code, the line number where the error occurs will appear after the file name. CIS 255 (Java Programming). Laboratory Assignment 5 Instructions Page 3 of 4 Once you run the javac command and receive no error messages, you are ready to test your program. To run your program, use the java command followed by the class name (with no extension); java FastLab05 If the program is able to read from the sample input file and generate the two output files, you will find the new files in the same location as your source code file; no output will appear in the console. Open these files, verifying that the hot days and cold nights were written properly to their respective files. You may want to modify the values in the sample input file to make sure that it handles varying sets of data properly (for example, longer or shorter months, a larger or smaller number of hot days or cold nights, etc.). Step 8: Add Comments and Submit Your Code Make sure that your code has three or more comments in the body of the program near the statements that you believe warrant the additional description (especially those related to files). Follow the instructions on the course website to attach and submit your source code. Make sure you complete the submission process! If you attach your file without submitting the assignment, your instructor will not receive the file, and you will not receive any credit! I CIS 255 (Java Programming) . Laboratory Assignment 5 Instrictions Page 4 of 4 CIS 255 - Java Programming Laboratory Assignment 5: File Input and Output In this laboratory assignment you will work with Java file input and output syntax to generate lists of hot days and cold nights in a given month. Background A local weather office stores information about the high and low temperatures each day for a month in the file MonthTemps.txt, where each line has information about the temperatures for one day: day high low An example line would be this (on the 28th, the high was 59, and the low was 28): 28 59 28 The weather office needs a new file named HotDays.txt that contains a line with all three values for each day in which the high temperature was at least 80 degrees; it also needs a new file named ColdNights.txt that contains a line with all three values for each day in which the low temperature was below 30 degrees. The number of lines in the original file varies, so you will not know ahead of time whether the number of days is 28, 29, 30, 31, or a smaller number for a partial month. Step 1: Download the Files and Open the Starter File in a Text Editor On Blackboard, right-click the link for the starter file to begin to download it. Make sure you change the file name to include your first initial and last name where indicated (FLast_Lab05.java) and that you specify the file location. Additionally, download the sample MonthTemps.txt file to the same location where you saved the starter file. Once you have downloaded both files, open the starter file by launching your text editor and using the Open command or by right-clicking the file in the folder where you saved it and selecting Open With > (name of your text editor) You need to save the input file to the same folder where you save your source code. Although writing code that opens an input file in a different location is possible, your instructor will not have the same folder setup as you have on your own computer Step 2: Customize Your Code and Add Import Statements Edit the comments at the top of the code to include your name, the course number, the laboratory assignment number, and a brief description of the contents / purpose of the program. You may also wish to include the date (perhaps after the laboratory assignment number). Also, modify the class name to match your file name. As you continue your code, you may go ahead and add comments to the statements or groups of statements that you believe deserve additional explanation, or you may wait until after you have written your entire program to add comments In dealing with file input and output, you will need to add the wildcard import statement for all of the classes in the java.io package and the import statement for the Scanner class used for the input file. The program should display an error message if the input file does not exist if you want the program to display this error message in a dialog box, udd the import statement for optionPane as well. Also, modify the header of the main method to indicate that exceptions of type IOException should be thrown so that you will not have to write more complicated exception-handling syntax. A program that involves file input and output will not compile without some indication as to how A the exceptions are to be handled. This is because the exceptions in the TOException family are checked exceptions; the other exceptions mentioned this semester have been unchecked exceptions. Step 3: Declare Variables for Primitive Values Declare variables for the following quantities (note that your program does not need to initialize these variables, as the program will assign values to them later): Description Day High temperature Low temperature Data Form Whole number Whole number Whole number Since the program writes the values to the appropriate output file when necessary, it does not need to maintain these values throughout the program, so it can reuse the variables to go from one record to the next; in other words, there is no need for 31 day variables, 31 high temperature variables, and 31 low temperature variables. You may also want to declare constants for the criteria for a hot day and a cold night, respectively. Step 4: Declare Objects to Open the Files For the input file, declare an object of type File that uses the file name Month Temps.txt (in quotation marks) as the argument to the new File object. Make sure that the program exits with an error message if it is unable to open the file. After the program confirms that the file does exist, pass the File object to a new Scanner object. A Do not declare the Scanner object until after you check for the existence of the File! An attempt to create a Scanner for a File that does not exist generates an exception message: exiting the program before it tries to create the Scanner prevents the exception message from appearing For the output files, declare two objects of type Printwriter: one using the file name HotDays.txt as the argument to its object, and the other using the file name ColdNights.txt instead. Assume that your program should overwrite the contents of each file if it already exists (do not append to the file). A You must have a separate Printwriter object for each output file, you cannot use the same object for both files. The object variable names must be different. If you want to add to contents of an existing file instead of overwriting it in a different program, not in this one), create a Filewriter object for that file first; then, place the Filewriter variable name in the parentheses of the statement that declares the Printwriter object. CIS 255 (Java Programming). Laboratory Assignments Instructions Page 2 of 4 Step 5: Use a Loop to Read the Contents of the Input Fllo Since you do not know how many days of data will be in the input file, your program needs a loop that continues reading data as long as there are more unread contents to be processed. You can accomplish this by invoking the method hasNext on your Scanner object as the Boolean expression for your loop. The calling object for hasNext() should be the Scanner, not the File. Inside the loop body, use three calls to nextInt() to read the next three values from the file into the variables for the day, high temperature, and low temperature. Then, use an if statement to determine if the data represents a hot day; if so, call the println method to send all three values for that day to the first output file. Write another if statement to determine if the data represents a cold night, with another println method call that sends all three values to the second output file. If the data represents neither a hot day nor a cold night, it should not be written to either output file. To send data to an output file instead of the console, use the name of the Printwriter object for that file instead of system.out as the calling object for the printin method call. A statement that begins with System.out will not send any output to a file. Although highly unlikely, a day might have both a high temperature of at least 80 and a low temperature below 30. Use separate if statements instead of else if to make this work. You must include spaces (in quotation marks) between the values to be written on a line of output to a file; otherwise, the values will run together, Step 6: Close the Files Invoke the close() method on all three file-related objects (the Scanner object and the two Printwriter objects) to ensure that the program terminates properly. Af you do not close an output file, the text to be sent to the file may remain in the output buffer before Step 7: Compile Your Code and Execute the Byte Code To determine if there are any syntax errors in your source code, and to generate the byte code once your source code is free of syntax errors, open the Command Prompt (Windows), the Terminal (macOS), or a similar program on your computer, and run the Java compiler using the javac command as shown below: javac Flast_Lab05.java The results of the build process will appear in the window where you executed the command. If this window indicates that there are syntax errors in your code, the line number where the error occurs will appear after the file name. CIS 255 (Java Programming). Laboratory Assignment 5 Instructions Page 3 of 4 Once you run the javac command and receive no error messages, you are ready to test your program. To run your program, use the java command followed by the class name (with no extension); java FastLab05 If the program is able to read from the sample input file and generate the two output files, you will find the new files in the same location as your source code file; no output will appear in the console. Open these files, verifying that the hot days and cold nights were written properly to their respective files. You may want to modify the values in the sample input file to make sure that it handles varying sets of data properly (for example, longer or shorter months, a larger or smaller number of hot days or cold nights, etc.). Step 8: Add Comments and Submit Your Code Make sure that your code has three or more comments in the body of the program near the statements that you believe warrant the additional description (especially those related to files). Follow the instructions on the course website to attach and submit your source code. Make sure you complete the submission process! If you attach your file without submitting the assignment, your instructor will not receive the file, and you will not receive any credit! I CIS 255 (Java Programming) . Laboratory Assignment 5 Instrictions Page 4 of 4
Step by Step Solution
There are 3 Steps involved in it
Step: 1
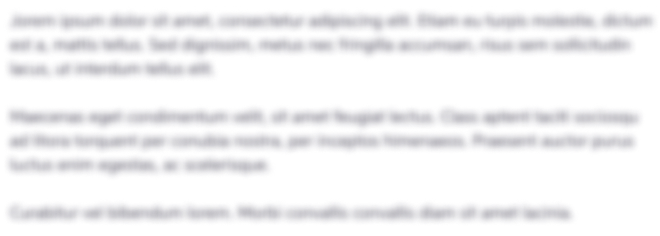
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started