Question
StdAudio /************************************************************************* * Compilation: javac StdAudio.java * Execution: java StdAudio * * Simple library for reading, writing, and manipulating .wav files. * * Limitations *
StdAudio
/************************************************************************* * Compilation: javac StdAudio.java * Execution: java StdAudio * * Simple library for reading, writing, and manipulating .wav files.
* * Limitations * ----------- * - Does not seem to work properly when reading .wav files from a .jar file. * - Assumes the audio is monaural, with sampling rate of 44,100. * *************************************************************************/
import java.applet.*; import java.io.*; import java.net.*;
import javax.sound.sampled.*;
/** * Standard audio. This class provides a basic capability for * creating, reading, and saving audio. *
* The audio format uses a sampling rate of 44,100 (CD quality audio), 16-bit, monaural. * *
* For additional documentation, see Section 1.5 of * Introduction to Programming in Java: An Interdisciplinary Approach by Robert Sedgewick and Kevin Wayne. */ public final class StdAudio {
/** * The sample rate - 44100 Hz for CD quality audio. */ public static final int SAMPLE_RATE = 44100;
private static final int BYTES_PER_SAMPLE = 2; // 16-bit audio private static final int BITS_PER_SAMPLE = 16; // 16-bit audio private static final double MAX_16_BIT = Short.MAX_VALUE; // 32,767 private static final int SAMPLE_BUFFER_SIZE = 4096;
private static SourceDataLine line; // to play the sound private static byte[] buffer; // our internal buffer private static int bufferSize = 0; // number of samples currently in internal buffer
// not-instantiable private StdAudio() { }
// static initializer static { init(); }
// open up an audio stream private static void init() { try { // 44,100 samples per second, 16-bit audio, mono, signed PCM, little Endian AudioFormat format = new AudioFormat((float) SAMPLE_RATE, BITS_PER_SAMPLE, 1, true, false); DataLine.Info info = new DataLine.Info(SourceDataLine.class, format);
line = (SourceDataLine) AudioSystem.getLine(info); line.open(format, SAMPLE_BUFFER_SIZE * BYTES_PER_SAMPLE);
// the internal buffer is a fraction of the actual buffer size, this choice is arbitrary // it gets divided because we can't expect the buffered data to line up exactly with when // the sound card decides to push out its samples. buffer = new byte[SAMPLE_BUFFER_SIZE * BYTES_PER_SAMPLE/3]; } catch (Exception e) { System.out.println(e.getMessage()); System.exit(1); }
// no sound gets made before this call line.start(); }
/** * Close standard audio. */ public static void close() { line.drain(); line.stop(); }
/** * Write one sample (between -1.0 and +1.0) to standard audio. If the sample * is outside the range, it will be clipped. */ public static void play(double in) {
// clip if outside [-1, +1] if (in +1.0) in = +1.0;
// convert to bytes short s = (short) (MAX_16_BIT * in); buffer[bufferSize++] = (byte) s; buffer[bufferSize++] = (byte) (s >> 8); // little Endian
// send to sound card if buffer is full if (bufferSize >= buffer.length) { line.write(buffer, 0, buffer.length); bufferSize = 0; } }
public static void play(double hz, double duration, double amplitude) { StdAudio.play(note(hz,duration,amplitude)); }
/** * Write an array of samples (between -1.0 and +1.0) to standard audio. If a sample * is outside the range, it will be clipped. */ public static void play(double[] input) { for (int i = 0; i
/** * Read audio samples from a file (in .wav or .au format) and return them as a double array * with values between -1.0 and +1.0. */ public static double[] read(String filename) { byte[] data = readByte(filename); int N = data.length; double[] d = new double[N/2]; for (int i = 0; i
/** * Play a sound file (in .wav or .au format) in a background thread. */ public static void play(String filename) { URL url = null; try { File file = new File(filename); if (file.canRead()) url = file.toURI().toURL(); } catch (MalformedURLException e) { e.printStackTrace(); } // URL url = StdAudio.class.getResource(filename); if (url == null) throw new RuntimeException("audio " + filename + " not found"); AudioClip clip = Applet.newAudioClip(url); clip.play(); }
/** * Loop a sound file (in .wav or .au format) in a background thread. */ public static void loop(String filename) { URL url = null; try { File file = new File(filename); if (file.canRead()) url = file.toURI().toURL(); } catch (MalformedURLException e) { e.printStackTrace(); } // URL url = StdAudio.class.getResource(filename); if (url == null) throw new RuntimeException("audio " + filename + " not found"); AudioClip clip = Applet.newAudioClip(url); clip.loop(); }
// return data as a byte array private static byte[] readByte(String filename) { byte[] data = null; AudioInputStream ais = null; try {
// try to read from file File file = new File(filename); if (file.exists()) { ais = AudioSystem.getAudioInputStream(file); data = new byte[ais.available()]; ais.read(data); }
// try to read from URL else { URL url = StdAudio.class.getResource(filename); ais = AudioSystem.getAudioInputStream(url); data = new byte[ais.available()]; ais.read(data); } } catch (Exception e) { System.out.println(e.getMessage()); throw new RuntimeException("Could not read " + filename); }
return data; }
/** * Save the double array as a sound file (using .wav or .au format). */ public static void save(String filename, double[] input) {
// assumes 44,100 samples per second // use 16-bit audio, mono, signed PCM, little Endian AudioFormat format = new AudioFormat(SAMPLE_RATE, 16, 1, true, false); byte[] data = new byte[2 * input.length]; for (int i = 0; i > 8); }
// now save the file try { ByteArrayInputStream bais = new ByteArrayInputStream(data); AudioInputStream ais = new AudioInputStream(bais, format, input.length); if (filename.endsWith(".wav") || filename.endsWith(".WAV")) { AudioSystem.write(ais, AudioFileFormat.Type.WAVE, new File(filename)); } else if (filename.endsWith(".au") || filename.endsWith(".AU")) { AudioSystem.write(ais, AudioFileFormat.Type.AU, new File(filename)); } else { throw new RuntimeException("File format not supported: " + filename); } } catch (Exception e) { System.out.println(e); System.exit(1); } }
/*********************************************************************** * sample test client ***********************************************************************/
// create a note (sine wave) of the given frequency (Hz), for the given // duration (seconds) scaled to the given volume (amplitude) public static double[] note(double hz, double duration, double amplitude) { int N = (int) (StdAudio.SAMPLE_RATE * duration); double[] a = new double[N+1]; for (int i = 0; i
/** * Test client - play an A major scale to standard audio. */ public static void main(String[] args) {
// 440 Hz for 1 sec double freq = 440.0; for (int i = 0; i
// scale increments int[] steps = { 0, 2, 4, 5, 7, 9, 11, 12 }; for (int i = 0; i
// need to call this in non-interactive stuff so the program doesn't terminate // until all the sound leaves the speaker. StdAudio.close();
// need to terminate a Java program with sound System.exit(0); } }
PLEASE USE JAVA
There are 88 keys on a standard piano. Each key produces a music note that is at a different frequency (a different musical pitch). For example the A4 note on a piano is key 49 and has a frequency of 440.0 Hz. You can convert any piano key into its corresponding frequency using the following formula: Where n is the piano note number (between f(n) = 2 "12.0" x 440.0Hz 1 and 88) and f(n) gives the frequency in Hertz. Note that 2 is raised to the power of that fraction. You are given a Helper file for this assignment called StdAudio.java and a starter BlueJ project. You should add your solution to this existing BlueJ project. The helper file allows you to play a musical note (provided you supply a frequency (double), duration (in seconds) (double)) and volume (as a percentage) (double). For example to play an A4 note for 0.7 seconds at half volume you do as follows: StdAudio.play (440.0, 0.7, 0.5); //or similarly: double a 4 = 440.0; StdAudio.play (a4, 0.7, 0.5); For Problem 1 you will create a program that plays a musical note (a piano key between 1 and 88 of your predetermined choosing) and asks the user to guess what the note is. The user will be asked to input a number between 1 and 88 representing their guess as to which piano key matches the sound they heard. Your program will then play their note so they can hear what they have input. You should output the user guessed piano key number and the corresponding frequency and the actual piano key number and its corresponding frequency indicating by how many keys the user was incorrect - or a congratulatory message if they got it correct. See below a screen capture. For this assignment your output should match exactly as you see below including number of decimal places, punctuation and spacing. See also a youtube clip so you may hear the wondrous music. Your program should match exactly the sample program: Blue Tem Windows w2020 I'll play a music note you try to guess the note! Press Enter when ready. Youtube Link: https:// www.youtube.com/ watch?v=hpok9h Ibuo What is your guess? (number between 1 and 88): You guessed 64, which is 1046.5Hz and sounds like this. Sorry! The correct answer is 50, which is 466.2Hz. You were off by 14 notes There are 88 keys on a standard piano. Each key produces a music note that is at a different frequency (a different musical pitch). For example the A4 note on a piano is key 49 and has a frequency of 440.0 Hz. You can convert any piano key into its corresponding frequency using the following formula: Where n is the piano note number (between f(n) = 2 "12.0" x 440.0Hz 1 and 88) and f(n) gives the frequency in Hertz. Note that 2 is raised to the power of that fraction. You are given a Helper file for this assignment called StdAudio.java and a starter BlueJ project. You should add your solution to this existing BlueJ project. The helper file allows you to play a musical note (provided you supply a frequency (double), duration (in seconds) (double)) and volume (as a percentage) (double). For example to play an A4 note for 0.7 seconds at half volume you do as follows: StdAudio.play (440.0, 0.7, 0.5); //or similarly: double a 4 = 440.0; StdAudio.play (a4, 0.7, 0.5); For Problem 1 you will create a program that plays a musical note (a piano key between 1 and 88 of your predetermined choosing) and asks the user to guess what the note is. The user will be asked to input a number between 1 and 88 representing their guess as to which piano key matches the sound they heard. Your program will then play their note so they can hear what they have input. You should output the user guessed piano key number and the corresponding frequency and the actual piano key number and its corresponding frequency indicating by how many keys the user was incorrect - or a congratulatory message if they got it correct. See below a screen capture. For this assignment your output should match exactly as you see below including number of decimal places, punctuation and spacing. See also a youtube clip so you may hear the wondrous music. Your program should match exactly the sample program: Blue Tem Windows w2020 I'll play a music note you try to guess the note! Press Enter when ready. Youtube Link: https:// www.youtube.com/ watch?v=hpok9h Ibuo What is your guess? (number between 1 and 88): You guessed 64, which is 1046.5Hz and sounds like this. Sorry! The correct answer is 50, which is 466.2Hz. You were off by 14 notesStep by Step Solution
There are 3 Steps involved in it
Step: 1
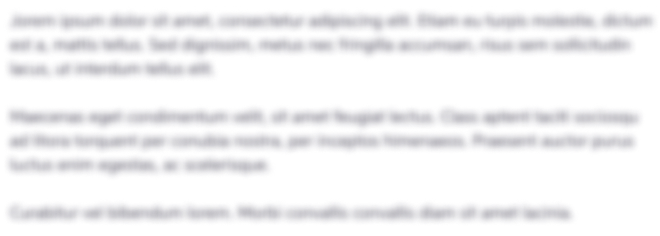
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started